Example 1 : Windows Store App and List Data (Web Services) - C# |
Example 1 : Windows Store App and List Data (Web Services) - C# สำหรับ Example : 1 จะเป็น Workshop ตัวอย่างการเขียน Windows Store Apps เพื่ออ่านข้อมูลจาก Web Services ซึ่งตัวอย่างนี้จะเป็นการประยุกต์และทำความเข้าใจการใช้งาน Web Services แบบง่าย ๆ หลังจากที่ได้อ่านในบทความ Web Services ก่อนหน้านี้เรียบร้อยแล้ว
Windows Store App and Web Services (C#)
Windows Store App and List Data (Web Services) - C#
สิ่งที่จะได้จากตัวอย่างนี้คือ
- การสร้าง Web Services การสร้าง JSON จาก MySQL
- การใช้ Windows Store Apps เรียกใช้งาน Web Services
- การนำ JSON มา Binding Data กับ ListView
ฝั่ง Web Services (ASP.Net/C#/MySQL)
MySQL Table บน Web Server

CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
เรียกใช้ NameSpace ไว้จัดการกับ MySQL Database
using System.Data;
using Newtonsoft.Json;
using MySql.Data.MySqlClient;
Method บน Web Services ซึ่งจะอ่านข้อมูลจาก MySQL แล้วส่งกลับเป็น JSON
[WebMethod]
public string getCustomerData()
{
MySqlConnection objConn = new MySqlConnection();
MySqlCommand objCmd = new MySqlCommand();
MySqlDataAdapter dtAdapter = new MySqlDataAdapter();
DataSet ds = new DataSet();
DataTable dt;
String strConnString, strSQL;
strConnString = "Server=localhost;User Id=root; Password=root; Database=mydatabase; Pooling=false";
strSQL = "SELECT * FROM customer";
objConn.ConnectionString = strConnString;
objCmd.Connection = objConn;
objCmd.CommandText = strSQL;
objCmd.CommandType = CommandType.Text;
dtAdapter.SelectCommand = objCmd;
dtAdapter.Fill(ds);
dt = ds.Tables[0];
dtAdapter = null;
objConn.Close();
objConn = null;
string json = JsonConvert.SerializeObject(dt, Formatting.Indented);
return json;
}
รูปแบบการอ่านค่า JSON ฝั่ง Windows Store Apps (C#)
var client = new myWebServices.myWSVSoapClient();
var result = await client.getCustomerDataAsync();
string jsonData = result.Body.getCustomerDataResult;
MemoryStream ms = new MemoryStream(Encoding.UTF8.GetBytes(jsonData));
ObservableCollection<myCustomer> list = new ObservableCollection<myCustomer>();
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(ObservableCollection<myCustomer>));
list = (ObservableCollection<myCustomer>)serializer.ReadObject(ms);
Example การเขียน Windows Store Apps เพื่ออ่านค่า จาก Web Services (C#)
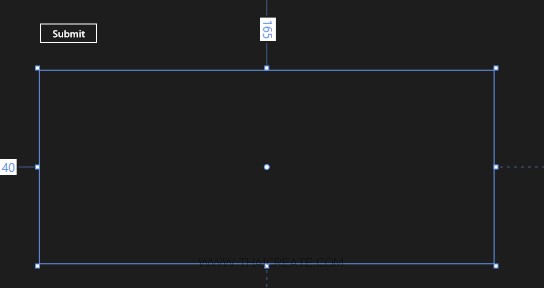
ออกแบบหน้าจอประกอบด้วย Button และ ListView
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Button x:Name="btnSubmit" Content="Submit" HorizontalAlignment="Left" Margin="137,68,0,0" VerticalAlignment="Top" FontSize="20" Width="120" Click="btnSubmit_Click"/>
<ListView x:Name="myListView" HorizontalAlignment="Left" Height="386" Margin="140,165,0,0" VerticalAlignment="Top" Width="907">
<ListView.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal" Margin="0,0,0,17">
<StackPanel Width="80">
<TextBlock Text="{Binding CustomerID}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0" FontFamily="Global User Interface"/>
</StackPanel>
<StackPanel Width="150">
<TextBlock Text="{Binding Name}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="300">
<TextBlock Text="{Binding Email}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="50">
<TextBlock Text="{Binding CountryCode}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Budget}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Used}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
</StackPanel>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using WindowsStoreApps.myWebServices;
using System.Xml.Linq;
using System.Runtime.Serialization;
using System.Runtime.Serialization.Json;
using System.Collections.ObjectModel;
using System.Text;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
public class myCustomer
{
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
private async void btnSubmit_Click(object sender, RoutedEventArgs e)
{
var client = new myWebServices.myWSVSoapClient();
var result = await client.getCustomerDataAsync();
string jsonData = result.Body.getCustomerDataResult;
MemoryStream ms = new MemoryStream(Encoding.UTF8.GetBytes(jsonData));
ObservableCollection<myCustomer> list = new ObservableCollection<myCustomer>();
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(ObservableCollection<myCustomer>));
list = (ObservableCollection<myCustomer>)serializer.ReadObject(ms);
this.myListView.ItemsSource = list;
}
}
}
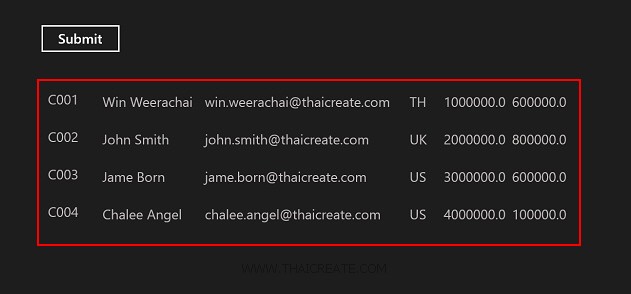
แสดงข้อมูลจาก Web Services บน Windows Store Apps
.
|