Windows Store App and Connect to localhost Server (C#) |
Windows Store App and Connect to localhost Server (C#) ยุคของการเขียนโปรแกรมเปลี่ยนไปจากแบบเดิมมาก เพราะแต่ก่อนนี้โปรแกรมทั่ว ๆ ไปจะเน้นการทำงานบน Local Database ผ่าน Database ทั่ว ๆ ไป เช่น MS Access , MySQL , SQL Server หรืออื่น ๆ แต่บนโปรแกรมบน Windows Store Apps จะเน้นการทำงานที่เป็น Online มากขึ้น และ App ก็มีความกระฉับกว่าเดิม ไม่ต้องอาศัยการติดตั้ง Database ไปด้วย ฉะนั้นรูปแบบการเรียกใช้งานร่วมกับ Database จึงจะต้องอาศัยการทำงานในรูปแบบของ API โดยอาศัยพวกเทคโนโลยี่ HTTP ,REST หรือ Web Services เป็นต้น ซึ่งเทคโนโลยี่เหล่านี้ เราสามารถช่องทางการใช้งานได้ด้วยตัวเอง บน Server แล้วติดตั้งโปรแกรมประเภท Web Server จากนั้นก็สร้าง Application ที่ทำหน้าที่อยู่บน Server คอยรับส่งข้อมูลระหว่าง Apps กับ Server
โดย Web Server ที่ได้รับความนิยมหลัก ๆ จะมีอยู่ 2 ตัวคือ IIS กับ Apache โดย IIS สามารถติดตั้งได้บน Windows Server ส่วน Apache มี Version ทั้งที่รันบน Windows Server และ Linux Server
Apache Web Server ทำงานได้ทั้งบน Windows และ Linux
IIS (Internet Information Services) ทำงานบน Windows OS
สำหรับวิธีการติดตั้งนั้นสามารถหาอ่านได้จากบทความในเว็บ ซึ่งทางทีมงานได้เขียนไว้หลายบทความมาก
ทำความเข้าใจเกี่ยวกับ การเชื่อมต่อไปยัง localhost ของ Server ซะก่อน ซึ่งในบทความนี้จะยกตัวอย่างการติดตั้งโปรแกรม Web Server ไว้เครื่องเดียวกับ App ซึ่งสามารถเรียกว่า Server ชื่อว่า localhost แต่การใช้งานจริงนั้น เราสามารถติดตั้งผ่านเครื่องอื่น ๆ ทั้ง Network ที่อยู่บนระบบ Lan หรือ Internet หรืออื่น ๆ และเรียกใช้งานตามขอบเขตที่สามารถใช้งานได้

Example การทดสอบเรียก localhost ที่อยู่บน Local Server
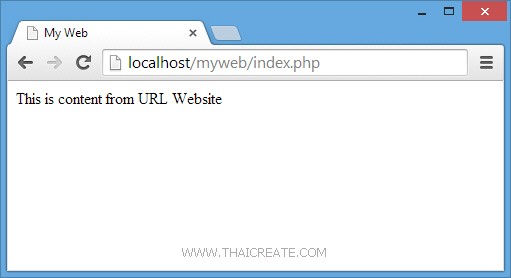
จากตัวอย่างนี้มีการเรียกใช้ HTTP URL ของ localhost เช่น
http://localhost/myweb/index.php
ซึ่งจะแสดงข้อความผลลัพธ์ที่ถูกส่งมาจาก Local Server
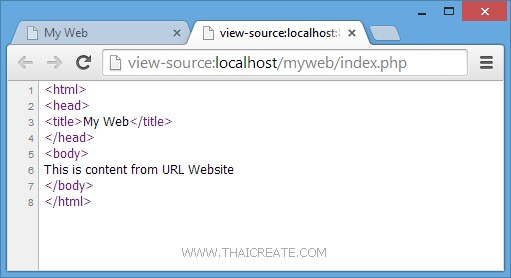
ข้อมูบที่ถูกส่งมาจาก HTTP จะอยู่ในรูปแบบของ HTML Tag แต่ตอนที่แสดงผลบน Web Browser เราจะมองไม่เห็น HTML Tag เหล่านั้น ซึ่งจะต้องใช้การ View Source
กลับมายังหน้าจอ Apps บน Windows Store Apps ให้ออกแบบหน้าจอดังรูป
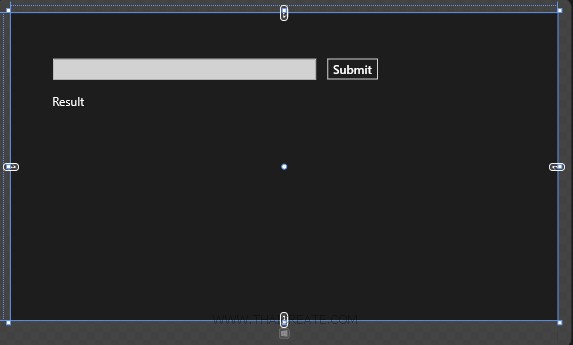
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblResult" HorizontalAlignment="Left" Margin="103,205,0,0" TextWrapping="Wrap" Text="Result" FontSize="30" VerticalAlignment="Top"/>
<TextBox x:Name="txtURL" HorizontalAlignment="Left" Margin="105,114,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="658" FontSize="30" Height="53"/>
<Button x:Name="btnSubmit" Content="Submit" HorizontalAlignment="Left" Margin="788,111,0,0" VerticalAlignment="Top" FontSize="30" Click="btnSubmit_Click"/>
</Grid>
</Page>
และในส่วนของ C# นั้นเราจะเรียกใช้ NameSpace ที่มีชื่อว่า System.Net.Http ซึ่งเป็น Library ที่ใช้จัดการและเชื่อมต่อกับ Protocal HTTP
using System.Net.Http;
เรียกใช้ System.Net.Http;
HttpClient http = new System.Net.Http.HttpClient();
HttpResponseMessage response = await http.GetAsync("http://localhost/myweb/index.php");
response.EnsureSuccessStatusCode();
string result = await response.Content.ReadAsStringAsync();
เป็น Code สำหรับการเชื่อมต่อไปยัง URL และการอ่านค่า Result ที่ได้
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.Net.Http;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private async void btnSubmit_Click(object sender, RoutedEventArgs e)
{
HttpClient http = new System.Net.Http.HttpClient();
HttpResponseMessage response = await http.GetAsync(this.txtURL.Text);
response.EnsureSuccessStatusCode();
//lblResult.Text = response.StatusCode + " " + response.ReasonPhrase + Environment.NewLine;
string result = string.Empty;
string result = await response.Content.ReadAsStringAsync();
result = result.Replace("<br>", Environment.NewLine); // Insert new lines
this.lblResult.Text = result;
}
}
}
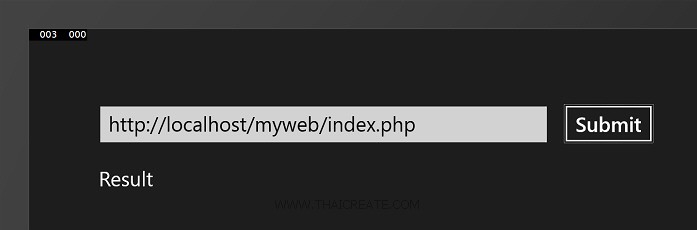
ทดสอบการทำงานเพื่ออ่าค่าจาก URL ที่อยู่บน Local Server

ผลลัพธ์ที่ได้ ซึ่งจะได้เหมือนกับ ผลลัพธ์ที่อยู่บน Web Browser
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2014-05-26 11:33:15 /
2017-03-19 14:48:55 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|