Windows Store App and Downloading & Progress Bar (C#) |
Windows Store App and Downloading & Progress Bar (C#) บทความนี้จะเป็นตัวอย่างการเขียน Windows Store Apps เพื่อ Download ไฟล์จาก Server ผ่าน HTTP และในระหว่างขั้นตอนการ Download นั้น ได้มีการนำ Control ของ ProgressBar มาแสดงสถานะ % ในขณะที่กำลัง Download ว่าในขณะที่กำลังดาวน์โหลดอยู่นั้นทำงานได้กี่แล้ว % เหมาะสำหรับการทำงานกับไฟล์ที่มีขนาดใหญ่ และสามารถใช้ได้กับไล์ทุกประเภท
รูปแบบการ Download ไฟล์จาก Server
Uri source = new Uri(this.txtURL.Text);
string destination = "myZip.zip";
Windows.Storage.StorageFolder localFolder = Windows.Storage.ApplicationData.Current.LocalFolder;
StorageFile destinationFile = await localFolder.CreateFileAsync(destination, CreationCollisionOption.ReplaceExisting);
BackgroundDownloader downloader = new BackgroundDownloader();
DownloadOperation download = downloader.CreateDownload(source, destinationFile);
await HandleDownloadAsync(download);
จาก Code จะมีการ Download ไฟล์และจัดเก็บไว้ใน Local Storage โดยมี Method ที่ Handle ควบคุมสถานะการทำงานชื่อว่า HandleDownloadAsync() และเราสามารถเขียนตรวจสอบสถานะได้ดังนี้
private async Task HandleDownloadAsync(DownloadOperation downloadOperation)
{
activeDownload = downloadOperation;
var progress = new Progress<DownloadOperation>(ProgressCallback);
await downloadOperation.StartAsync().AsTask(progress);
}
ในระหว่างการทำงานนั้นสามารถส่ง Call back เพื่อไป Update สถานะของ ProgressBar
private void ProgressCallback(DownloadOperation obj)
{
double progress
= ((double)obj.Progress.BytesReceived / obj.Progress.TotalBytesToReceive);
DownloadProgress.Value = progress * 100;
if (progress >= 1.0)
{
activeDownload = null;
}
}
ในส่วนของ Method ที่ทำหน้าที่ Call back ก็จะคำนวณจำนวน Byte ที่ Download เรียบร้อยแล้ว ซึ่งเราจะได้ทำนวน % ที่มีค่า 0-100% ไป Update ให้กับ ProgressBar ลองมาดูตัวอย่างแบบเต็ม ๆ
Example การเขียน Windows Store Apps แสดงการ Download และสถานะการ Download ด้วย ProgressBar
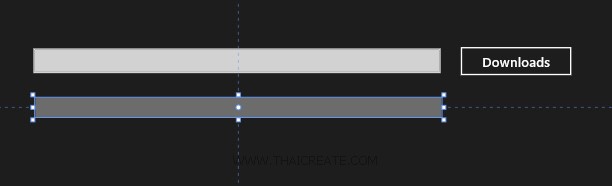
ออกแบบหน้าจอ Apps ดังนี้
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBox x:Name="txtURL" HorizontalAlignment="Left" Margin="105,114,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="608" FontSize="20" Height="37"/>
<Button x:Name="btnDownload" Content="Downloads" HorizontalAlignment="Left" Margin="740,109,0,0" VerticalAlignment="Top" FontSize="20" Click="btnDownload_Click" Height="48" Width="171"/>
<ProgressBar HorizontalAlignment="Center"
Height="30" Margin="108,187,652,551"
VerticalAlignment="Center"
Width="606" x:Name="DownloadProgress"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.Net.Http;
using Windows.Networking.BackgroundTransfer;
using Windows.Storage;
using System.Threading.Tasks;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private DownloadOperation activeDownload;
private async void btnDownload_Click(object sender, RoutedEventArgs e)
{
try
{
Uri source = new Uri(this.txtURL.Text);
string destination = "myZip.zip";
Windows.Storage.StorageFolder localFolder = Windows.Storage.ApplicationData.Current.LocalFolder;
StorageFile destinationFile = await localFolder.CreateFileAsync(destination, CreationCollisionOption.ReplaceExisting);
BackgroundDownloader downloader = new BackgroundDownloader();
DownloadOperation download = downloader.CreateDownload(source, destinationFile);
await HandleDownloadAsync(download);
}
catch (Exception ex)
{
//LogException("Download Error", ex);
}
}
private void ProgressCallback(DownloadOperation obj)
{
double progress
= ((double)obj.Progress.BytesReceived / obj.Progress.TotalBytesToReceive);
DownloadProgress.Value = progress * 100;
if (progress >= 1.0)
{
activeDownload = null;
}
}
private async Task HandleDownloadAsync(DownloadOperation downloadOperation)
{
activeDownload = downloadOperation;
var progress = new Progress<DownloadOperation>(ProgressCallback);
await downloadOperation.StartAsync().AsTask(progress);
}
}
}
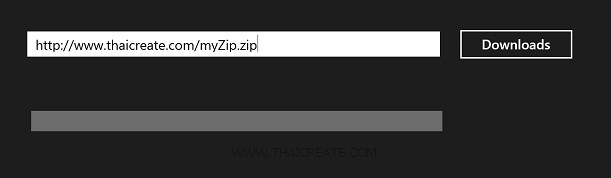
ทดสอบการทำงาน ให้กรอก URL สำหรับการ Download ไฟล์
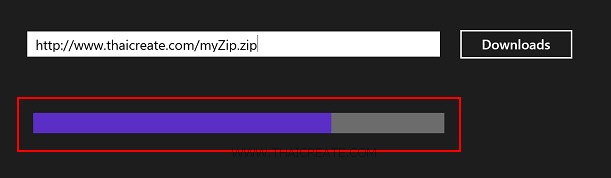
ในขณะที่ Download โปรแกรมจะแสดงสถานะและ ProgressBar สถานะการทำงาน
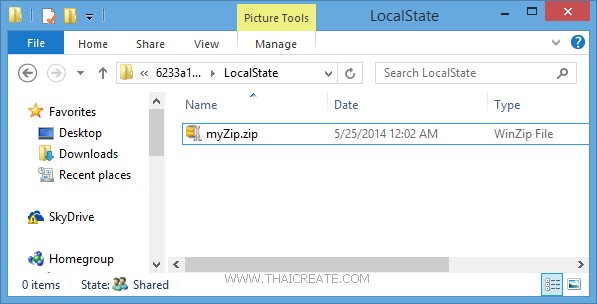
เมื่อ Download ครบ 100% ไฟล์จะถูกจดัเก็บลงใน Path ที่ได้กำหนดไว้ในขั้นต้น
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2014-05-26 16:08:48 /
2017-03-19 15:05:38 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|