Windows Store Apps and Play Media / Sound / Slider Control (C# ) |
Windows Store Apps and Play Media / Sound / Slider Control (C# ) ใบทความนี้จะเป็นเทคนิคการใช้ Control ของ MediaElement บน Windows Store Apps ซึ่งตัว Control นี้มีประโยชน์ไว้เล่นไฟล์ Media ต่าง ๆ ที่อยู่บน Windows Store Apps สามารถเล่นไฟล์ Media ได้ทั้งที่เป็นแบบ Video Clip หรือ Sound รองรับไฟล์หลาย ๆ Format เช่น .wma , .wmv , .flv , .mp3 และอื่น ๆ อีกมากมาย
และในการเล่นไฟล์ Media สิ่งหนึ่งที่เราจะต้องจำเป็นจะรู้ คือ สถานะและตำแหน่งของไฟล์ Media ซึ่งจะบ่งบอกถึงความความยาว และ สถานะของการเล่นไฟล์ว่าตอนนี้เล่นถึงตำแหน่งไหน ที่เท่าไหร่ และอีกเมื่อไหร่ถึงจะจบไฟล์ และที่สำคัญอีกอย่างหหนึ่งคือการเลื่อนไป เลื่อนมา เพื่อเล่นไฟล์ในตำแหน่งต่าง ๆ ที่ต้องการ และเครื่องมือที่จะใช้ควบคุมสถานะนี้บน Windows Store Apps เราจะใช้ Control ที่มีชื่อว่า Slider เป็น Control ที่ใช้งานง่าย ๆ แต่สามารถประยุกต์การใช้งานได้หลากหลาย
MediaElement Format Supported
http://msdn.microsoft.com/en-us/library/cc189080(vs.95).aspx
และความสามารถของ MediaElement คือการเก็บ State ของ Media ลงใน Memory ได้ ฉะนั้นในกรณีที่เล่นไปแล้ว จะไม่ทำการ Download ในส่วนนั้นอีก ฉะนั้นเราสามารถทำปุ่ม PAUSE , STOP และ PLAY ในกรณีที่ต้องการหยุด หรือ เล่นไฟล์นั้น ๆ ได้ต่ออีก
MediaElement State Diagram
ขั้นตอนการสร้าง MediaElement กับ Slider
สร้าง Control MediaElement/Slider พร้อมกับกำหนด Method ของ ValueChanged
<MediaElement x:Name="videosource" />
<Slider x:Name="seek" HorizontalAlignment="Left" ValueChanged="seek_ValueChanged"/>
จุดสำคัญจะอยู่ที่ MediaOpened ซึ่งหมายถึงเมื่อ Media มีการเล่นไฟล์ จะสร้าง Timer เพื่อตรวจสอบ ตำแหน่งของ Media และ Update Slider
private void videosource_MediaOpened(object sender, RoutedEventArgs e)
{
seek.Maximum = videosource.NaturalDuration.TimeSpan.TotalMilliseconds;
ticks.Interval = TimeSpan.FromMilliseconds(1);
ticks.Tick += ticks_Tick;
ticks.Start();
}
การ Update ตำแหน่งและสถานะไปยัง Slider
void ticks_Tick(object sender, object e)
{
seek.Value = videosource.Position.TotalMilliseconds;
}
เมื่อมีการเลื่อน Slider โดยผู้ใช้ ก็ให้เลือนตำแหน่งที่เล่นไปยัง ตำแหน่งที่เลือก
private void seek_ValueChanged(object sender, RangeBaseValueChangedEventArgs e)
{
int slidervalue = (int)seek.Value;
TimeSpan ts = new TimeSpan(0, 0, 0, 0, slidervalue);
videosource.Position = ts;
}
ลองมาดู Code แบบเต็ม ๆ
Example ตัวอย่างการเล่นไฟล์ Media และควบคุมตำแหน่งด้วย Slider ด้วยการ Browse เลือกไฟล์จาก Desktop
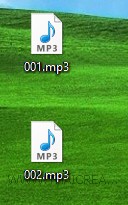
เรามีไฟล์ MP3 อยู่บน Desktop ของ Windows 8
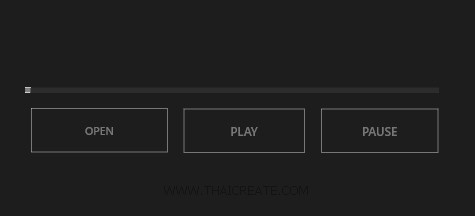
ออกแบบหน้าจอประกอบด้วย MediaElement , Slider และ Button สำหรับปุ่ม OPEN , PLAY และ PAUSE
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<MediaElement x:Name="videosource" HorizontalAlignment="Center" Height="910" Margin="-23.913,-26.583,-188.087,-116.417" VerticalAlignment="Bottom" Width="1580" RenderTransformOrigin="0.5,0.5" UseLayoutRounding="False" d:LayoutRounding="Auto" MediaOpened="videosource_MediaOpened">
<MediaElement.RenderTransform>
<CompositeTransform Rotation="-0.185"/>
</MediaElement.RenderTransform>
</MediaElement>
<Button x:Name="open" Content="OPEN" HorizontalAlignment="Left" Height="95" Margin="255,311,0,0" VerticalAlignment="Top" Width="280" Opacity="0.4" Click="open_Click" FontSize="22"/>
<Button x:Name="Play" Content="PLAY" HorizontalAlignment="Left" Height="95" Margin="560,312,0,0" VerticalAlignment="Top" Width="249" Opacity="0.4" Click="Play_Click" FontSize="24"/>
<Button x:Name="pause" Content="PAUSE" HorizontalAlignment="Left" Height="95" Margin="835,312,0,0" VerticalAlignment="Top" Width="241" Opacity="0.4" Click="pause_Click" FontSize="24"/>
<Slider x:Name="seek" HorizontalAlignment="Left" Height="65" Margin="246,256,0,0" VerticalAlignment="Top" Width="828" Opacity="0.4" SmallChange="0.1" StepFrequency="0.01" LargeChange="10" ValueChanged="seek_ValueChanged"/>
</Grid>
</Page>
MainPage.xam.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using Windows.Media.PlayTo;
using Windows.Storage;
using Windows.Storage.Pickers;
using Windows.Storage.Streams;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
PlayToManager playToManager = null;
CoreDispatcher dispatcher = null;
DispatcherTimer ticks;
public MainPage()
{
this.InitializeComponent();
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
dispatcher = Window.Current.CoreWindow.Dispatcher;
playToManager = PlayToManager.GetForCurrentView();
playToManager.SourceRequested += playToManager_SourceRequested;
}
private void playToManager_SourceRequested(PlayToManager sender, PlayToSourceRequestedEventArgs args)
{
var deferral = args.SourceRequest.GetDeferral();
var handler = dispatcher.RunAsync(CoreDispatcherPriority.Normal, () =>
{
args.SourceRequest.SetSource(videosource.PlayToSource);
deferral.Complete();
});
}
async private void open_Click(object sender, RoutedEventArgs e)
{
seek.Value = 0;
FileOpenPicker filepicker = new FileOpenPicker();
filepicker.SuggestedStartLocation = PickerLocationId.VideosLibrary;
filepicker.FileTypeFilter.Add(".mp4");
filepicker.FileTypeFilter.Add(".wmv");
filepicker.FileTypeFilter.Add(".mp3");
filepicker.FileTypeFilter.Add(".flv");
filepicker.ViewMode = PickerViewMode.Thumbnail;
StorageFile localVideo = await filepicker.PickSingleFileAsync();
if (localVideo != null)
{
var stream = await localVideo.OpenAsync(FileAccessMode.Read);
videosource.SetSource(stream, localVideo.ContentType);
}
}
private void videosource_MediaOpened(object sender, RoutedEventArgs e)
{
seek.Maximum = videosource.NaturalDuration.TimeSpan.TotalMilliseconds;
ticks.Interval = TimeSpan.FromMilliseconds(1);
ticks.Tick += ticks_Tick;
ticks.Start();
}
void ticks_Tick(object sender, object e)
{
seek.Value = videosource.Position.TotalMilliseconds;
}
private void Play_Click(object sender, RoutedEventArgs e)
{
videosource.Play();
}
private void pause_Click(object sender, RoutedEventArgs e)
{
videosource.Pause();
}
private void seek_ValueChanged(object sender, RangeBaseValueChangedEventArgs e)
{
int slidervalue = (int)seek.Value;
TimeSpan ts = new TimeSpan(0, 0, 0, 0, slidervalue);
videosource.Position = ts;
}
}
}
Result
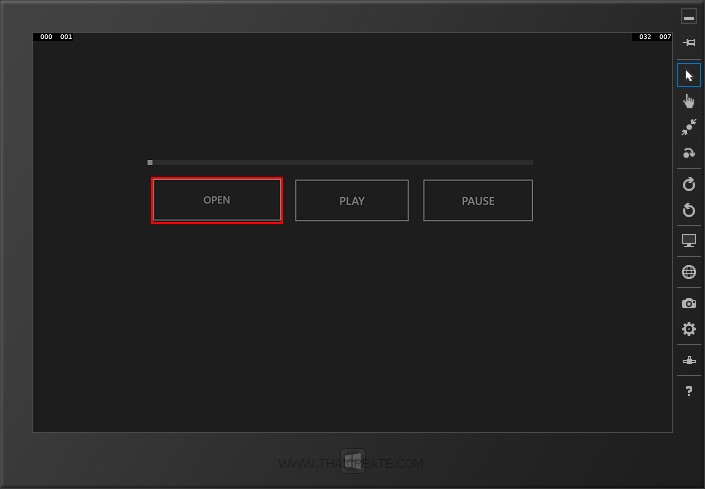
แสดงหน้าจอของ Apps ให้คลิก Open เพื่อเลือกไฟล์ Media
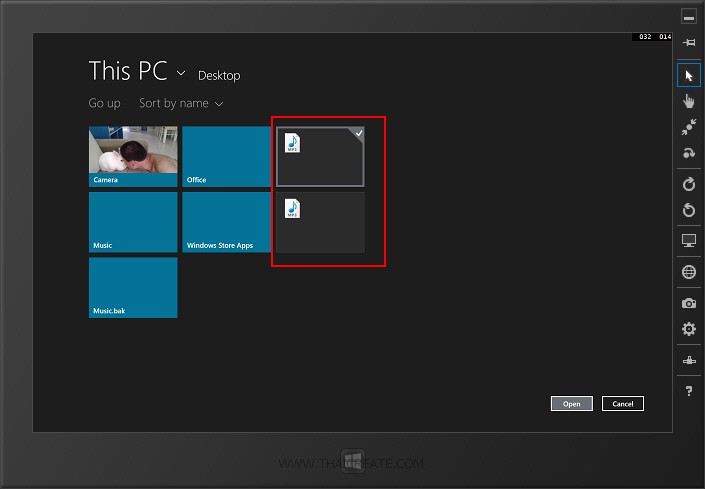
เลือกไฟล์ Media ที่ซึ่งในนี้จะเลือกเล่นไฟล์ MP3 ที่อยู่บน Desktop
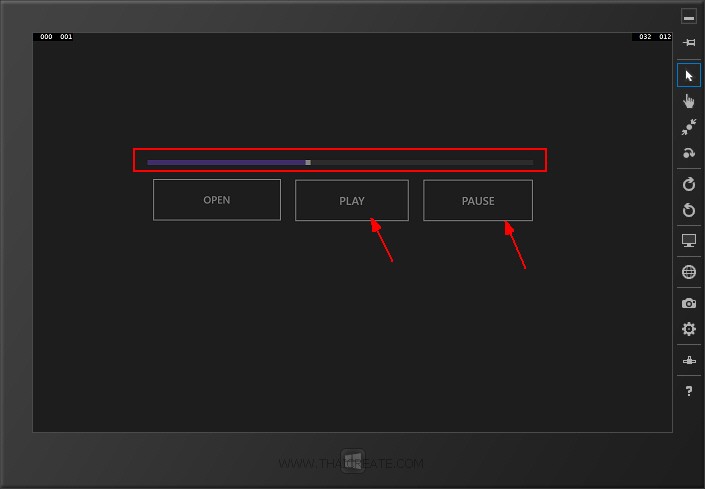
โปรแกรมกำลังเล่นไฟล์บน MediaElement พร้อมกับ Slider เป็นตัวควบคุมสำแหน่งของการเล่น
.
Reference : http://code.msdn.microsoft.com/windowsapps/Media-Player-Sample-App-f9c72fb8
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2014-06-23 13:18:38 /
2017-03-19 15:01:41 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|