ตอนที่ 6 : How to use php Add Entity to a Table Storage - บันทึกข้อมูลลงตาราง |
ตอนที่ 6 : How to use php Add Entity to a Table Storage - บันทึกข้อมูลลงตาราง บทความที่สองจะเป็นตัวอย่างเกี่ยวกับการ Insert ข้อมูลลงใน Table Storage ของ Windows Azure ซึ่งจะเหมือนกับการ Insert ข้อมูลแบบ SQL ปกติ เพียงแต่เราจะใช้การ Insert ผ่าน SDK ด้วยการเขียนคำสั่งเพียงง่าย ๆ ก็สามารถที่จะ Insert ลงใน Table ได้ทันที
ตาราง Table ที่อยู่บน Windows Azure
Syntax การ Insert ข้อมูล
$entity = new Entity();
$entity->setPartitionKey("myCustomer");
$entity->setRowKey("C001");
$entity->addProperty("CustomerID", EdmType::STRING, "C001");
$entity->addProperty("Name", EdmType::STRING, "Win Weerachai");
$entity->addProperty("Email", EdmType::STRING, "[email protected]");
$entity->addProperty("CountryCode", EdmType::STRING, "TH");
$entity->addProperty("Budget", EdmType::DOUBLE, 1000000.00);
$entity->addProperty("Used", EdmType::DOUBLE, 600000.00);
$tableRestProxy->insertEntity("customer", $entity);
จัดเก็บไว้ใน Table ชื่อว่า customer จะสังเกตุว่ามีการ Insert ข้อมูลที่เป็น PartitionKey และ RowKey ซึ่งตัวนี้เปรียบเหสมือน Key ของ Rows ควรเป็นค่าที่ไม่ซ้ำกัน ใช้ในการอ้างอิงถึง Key และ Index ทำให้สามารถอ่านข้อมูลได้อย่างรวดเร็ว
Example 1 เขียน PHP เพื่บันทึกข้อมูลลงใน Table Storage ของ Windows Azure
phpAzureTable.php
<?php
require_once "WindowsAzure/WindowsAzure.php";
require_once 'PHPUnit\autoload.php';
use WindowsAzure\Common\ServicesBuilder;
use WindowsAzure\Common\ServiceException;
use WindowsAzure\Table\Models\Entity;
use WindowsAzure\Table\Models\EdmType;
// Create Connection String
$connectionString = "DefaultEndpointsProtocol=http;AccountName=[yourAccount];AccountKey=[yourKey]";
// Create table REST proxy.
$tableRestProxy = ServicesBuilder::getInstance()->createTableService($connectionString);
$entity = new Entity();
$entity->setPartitionKey("myCustomer");
$entity->setRowKey("C001");
$entity->addProperty("CustomerID", EdmType::STRING, "C001");
$entity->addProperty("Name", EdmType::STRING, "Win Weerachai");
$entity->addProperty("Email", EdmType::STRING, "[email protected]");
$entity->addProperty("CountryCode", EdmType::STRING, "TH");
$entity->addProperty("Budget", EdmType::DOUBLE, 1000000.00);
$entity->addProperty("Used", EdmType::DOUBLE, 600000.00);
try {
$tableRestProxy->insertEntity("customer", $entity);
echo "Storage Table 'customer' has been inserted.";
}
catch(ServiceException $e){
$code = $e->getCode();
$error_message = $e->getMessage();
// Handle exception based on error codes and messages.
// Error codes and messages can be found here:
// http://msdn.microsoft.com/en-us/library/windowsazure/dd179438.aspx
}
?>
Screenshot
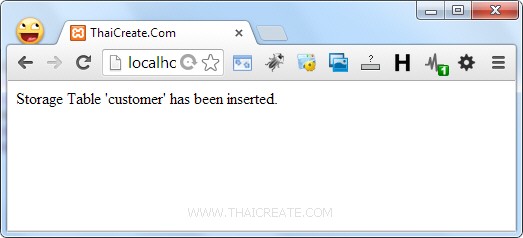
แสดงการ Insert ข้อมูล
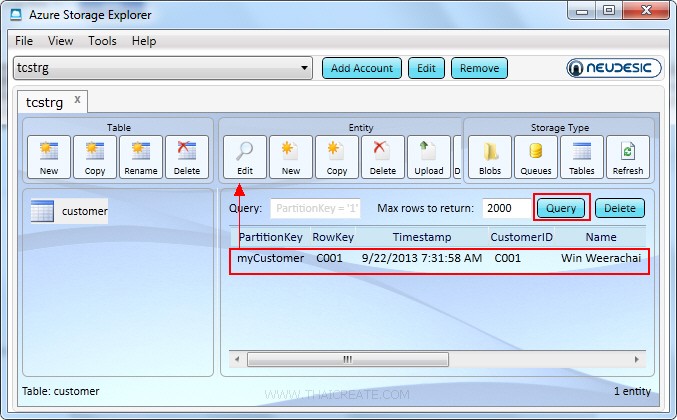
ดูผ่านโปรแกรม Azure Storage Explorer เลือก Edit
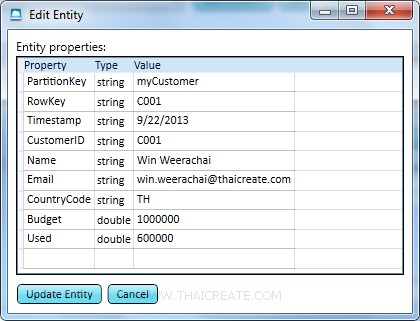
แสดงข้อมูลที่ถูก Insert ลงไปใน Table

Example 2 เขียน PHP สร้าง Form สำหรับ Insert ข้อมูลลงใน Azure Table Storage
phpAzureTable1.php
<html>
<head>
<title>ThaiCreate.Com</title>
</head>
<body>
<form action="phpAzureTable2.php" name="frmAdd" method="post">
<table width="600" border="1">
<tr>
<th width="91"> <div align="center">CustomerID </div></th>
<th width="160"> <div align="center">Name </div></th>
<th width="198"> <div align="center">Email </div></th>
<th width="97"> <div align="center">CountryCode </div></th>
<th width="70"> <div align="center">Budget </div></th>
<th width="70"> <div align="center">Used </div></th>
</tr>
<tr>
<td><div align="center"><input type="text" name="txtCustomerID" size="5"></div></td>
<td><input type="text" name="txtName" size="20"></td>
<td><input type="text" name="txtEmail" size="20"></td>
<td><div align="center"><input type="text" name="txtCountryCode" size="2"></div></td>
<td align="right"><input type="text" name="txtBudget" size="5"></td>
<td align="right"><input type="text" name="txtUsed" size="5"></td>
</tr>
</table>
<input type="submit" name="submit" value="submit">
</form>
</body>
</html>
phpAzureTable2.php
<?php
require_once "WindowsAzure/WindowsAzure.php";
require_once 'PHPUnit\autoload.php';
use WindowsAzure\Common\ServicesBuilder;
use WindowsAzure\Common\ServiceException;
use WindowsAzure\Table\Models\Entity;
use WindowsAzure\Table\Models\EdmType;
// Create Connection String
$connectionString = "DefaultEndpointsProtocol=http;AccountName=[yourAccount];AccountKey=[yourKey]";
// Create table REST proxy.
$tableRestProxy = ServicesBuilder::getInstance()->createTableService($connectionString);
$entity = new Entity();
$entity->setPartitionKey("myCustomer");
$entity->setRowKey($_POST["txtCustomerID"]);
$entity->addProperty("CustomerID", EdmType::STRING, $_POST["txtCustomerID"]);
$entity->addProperty("Name", EdmType::STRING, $_POST["txtName"]);
$entity->addProperty("Email", EdmType::STRING, $_POST["txtEmail"]);
$entity->addProperty("CountryCode", EdmType::STRING, $_POST["txtCountryCode"]);
$entity->addProperty("Budget", EdmType::DOUBLE, (double)$_POST["txtBudget"]);
$entity->addProperty("Used", EdmType::DOUBLE, (double)$_POST["txtUsed"]);
try {
$tableRestProxy->insertEntity("customer", $entity);
echo "Storage Table 'customer' has been inserted.";
}
catch(ServiceException $e){
$code = $e->getCode();
$error_message = $e->getMessage();
// Handle exception based on error codes and messages.
// Error codes and messages can be found here:
// http://msdn.microsoft.com/en-us/library/windowsazure/dd179438.aspx
}
?>
Screenshot
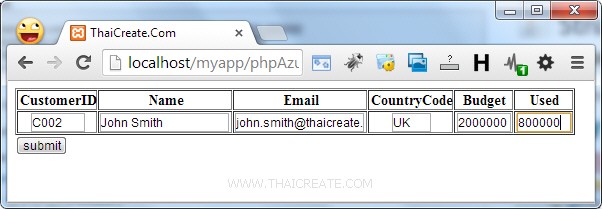
แสดง Form สำหรับ Insert ข้อมูล
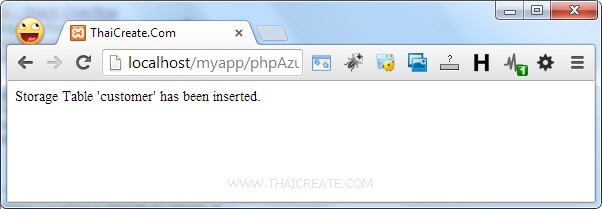
แสดงการ Insert ข้อมูล
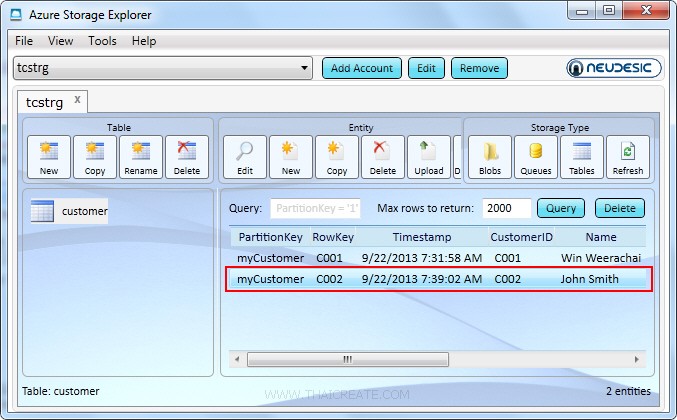
ดูผ่านโปรแกรม Azure Storage Explorer เลือก Edit
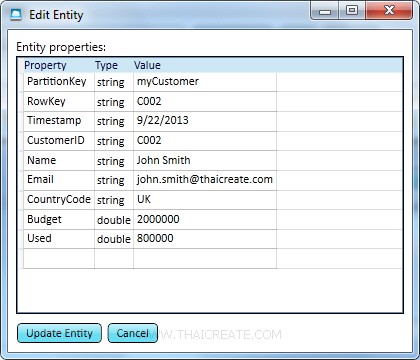
แสดงข้อมูลที่ถูก Insert เข้าไปใน Table Storage
บทความที่เกี่ยวข้อง
บทความถัดไปที่แนะนำให้อ่าน
|