Windows Store App and Binding ListView/ListBox from JSON (C#) |
Windows Store App and Binding ListView/ListBox from JSON (C#) บทความนี้จะเป็นเทคนิคการเขียน Windows Store Apps กับการ Binding Data ข้อมูลบน ListBox และ ListView โดยอาศัยแหล่งข้อมูลจาก Web Server ที่ถูกส่งมาจาก HTTP / URL ในรูปแบบของ JSON ซึ่งข้อมูลเหล่านี้จะมาจาก PHP กับ MySQL Database ที่ทำงานอยู่บน Web Server
ฝั่ง Web Server (PHP/MySQL)
MySQL Table บน Web Server
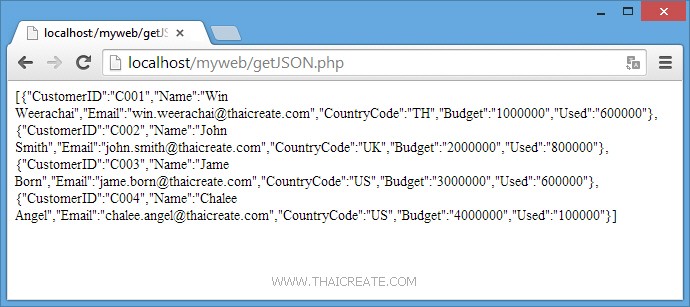
CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
PHP ที่ทำหน้าที่อ่านข้อมูล MySQL Database และแปลงเป็น JSON
getJSON.php
<?php
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM customer WHERE 1 ";
$objQuery = mysql_query($strSQL);
$intNumField = mysql_num_fields($objQuery);
$resultArray = array();
while($obResult = mysql_fetch_array($objQuery))
{
$arrCol = array();
for($i=0;$i<$intNumField;$i++)
{
$arrCol[mysql_field_name($objQuery,$i)] = $obResult[$i];
}
array_push($resultArray,$arrCol);
}
mysql_close($objConnect);
echo json_encode($resultArray);
?>
เมื่อทดสอบเรียก PHP เราจะได้ Result ที่เป็น JSON ดังนี้
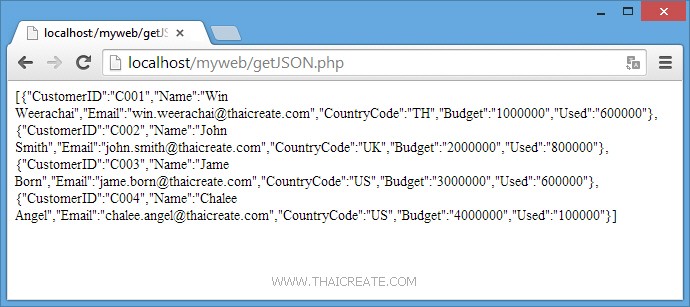
[
{"CustomerID":"C001","Name":"Win Weerachai","Email":"[email protected]" ,"CountryCode":"TH","Budget":"1000000","Used":"600000"},
{"CustomerID":"C002","Name":"John Smith","Email":"[email protected]" ,"CountryCode":"UK","Budget":"2000000","Used":"800000"},
{"CustomerID":"C003","Name":"Jame Born","Email":"[email protected]" ,"CountryCode":"US","Budget":"3000000","Used":"600000"},
{"CustomerID":"C004","Name":"Chalee Angel","Email":"[email protected]" ,"CountryCode":"US","Budget":"4000000","Used":"100000"}
]
ฝั่ง Windows Store Apps (C#)
สร้าง Class สำหรับ Mapping Column
public class myCustomer
{
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
สร้างชุดคำสั่งการเชื่อมต่อกับ HTTP และการอ่านค่า Result จาก URL
HttpClient http = new System.Net.Http.HttpClient();
HttpResponseMessage response = await http.GetAsync("http://localhost/myweb/getJSON.php");
response.EnsureSuccessStatusCode();
string resultJSON = string.Empty;
resultJSON = await response.Content.ReadAsStringAsync();
สร้างชุดคำสั่งการ Decode JSON และการ Binding Data กับ ListView หรือ ListBox
MemoryStream ms = new MemoryStream(Encoding.UTF8.GetBytes(resultJSON));
ObservableCollection<myCustomer> list = new ObservableCollection<myCustomer>();
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(ObservableCollection<myCustomer>));
list = (ObservableCollection<myCustomer>)serializer.ReadObject(ms);
this.myListBox.ItemsSource = list;
ลองมาดูตัวอย่างแบบเต็ม ๆ
Example 1 การเขียน Windows Store Apps เพื่ออ่านค่า JSON และ Binding Data ให้กับ ListBox
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<ListBox x:Name="myListBox" HorizontalAlignment="Left" Height="453" Margin="58,98,0,0" VerticalAlignment="Top" Width="989">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal" Margin="0,0,0,17">
<StackPanel Width="80">
<TextBlock Text="{Binding CustomerID}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0" FontFamily="Global User Interface"/>
</StackPanel>
<StackPanel Width="150">
<TextBlock Text="{Binding Name}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="300">
<TextBlock Text="{Binding Email}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="50">
<TextBlock Text="{Binding CountryCode}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Budget}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Used}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.Net.Http;
using System.Xml.Linq;
using System.Runtime.Serialization;
using System.Runtime.Serialization.Json;
using System.Collections.ObjectModel;
using System.Text;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
public class myCustomer
{
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
HttpClient http = new System.Net.Http.HttpClient();
HttpResponseMessage response = await http.GetAsync("http://localhost/myweb/getJSON.php");
response.EnsureSuccessStatusCode();
string resultJSON = string.Empty;
resultJSON = await response.Content.ReadAsStringAsync();
MemoryStream ms = new MemoryStream(Encoding.UTF8.GetBytes(resultJSON));
ObservableCollection<myCustomer> list = new ObservableCollection<myCustomer>();
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(ObservableCollection<myCustomer>));
list = (ObservableCollection<myCustomer>)serializer.ReadObject(ms);
this.myListBox.ItemsSource = list;
}
}
}
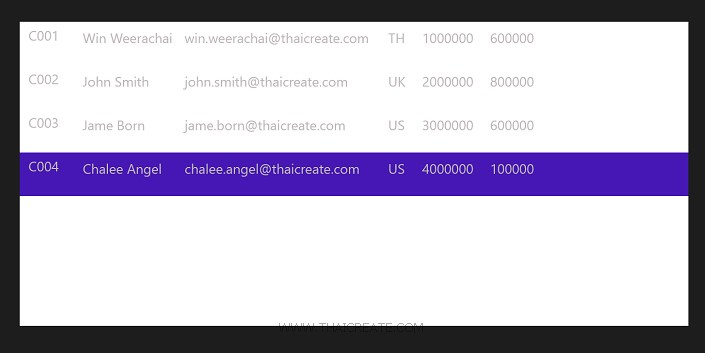
ผลลัพธ์ที่ได้แสดงบน ListBox
Example 2 การเขียน Windows Store Apps เพื่ออ่านค่า JSON และ Binding Data ให้กับ ListView
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<ListView x:Name="myListView" HorizontalAlignment="Left" Height="453" Margin="58,98,0,0" VerticalAlignment="Top" Width="989">
<ListView.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal" Margin="0,0,0,17">
<StackPanel Width="80">
<TextBlock Text="{Binding CustomerID}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0" FontFamily="Global User Interface"/>
</StackPanel>
<StackPanel Width="150">
<TextBlock Text="{Binding Name}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="300">
<TextBlock Text="{Binding Email}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="50">
<TextBlock Text="{Binding CountryCode}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Budget}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Used}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
</StackPanel>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.Net.Http;
using System.Xml.Linq;
using System.Runtime.Serialization;
using System.Runtime.Serialization.Json;
using System.Collections.ObjectModel;
using System.Text;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
public class myCustomer
{
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
HttpClient http = new System.Net.Http.HttpClient();
HttpResponseMessage response = await http.GetAsync("http://localhost/myweb/getJSON.php");
response.EnsureSuccessStatusCode();
string resultJSON = string.Empty;
resultJSON = await response.Content.ReadAsStringAsync();
MemoryStream ms = new MemoryStream(Encoding.UTF8.GetBytes(resultJSON));
ObservableCollection<myCustomer> list = new ObservableCollection<myCustomer>();
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(ObservableCollection<myCustomer>));
list = (ObservableCollection<myCustomer>)serializer.ReadObject(ms);
this.myListView.ItemsSource = list;
}
}
}
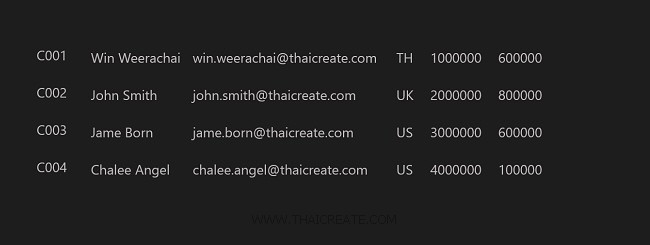
ผลลัพธ์ที่ได้แสดงบน ListView
.
|