File Pickers กับ Save Dialog / Open Dialog บน Windows Store Apps (C#) |
File Pickers กับ Save Dialog / Open Dialog บน Windows Store Apps (C#) บทความนี้เราจะมาเรียนรู้การใช้งาน File Pickers ซึ่งการเขียน File Pickers บน Windows Store Apps จะมีรูปแบบที่เหมือนกับการเขียน Windows Form คือ สามารถที่จะ Browse เพื่อ Select เลือกไฟล์ และ Browse เพื่อที่จะ Save ไฟล์ ลงบน Windows 8 ได้ แต่รูปแบบ File และ Folder ที่อยู่บน Windows 8 อาจจะแตกต่างไปจาก Windows ก่อนหน้านี้พอสมควร เรามาลองดูตัวอย่างทั้ง 2 วิธี
Example 1 ทดสอบสร้าง File Pickers แบบเลือก Save ไฟล์บนเครื่อง Windows 8
FileSavePicker saver = new FileSavePicker();
saver.SuggestedStartLocation = PickerLocationId.Desktop;
saver.FileTypeChoices.Add("Text File", new List<string>() { ".txt" });
saver.SuggestedFileName = "text-name";
รูปแบบการเลือก Save ไฟล์ โดยสามารถกำหนด Location เป็น PickerLocationId.Desktop; (ไว้สำแหน่ง Default ที่ Desktop)
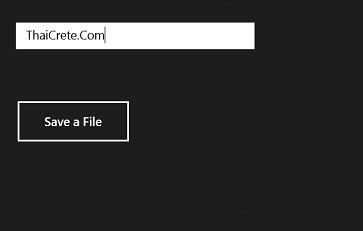
ในตัวอย่างนี้เราจะทดสอบรับค่าและเขียนลงใน Textfile และเลือก Save ไฟล์ในตำแหน่ง Location ที่ต้องการ
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Button x:Name="SaveFileDialog" Width="140" Height="54" Content="Save a File" Click="SaveAFile_Click" Margin="29,103,0,611" />
<TextBox x:Name="txtName" HorizontalAlignment="Left" Margin="30,55,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="288"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.Storage;
using Windows.Storage.AccessCache;
using Windows.Storage.Pickers;
using Windows.Storage.Provider;
using Windows.Storage.Streams;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
}
private async void SaveAFile_Click(object sender, RoutedEventArgs e)
{
FileSavePicker saver = new FileSavePicker();
saver.SuggestedStartLocation = PickerLocationId.Desktop;
saver.FileTypeChoices.Add("Text File", new List<string>() { ".txt" });
saver.SuggestedFileName = "text-name";
StorageFile file = await saver.PickSaveFileAsync();
if (file != null)
{
CachedFileManager.DeferUpdates(file);
await FileIO.WriteTextAsync(file, this.txtName.Text);
FileUpdateStatus status = await CachedFileManager.CompleteUpdatesAsync(file);
}
}
}
}
Screenshot
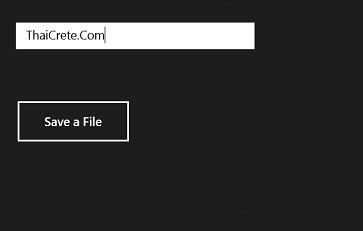
ทดสอบการกรอกข้อมูล และคลิกที่ Save a File
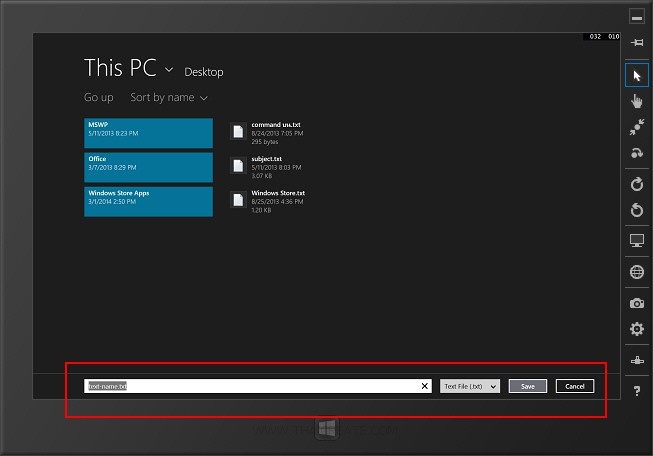
จากนั้นจะปรากฏ Dialog เพื่อให้เลือก Save ไฟล์ในตำแหน่ง Desktop
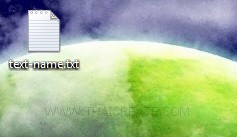
หลังจาก Save ไฟล์แล้ว ไฟล์จะถูกสร้างใน Desktop หรือ Location อื่น ๆ ที่เราได้เลือก Save ไว้
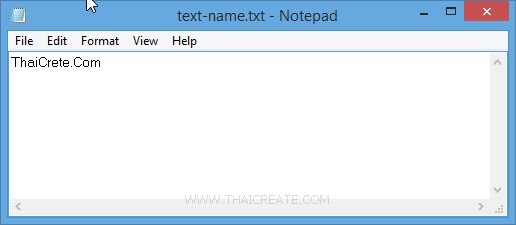
ทดสอบเปิดไฟล์
Example 2 ทดสอบ File Pickers แบบเลือก Browse ไฟล์และเรียกไฟล์นั้นมาใช้งาน
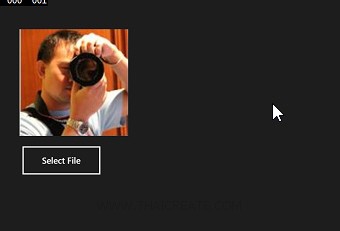
โดยในตัวอย่างนี้จะใช้ Image และ Button สำหรับ Browse ไฟล์รูปภาพ จากนั้นเรียกรูปภาพนั้นมาแสดงผล
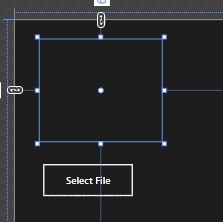
สร้าง Control ของ Image และ Button สำหรับแสดงผลรูปภาพ และการ Browse เลือกรูปภาพ
FileOpenPicker picker = new FileOpenPicker();
picker.FileTypeFilter.Add(".png");
picker.FileTypeFilter.Add(".jpg");
picker.FileTypeFilter.Add(".gif");
picker.FileTypeFilter.Add(".bmp");
picker.SuggestedStartLocation = PickerLocationId.Desktop;
picker.ViewMode = PickerViewMode.Thumbnail;
StorageFile file = await picker.PickSingleFileAsync();
if (file != null)
{
using (IRandomAccessStream fileStream = await file.OpenAsync(Windows.Storage.FileAccessMode.Read))
{
BitmapImage bitmapImage = new BitmapImage();
await bitmapImage.SetSourceAsync(fileStream);
this.imgView.Source = bitmapImage;
}
}
คำสั่งสำหรับ Browse เลือกไฟล์ และ เรียกใช้งาน Image มาแสดงบนหน้าจอ
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Image x:Name="imgView" Margin="37,29,1147,587"/>
<Button x:Name="SelectFile" Width="140" Height="54" Content="Select File" Click="SelectFile_Click" Margin="39,212,0,502" />
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.Storage;
using Windows.Storage.AccessCache;
using Windows.Storage.Pickers;
using Windows.Storage.Provider;
using Windows.Storage.Streams;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using Windows.UI.Xaml.Media.Imaging;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private async void SelectFile_Click(object sender, RoutedEventArgs e)
{
FileOpenPicker picker = new FileOpenPicker();
picker.FileTypeFilter.Add(".png");
picker.FileTypeFilter.Add(".jpg");
picker.FileTypeFilter.Add(".gif");
picker.FileTypeFilter.Add(".bmp");
picker.SuggestedStartLocation = PickerLocationId.Desktop;
picker.ViewMode = PickerViewMode.Thumbnail;
StorageFile file = await picker.PickSingleFileAsync();
if (file != null)
{
using (IRandomAccessStream fileStream = await file.OpenAsync(Windows.Storage.FileAccessMode.Read))
{
BitmapImage bitmapImage = new BitmapImage();
await bitmapImage.SetSourceAsync(fileStream);
this.imgView.Source = bitmapImage;
}
}
}
}
}
Screenshot
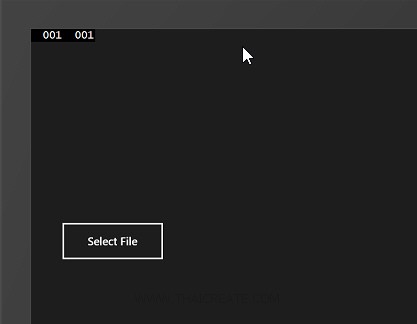
แสดงหน้าจอ Apps ให้คลิกที่ Select File เพื่อ Open Dialog
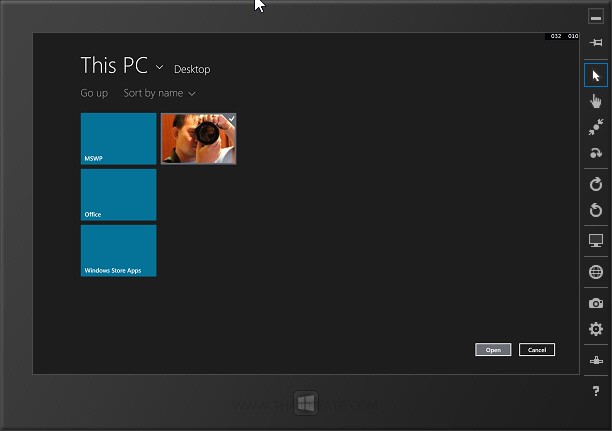
เลือกไฟล์ที่อยู่บน Desktop และเลือก Open
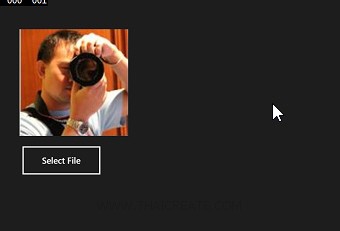
ไฟล์รูปภาพจะถุกนำมาแสดงผลบน Control ของ Image ในหน้าจอ Apps
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2014-03-02 10:56:43 /
2017-03-19 14:42:45 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|