ตอนที่ 4 : การนำข้อมูลจาก Azure Mobile Services มาแสดงบน Windows Store App |
ตอนที่ 4 : การนำข้อมูลจาก Azure Mobile Services มาแสดงบน Windows Store App บทความนี้จะเป็นการใช้ Windows Store App อ่านข้อมูลจาก Table ของ Azure Mobile Services ที่อยู่บน Windows Azure โดยข้อมูลของ Table ได้ถูกจัดเก็บแบบ Column และ Rows คล้าย ๆ กับ Table ของ SQL Database ทั่ว ๆ ไป และใน Windows Store App จะมีการใช้ Listbox ซึ่งจะแสดงข้อมูลที่อยู่ในรูปแบบของ Object ด้วยการกำหนด DataSource ข้อมูลและนำข้อมูลที่ได้แสดงผลรายการใน Column และ Rows ของ Listbox
รูปแบบการอ่านข้อมูลจาก Table ของ Mobile Services ด้วย Windows Store App
private MobileServiceCollection<MyMember, MyMember> items;
private IMobileServiceTable<MyMember> memberTable = App.MobileService.GetTable<MyMember>();
items = await memberTable.ToCollectionAsync();
รูปแบบการใช้ Windows Store App อ่านข้อมูลจาก Mobile Services บน Windows Azure
การ Bind ข้อมูล DataSource ให้กับ Listbox
myListbox.ItemsSource = items;

ตอนนี้เรามี Mobile Services อยู่ 1 รายการ
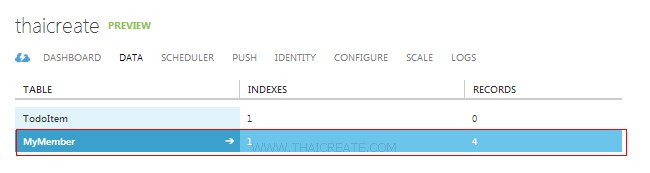
อตารางว่า MyMember
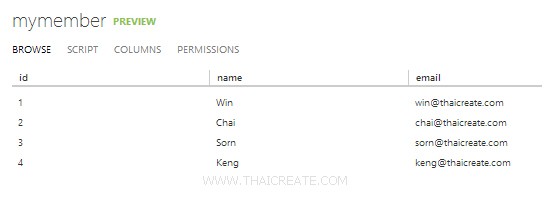
ข้อมูลในตาราง MyMember ประกอบด้วย Column ชื่อว่า id,name,email
กลับมายังหน้า Project บน Visual Studio
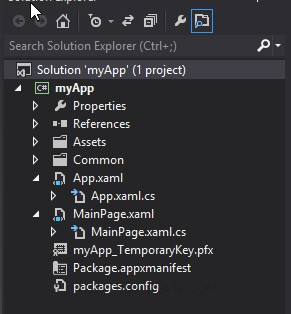
โครงสร้างไฟล์
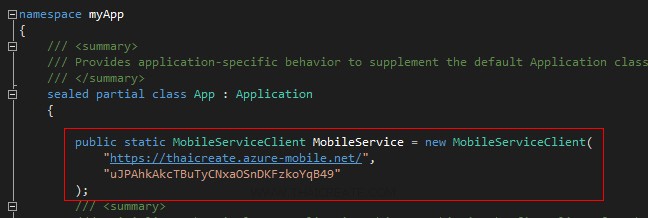
sealed partial class App : Application
{
public static MobileServiceClient MobileService = new MobileServiceClient(
"https://thaicreate.azure-mobile.net/",
"uJPAhkAkcTBuTyCNxaOSnDKFzkoYqB49"
);
ในไฟล์ App.xaml.cs ให้กำหนด Url และ Key ลงไป

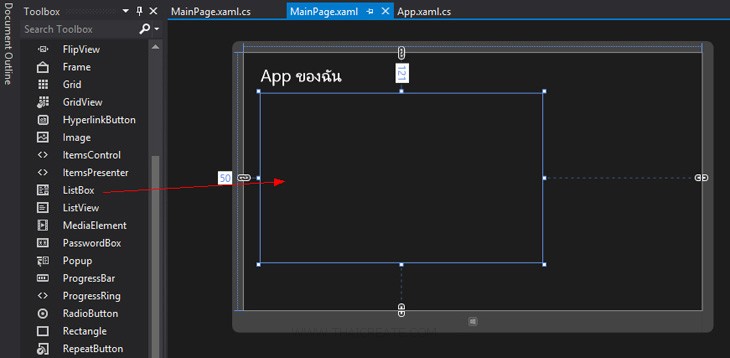
ในหน้า Layout สร้าง Listbox สำหรับแสดงข้อมูล โดยมี Code ดังนี้
MainPage.xaml
<Page
x:Class="myApp.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:myApp"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<TextBlock HorizontalAlignment="Left" Margin="50,41,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="50" Width="355" Height="80">
<Run Text="App ของฉัน"/>
<LineBreak/>
<Run/>
</TextBlock>
<ListBox x:Name="myListbox" HorizontalAlignment="Left" Height="504" Margin="50,121,0,0" VerticalAlignment="Top" Width="840" RenderTransformOrigin="-0.055,-1.65" Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal" Margin="0,0,0,17">
<StackPanel Width="50">
<TextBlock Text="{Binding Id}" TextWrapping="Wrap" FontSize="35" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="150">
<TextBlock Text="{Binding Name}" TextWrapping="Wrap" FontSize="35" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="400">
<TextBlock Text="{Binding Email}" TextWrapping="Wrap" FontSize="35" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</Grid>
</Page>
จากนั้นเขียน Code ดังนี้
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using Microsoft.WindowsAzure.MobileServices;
using Newtonsoft.Json;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace myApp
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public class MyMember
{
public int Id { get; set; }
[JsonProperty(PropertyName = "name")]
public string Name { get; set; }
[JsonProperty(PropertyName = "email")]
public string Email { get; set; }
}
public sealed partial class MainPage : Page
{
private MobileServiceCollection<MyMember, MyMember> items;
private IMobileServiceTable<MyMember> memberTable = App.MobileService.GetTable<MyMember>();
public MainPage()
{
this.InitializeComponent();
}
private async void RefreshMemberItems()
{
try
{
items = await memberTable.ToCollectionAsync();
}
catch (MobileServiceInvalidOperationException e)
{
throw e;
}
myListbox.ItemsSource = items;
}
/// <summary>
/// Invoked when this page is about to be displayed in a Frame.
/// </summary>
/// <param name="e">Event data that describes how this page was reached. The Parameter
/// property is typically used to configure the page.</param>
protected override void OnNavigatedTo(NavigationEventArgs e)
{
RefreshMemberItems();
}
}
}
Screenshot
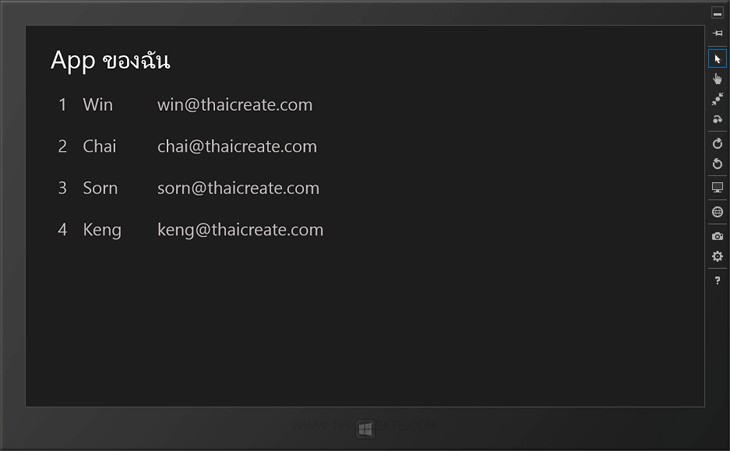
แสดงข้อมูลจาก Table ของ Mobile Services บน Windows Store App
|