C++ และทดสอบสร้าง Project ของ Windows Store Apps ด้วย C++ |
C++ และทดสอบสร้าง Project ของ Windows Store Apps ด้วย C++ สำหรับภาษา C++ เชื่อว่าหลาย ๆ คนอาจจะยังไม่คุ้น และได้รับความนิยมค่อนข้างน้อยมากในปัจจุบัน ส่วนหนึ่งเพราะภาษา C++ มีโครงสร้างที่ค่อนข้างจะซับซ้อนมากกว่าภาษา C# หรือ VB.Net แต่ภาษา C++ เป็นภาษาที่มีความสามารถค่อนข้างสูง สามารถรองรับการเขียนโปรแกรมในระดับภาษาเครื่องได้ ฉะนั้นจึงเหมาะสำหรับการนำมาเขียนอะไรที่ต้องการความซับซ้อน เช่น การเขียน DirectX ประเภท Game , Graphic และ Multimedia และใน Windows Store Apps ก็ได้จัดให้ C++ ในการเขียน Application เกี่ยวกับด้านนี้โดยเฉพาะ
Windows Store Apps ด้วย C++
นอกจากจะเขียน Apps พวก Game ประเภท DirectX แล้ว C++ ยังสามารถเขียน Windows Store Apps ทั่ว ๆ ไป ได้เช่นเดียวกัน โดยจะใช้ Interface ของ XAML เหมือนกับภาษา VB.Net และ C# เรามาลองดูตัวอย่างการเขียนแบบง่าย ๆ
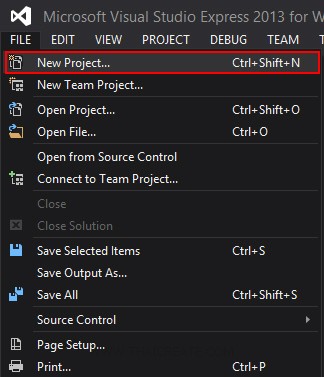
เปิดโปรแกรม Visual Studio เลือก FILE -> New Project....
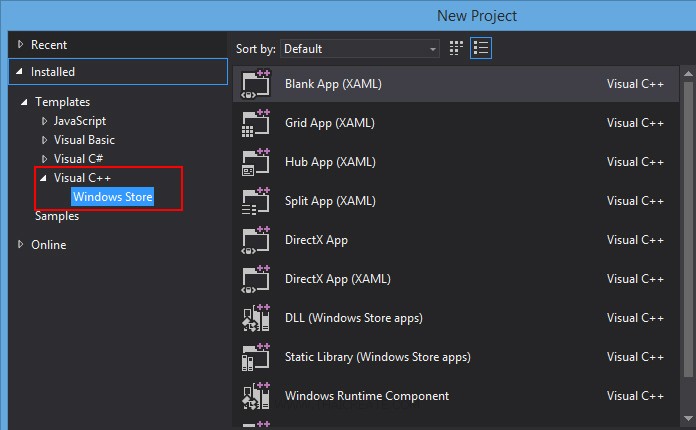
เลือก Visual C++ -> Windows Store
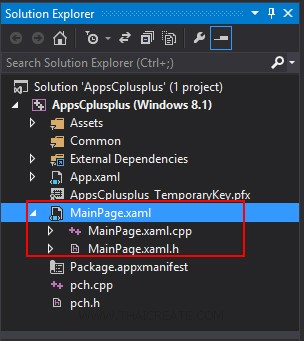
เราจะได้โครงสร้างของ Project ดังรูป ซึ่งไฟล์ที่เราจะให้ความสนใจก็คือ MainPage.xaml , MainPage.xaml.h และ MainPage.xaml.cpp ซึ่งเป็นไฟล์แรกของ Apps ที่จะทำงานเมื่อมีการ Run โปรแกรม
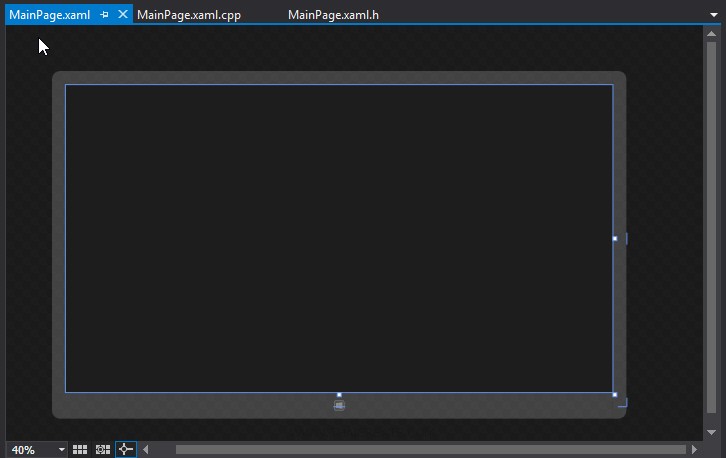
คลิกที่ MainPage.xaml จะได้หน้าจอดังรูป ซึ่งไฟล์ MainPage.xaml เป็น Layout Design หรือ หน้าจอของ Apps ที่เราจะออกแบบโปรแกรมต่าง ๆ โดยเราจะเรียกส่วนนี้ว่า Page ซึ่งพวก Page นี้จะใช้ XAML เป็น Tags สำหรับการสร้าง Control ต่าง
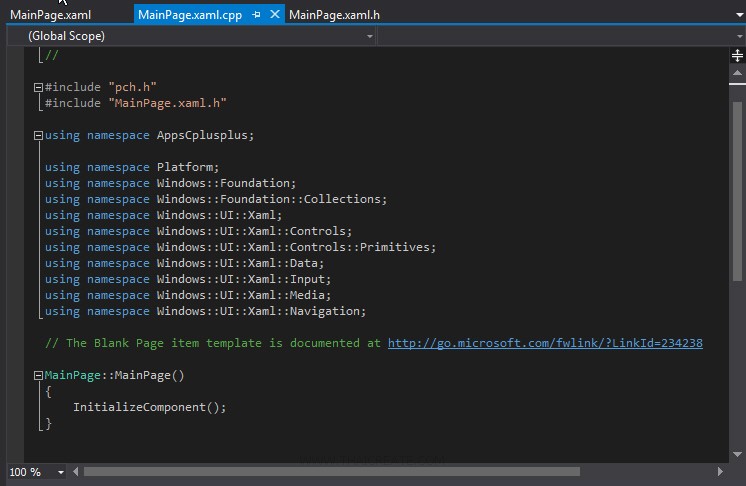
ไฟล์ MainPage.xaml.cpp เป็นไฟล์สำหรับ Coding ของภาษา C++ ซึ่งทำงานอยู่เบื้องหลัง ควบคุมการทำงานต่าง ๆ ของหน้าจอในหน้า MainPage.xaml
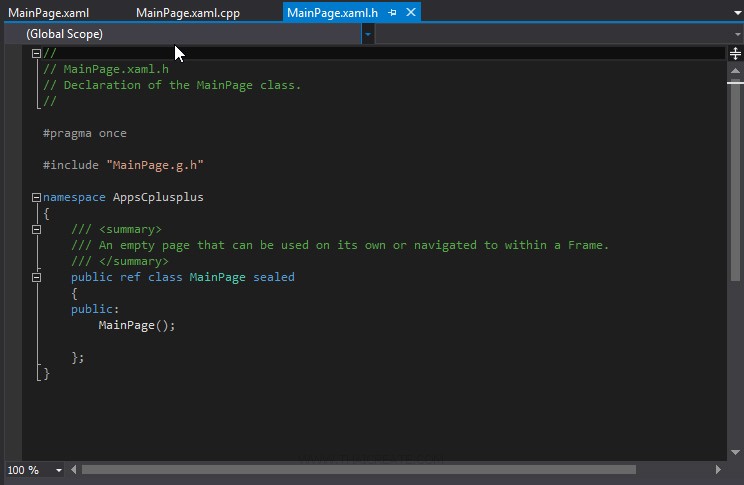
และไฟล์ MainPage.xaml.h เป็นไฟล์ header ของภาษา C++ ใช้สำหรับประกาศพวกค่าต่าง ๆ ซึ่งจะทำงานร่วมกับ MainPage.xaml.cpp
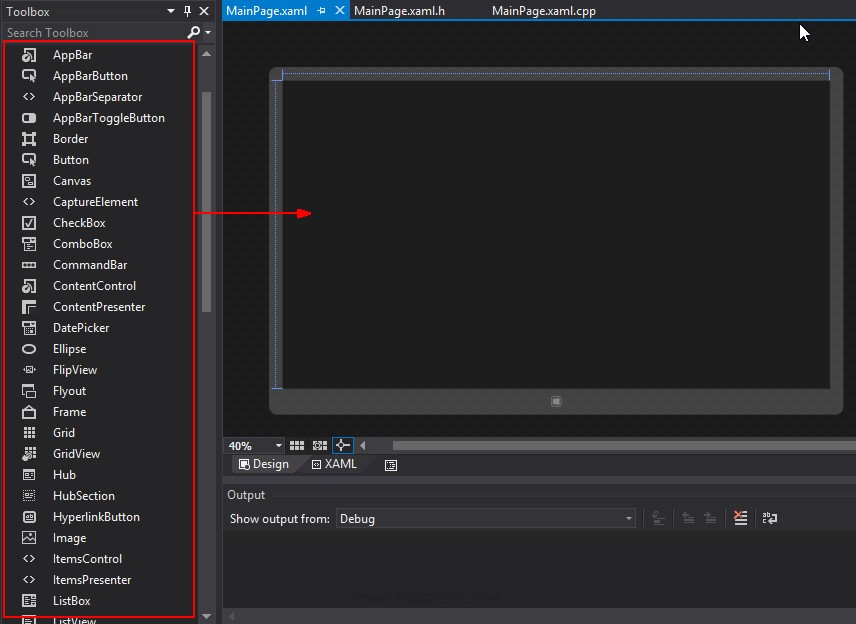
ฝั่งซ้ายเราจะเห็นรายการ Controls ต่าง ๆ ที่สามารถใช้ออกแบบหน้าจอบนหน้า Page โดยเมื่อ Control ถูกสร้างในหน้่า Page จะมีการ Generate ตัว Tags ของ XAML มาให้ด้วย
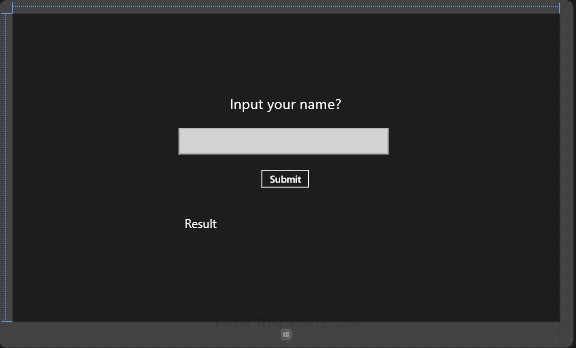
ทดสอบออกแบบหน้าจอดังรูป ซึ่งประกอบด้วย TextBlock , TextBox และ Button
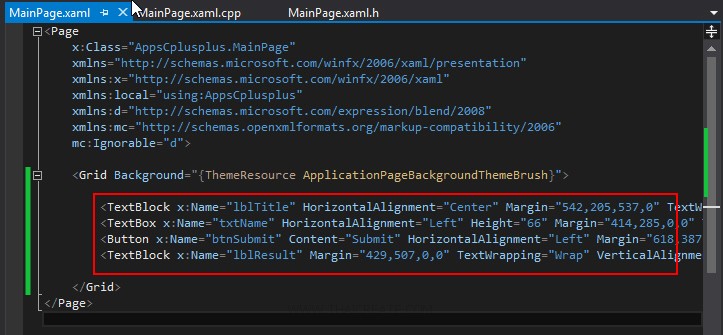
Tags ของ XAML ที่ถูกสร้างหลังจากการ สร้าง Controls ซึ่งเราสามารถแก้ไขรายละเอียดต่าง ๆ ได้ผ่านส่วนของ XAML ได้เช่นเดียวกัน
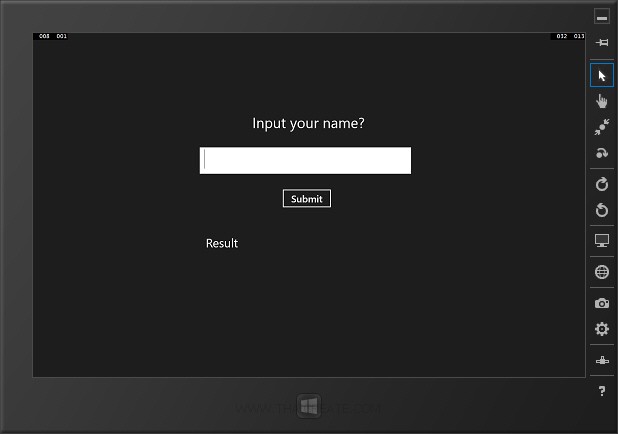
เราจะได้ Code ดังนี้
MainPage.xaml
<Page
x:Class="AppsCplusplus.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:AppsCplusplus"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblTitle" HorizontalAlignment="Center" Margin="542,205,537,0" TextWrapping="Wrap" Text="Input your name?" VerticalAlignment="Top" Height="43" Width="287" FontSize="36"/>
<TextBox x:Name="txtName" HorizontalAlignment="Left" Height="66" Margin="414,285,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="525" FontFamily="Global User Interface" FontSize="36"/>
<Button x:Name="btnSubmit" Content="Submit" HorizontalAlignment="Left" Margin="618,387,0,0" VerticalAlignment="Top" Height="50" Width="125" FontSize="24" Click="btnSubmit_Click"/>
<TextBlock x:Name="lblResult" Margin="429,507,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="30" Width="510" RenderTransformOrigin="0.035,0.558" HorizontalAlignment="Left" Text="Result"/>
</Grid>
</Page>
MainPage.xaml.h
//
// MainPage.xaml.h
// Declaration of the MainPage class.
//
#pragma once
#include "MainPage.g.h"
namespace AppsCplusplus
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public ref class MainPage sealed
{
public:
MainPage();
private:
void btnSubmit_Click(Platform::Object^ sender, Windows::UI::Xaml::RoutedEventArgs^ e);
};
}
MainPage.xaml.cpp
//
// MainPage.xaml.cpp
// Implementation of the MainPage class.
//
#include "pch.h"
#include "MainPage.xaml.h"
using namespace AppsCplusplus;
using namespace Platform;
using namespace Windows::Foundation;
using namespace Windows::Foundation::Collections;
using namespace Windows::UI::Xaml;
using namespace Windows::UI::Xaml::Controls;
using namespace Windows::UI::Xaml::Controls::Primitives;
using namespace Windows::UI::Xaml::Data;
using namespace Windows::UI::Xaml::Input;
using namespace Windows::UI::Xaml::Media;
using namespace Windows::UI::Xaml::Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
MainPage::MainPage()
{
InitializeComponent();
}
void AppsCplusplus::MainPage::btnSubmit_Click(Platform::Object^ sender, Windows::UI::Xaml::RoutedEventArgs^ e)
{
this->lblResult->Text = "Sawatdee Khun " + this->txtName->Text;
}
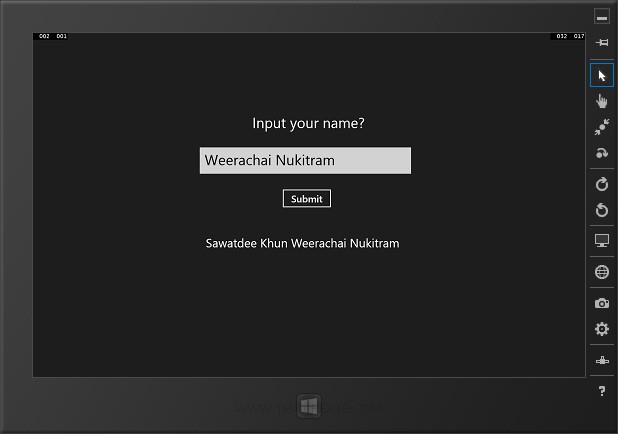
ทดสอบการรันผ่าน Simulator ทดสอบกรอกชื่อและคลิกที่ Button โปรแกรมจะแสดงโต้ตอบ
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2014-02-01 14:46:01 /
2017-03-19 14:37:02 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|