ตอนที่ 8 : How to use php Update Entity in Table Storage - แก้ไขข้อมูลในตาราง |
ตอนที่ 8 : How to use php Update Entity in Table Storage - แก้ไขข้อมูลในตาราง บทความนี้จะเป็นตัวอย่างการเขียน PHP กับ Table Storage บน Windows Azure โดยจะเป็นรูปแบบการแก้ไข Entity หรือแก้ไขข้อมูลใน Table Storage ซึ่งใน Concept การจัดเก็บนั้นเราจะสามารถที่จะแก้ไข Update ได้เหมือนกับ Table แบบ Rows คือเลือกที่จะ Update ในแต่ล่ะฟิวด์ของข้อมูลได้ โดยในตัวอย่างนี้จะแบ่งเป็น Step การแสดงข้อมูล การเลือกข้อมูลที่จะแก้ไขและ Update แสดงบน Form เพื่อให้ User ทำการแก้ไขข้อมูลต่าง ๆ ที่ต้องการ จากนั้นก็เป็นการบันทึกการแก้ไขไปยัง Table Storage บน Windows Azure
ข้อมูล Table Storage ที่อยู่บน Windows Azure
Syntax การ Update ข้อมูล
$result = $tableRestProxy->getEntity("customer", "myCustomer", $_GET["CusID"]);
$entity = $result->getEntity();
$entity->addProperty("Name", EdmType::STRING, $_POST["txtName"]);
$entity->addProperty("Email", EdmType::STRING, $_POST["txtEmail"]);
$entity->addProperty("CountryCode", EdmType::STRING, $_POST["txtCountryCode"]);
$entity->addProperty("Budget", EdmType::DOUBLE, (double)$_POST["txtBudget"]);
$entity->addProperty("Used", EdmType::DOUBLE, (double)$_POST["txtUsed"]);
$tableRestProxy->updateEntity("customer", $entity);
เป็นการ Update ข้อมูลใน Table Storage โดยในขั้นแรกจะต้อง Filter เลือกข้อมูลที่จะ Update ซะก่อน
Example เขียน PHP เพื่อ Update แก้ไขข้อมูล Table Storage บน Windows Azure
phpAzureTable1.php (ไฟล์สำหรับแสดงรายการข้อมูลทั้งหมด และ สามารถเลือกรายการที่จะทำการแก้ไขได้)
<?php
require_once "WindowsAzure/WindowsAzure.php";
require_once 'PHPUnit\autoload.php';
use WindowsAzure\Common\ServicesBuilder;
use WindowsAzure\Common\ServiceException;
// Create Connection String
$connectionString = "DefaultEndpointsProtocol=http;AccountName=[yourAccount];AccountKey=[yourKey]";
// Create table REST proxy.
$tableRestProxy = ServicesBuilder::getInstance()->createTableService($connectionString);
$filter = " PartitionKey eq 'myCustomer' ";
try {
$result = $tableRestProxy->queryEntities("customer", $filter);
}
catch(ServiceException $e){
$code = $e->getCode();
$error_message = $e->getMessage();
}
$entities = $result->getEntities();
echo "
<table width=\"800\" border=\"1\">
<tr>
<th width=\"91\"> <div align=\"center\">PartitionKey </div></th>
<th width=\"91\"> <div align=\"center\">RowKey </div></th>
<th width=\"91\"> <div align=\"center\">CustomerID </div></th>
<th width=\"98\"> <div align=\"center\">Name </div></th>
<th width=\"198\"> <div align=\"center\">Email </div></th>
<th width=\"97\"> <div align=\"center\">CountryCode </div></th>
<th width=\"59\"> <div align=\"center\">Budget </div></th>
<th width=\"71\"> <div align=\"center\">Used </div></th>
<th width=\"71\"> <div align=\"center\">Edit </div></th>
</tr> ";
foreach($entities as $entity){
echo "
<tr>
<td><div align=\"center\">". $entity->getPartitionKey()."</div></td>
<td><div align=\"center\">".$entity->getRowKey()."</div></td>
<td><div align=\"center\">".$entity->getProperty("CustomerID")->getValue()."</div></td>
<td>".$entity->getProperty("Name")->getValue()."</td>
<td>".$entity->getProperty("Email")->getValue()."</td>
<td><div align=\"center\">".$entity->getProperty("CountryCode")->getValue()."</div></td>
<td align=\"right\">".$entity->getProperty("Budget")->getValue()."</td>
<td align=\"right\">".$entity->getProperty("Used")->getValue()."</td>
<td align=\"center\"><a href='phpAzureTable2.php?CusID=".$entity->getProperty("CustomerID")->getValue()."'>Edit</a></td>
</tr>
";
}
echo "</table>";
?>
phpAzureTable2.php (ไฟล์สำหรับเป็น Form ในการรับข้อมูลที่จะแก้ไข)
<?php
require_once "WindowsAzure/WindowsAzure.php";
require_once 'PHPUnit\autoload.php';
use WindowsAzure\Common\ServicesBuilder;
use WindowsAzure\Common\ServiceException;
// Create Connection String
$connectionString = "DefaultEndpointsProtocol=http;AccountName=[yourAccount];AccountKey=[yourKey]";
// Create table REST proxy.
$tableRestProxy = ServicesBuilder::getInstance()->createTableService($connectionString);
try {
$result = $tableRestProxy->getEntity("customer", "myCustomer", $_GET["CusID"]);
}
catch(ServiceException $e){
$code = $e->getCode();
$error_message = $e->getMessage();
}
$entity = $result->getEntity();
?>
<form action="phpAzureTable3.php?CusID=<?php echo $_GET["CusID"];?>" name="frmEdit" method="post">
<table width="329" border="1">
<tr>
<td width="118">PartitionKey</td>
<td width="195"><?php echo $entity->getPartitionKey()?></td>
</tr>
<tr>
<td>RowKey</td>
<td><?php echo $entity->getRowKey();?></td>
</tr>
<tr>
<td>CustomerID</td>
<td><?php echo $entity->getProperty("CustomerID")->getValue();?></td>
</tr>
<tr>
<td>Name</td>
<td><input type="text" name="txtName" size="20" value="<?php echo $entity->getProperty("Name")->getValue();?>"></td>
</tr>
<tr>
<td>Email</td>
<td><input type="text" name="txtEmail" size="20" value="<?php echo $entity->getProperty("Email")->getValue();?>"></td>
</tr>
<tr>
<td>CountryCode</td>
<td><input type="text" name="txtCountryCode" size="2" value="<?php echo $entity->getProperty("CountryCode")->getValue();?>"></td>
</tr>
<tr>
<td>Budget</td>
<td><input type="text" name="txtBudget" size="5" value="<?php echo $entity->getProperty("Budget")->getValue();?>"></td>
</tr>
<tr>
<td>Used</td>
<td><input type="text" name="txtUsed" size="5" value="<?php echo $entity->getProperty("Used")->getValue();?>"></td>
</tr>
</table>
<br>
<input type="submit" name="submit" value="submit">
</form>
phpAzureTable3.php (ไฟล์สำหรับทำการ Update แก้ไขข้อมูล)
<?php
require_once "WindowsAzure/WindowsAzure.php";
require_once 'PHPUnit\autoload.php';
use WindowsAzure\Common\ServicesBuilder;
use WindowsAzure\Common\ServiceException;
use WindowsAzure\Table\Models\Entity;
use WindowsAzure\Table\Models\EdmType;
// Create Connection String
$connectionString = "DefaultEndpointsProtocol=http;AccountName=[yourAccount];AccountKey=[yourKey]";
// Create table REST proxy.
$tableRestProxy = ServicesBuilder::getInstance()->createTableService($connectionString);
$result = $tableRestProxy->getEntity("customer", "myCustomer", $_GET["CusID"]);
$entity = $result->getEntity();
$entity->addProperty("Name", EdmType::STRING, $_POST["txtName"]);
$entity->addProperty("Email", EdmType::STRING, $_POST["txtEmail"]);
$entity->addProperty("CountryCode", EdmType::STRING, $_POST["txtCountryCode"]);
$entity->addProperty("Budget", EdmType::DOUBLE, (double)$_POST["txtBudget"]);
$entity->addProperty("Used", EdmType::DOUBLE, (double)$_POST["txtUsed"]);
try {
// Update Table
$tableRestProxy->updateEntity("customer", $entity);
header("location:phpAzureTable1.php");
exit();
}
catch(ServiceException $e){
$code = $e->getCode();
$error_message = $e->getMessage();
}
?>
Screenshot
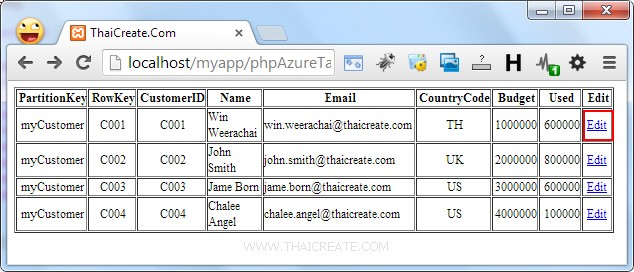
แสดงรายการข้อมูลจาก Table Storage ให้เลือกรายการที่ต้องการ Update
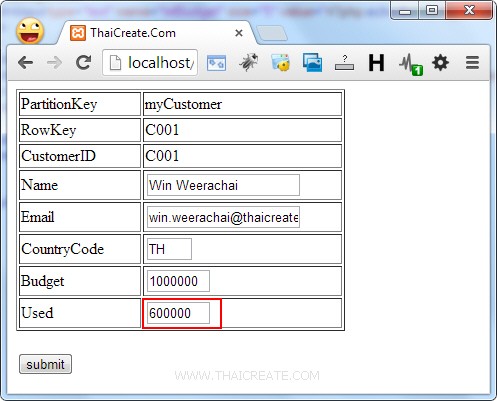
แสดงข้อมูลบน Form ที่จะ Update เราจะทดสอบการแก้ไขค่า Used ซึ่งปัจจุบันเป็น 60000

แก้ไขเป็นยอดใหม่ 90000 และเลือก Submit เพื่อทำการแก้ไขข้อมูล
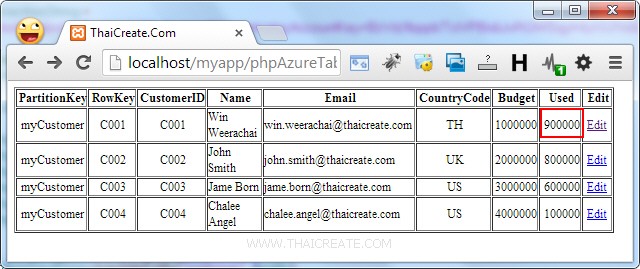
แก้ไขข้อมูลเรียบร้อยแล้ว ข้อมูลจะมีการ Update ทันที
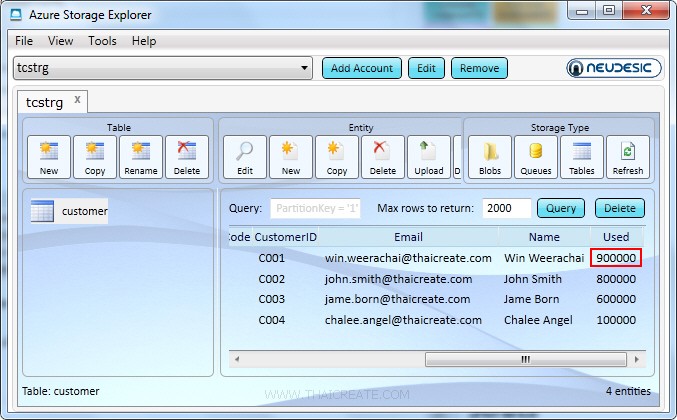
เมื่อเข้าไปดูใน Table Storage บน Windows Azure ผ่านโปรแกรม Azure Storage Explorer ข้อมูลจะมีการแก้ไข Update ตามที่เราแก้ไขไปก่อนหน้านี้
บทความที่เกี่ยวข้อง
บทความถัดไปที่แนะนำให้อ่าน
|