Windows Store App and HTTP Connect to HTML / Web URL (C#) |
Windows Store App and HTTP Connect to HTML / Web URL (C#) ในการเขียนโปรแกรมบน Windows Store Apps เพื่อติดต่ออ่านค่าต่าง ๆ ที่อยู่ใน Server นั้น สามารถทำได้ง่าย ๆ ด้วยการใช้ System.Net.Http ซึ่งเป็น Class Library ไว้สำหรับการติดต่อกับ HTTP ผ่าน Web URL ทั้งแบบ Local Server และ WWW Server โดยการอ่านวิธีนี้ในฝั่ง Web Server จะต้องสร้างเป็น URL สำหรับทำการแสดงข้อมูล และ Windows Store Apps จะอ่านข้อมูลจาก URL นั้น ๆ โดยส่ง Request ไปยัง Web Server และ Web Server จะ Response ค่าออกมา จะได้รูปแบบที่ต้องการออกมาเป็นข้อความหรือ String ซึ่งในฝั่งของ Web Server จะทำงานด้วยภาษาอะไรก็ตาม เช่น PHP/ASP/ASP.NET หรือ JSP
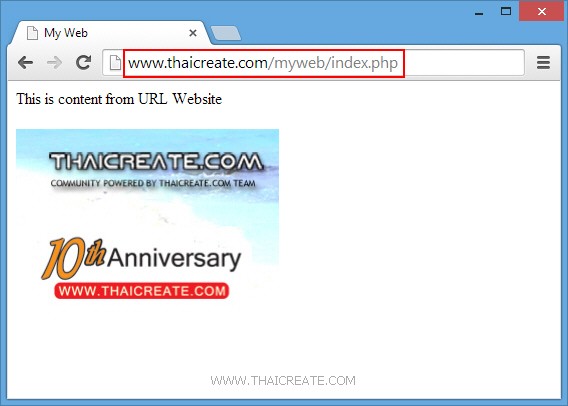
ทดสอบการเรียกจาก Web Server ซึ่งเมื่อแสดงผลบน Web Browser เราจะเห็นเป็น HTML result ที่ถูกแสดงผลบน Web Browser
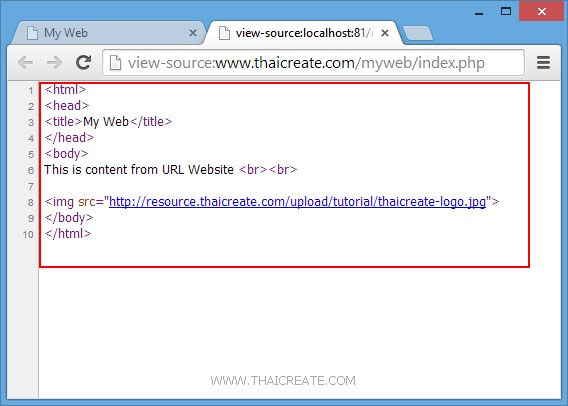
แต่ในมุมมองการรับส่งจะอยู่ในรูปแบของ HTML Tag
HttpClient http = new System.Net.Http.HttpClient();
HttpResponseMessage response = await http.GetAsync("http://localhost/myweb/index.php");
response.EnsureSuccessStatusCode();
//this.lblStatus.Text = response.StatusCode + " " + response.ReasonPhrase + Environment.NewLine;
string result = string.Empty;
result = await response.Content.ReadAsStringAsync();
this.lblResult.Text = result;
คำสั่งรูปแบบการเรียกข้อมูลที่อยู่ในรูปแบบของ URL ซึ่งผลลัพธ์ที่ได้จะแสดงในรูปแบบของ HTML Tags
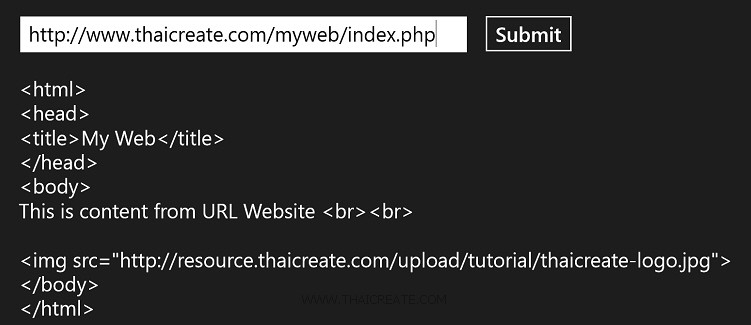
แต่ในกรณีที่ต้องการแสดงผลให้เหมือนกับผลลัพธ์ที่อยู่บน Web Browser เราสามารถใช้ Control ที่มีชื่อว่า WebView ซึ่งจะแสดงผลได้เช่นเดียวกับ Web Browser ทั่ว ๆ ไป ลองมาดูใตัวอย่างแรก
Example การเรียกข่อมูลจาก URL ในรูปแบบของ HTML Tag และแสดงผลบน WebView
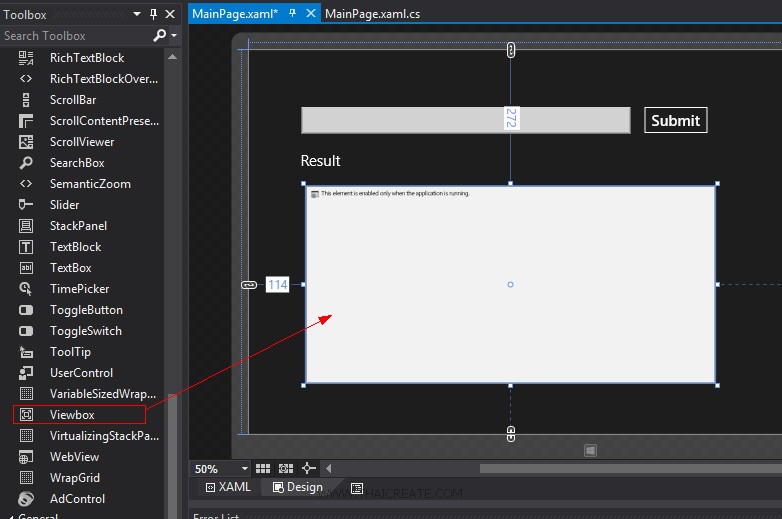
สร้างหน้าจอดังรูป ซึ่งประกอบด้วย TextBox , Button และ WebView
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblResult" HorizontalAlignment="Left" Margin="103,205,0,0" TextWrapping="Wrap" Text="Result" FontSize="30" VerticalAlignment="Top"/>
<TextBox x:Name="txtURL" HorizontalAlignment="Left" Margin="105,114,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="658" FontSize="30" Height="53"/>
<Button x:Name="btnSubmit" Content="Submit" HorizontalAlignment="Left" Margin="788,111,0,0" VerticalAlignment="Top" FontSize="30" Click="btnSubmit_Click"/>
<WebView x:Name="myWebView" HorizontalAlignment="Left" Height="394" Margin="114,272,0,0" VerticalAlignment="Top" Width="818"/>
</Grid>
</Page>
และในส่วนของ C# นั้นเราจะทำค่า Result ที่ได้แสดงผลผ่าน WebView เช่น
result = await response.Content.ReadAsStringAsync();
this.myWebView.NavigateToString(result);
การนำ Result ที่ได้แสดงผ่าน WebView
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.Net.Http;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private async void btnSubmit_Click(object sender, RoutedEventArgs e)
{
try
{
HttpClient http = new System.Net.Http.HttpClient();
HttpResponseMessage response = await http.GetAsync(this.txtURL.Text);
response.EnsureSuccessStatusCode();
//this.lblStatus.Text = response.StatusCode + " " + response.ReasonPhrase + Environment.NewLine;
string result = string.Empty;
result = await response.Content.ReadAsStringAsync();
this.myWebView.NavigateToString(result);
}
catch (HttpRequestException hre)
{
//this.lblStatus.Text = hre.ToString();
}
catch (Exception ex)
{
// For debugging
//this.lblStatus.Text = ex.ToString();
}
}
}
}
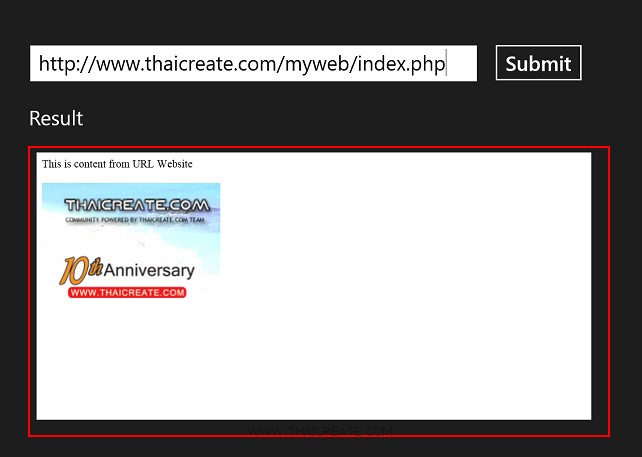
ทดสอบการทำงาน ซึ่งจะเห็นว่า Windows Store Apps สามารถแสดงผลลัพธ์ที่ถูกส่งมาในรูปแบบของ HTML Tag ได้เช่นเดียวกับ Web Browser
นอกนากนี้ WebView ยังสามารถแสดงผลลัพธ์ของ URL ได้ทันที เดียวที่ไม่ต้องส่งมาในรูปแบบของ URL ได้เช่นกัน
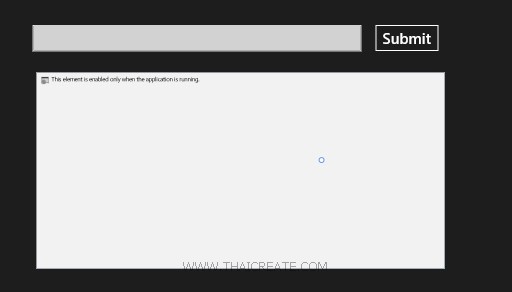
สร้าง Apps หน้าดังรูป
<TextBox x:Name="txtURL" HorizontalAlignment="Left" Margin="105,114,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="658" FontSize="30" Height="53"/>
<Button x:Name="btnSubmit" Content="Submit" HorizontalAlignment="Left" Margin="788,111,0,0" VerticalAlignment="Top" FontSize="30" Click="btnSubmit_Click"/>
<WebView x:Name="myWebView" HorizontalAlignment="Left" Height="394" Margin="112,208,0,0" VerticalAlignment="Top" Width="818"/>
this.myWebView.Navigate(new Uri(this.txtURL.Text, UriKind.Absolute));
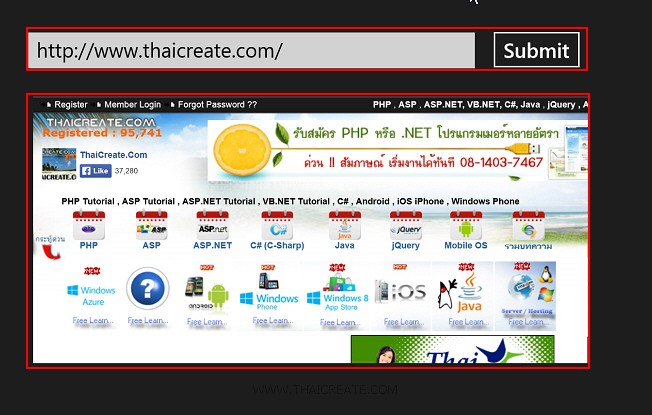
ตัวอย่างการแสดง URL บน WebView
แต่ทั้งนี้การส่งมาในรูปแบบของ HTML Tag หรือ HTML Result จะใช้ในการณีที่ต้องการรับส่งค่า Result ที่ได้จาก Web Server เช่น ข้อมูลในรูปแบบของ String , XML หรือ JSON โดยที่ข้อมูลเหล่านี้สามารถนำไปใช้งานในด้านอื่น ๆ ของ Apps ได้ในรูปแบบต่าง ๆ ซึ่งจะได้อ่านได้จากในหัวข้อถัดไป
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2014-05-26 12:12:11 /
2017-03-19 15:06:29 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|