Windows Store Apps การส่ง ตัวแปร หรือ Parameters ข้าม Page (C#) |
Windows Store Apps การส่ง ตัวแปร หรือ Parameters ข้าม Page (C#) การส่งค่า Parameters ระหว่าง Page บน Windows Store Apps จะใช้ในกรณีที่ต้องการส่งค่าตัวแปรหรือ Parameters ที่เกิดขึ้นใน Page ปัจจุบันไปยัง Page อื่น ๆ ซึ่งหลักการเดียวกับการค่าในรูปแบบของ QueryString คือส่งไปกับ Event ของ Frame.Navigate และ Page ปลายทางที่ทำหน้าที่รับค่าก็จะใช้ผ่าน method ของ OnNavigatedTo ด้วยการอ่านค่า Parameters ที่ถูกส่งมา โดยค่าที่ส่งไปนั้นสามารถส่งได้ทั้งค่าที่เป็น String ธรรมดา หรือจะเป็น Array , List หรือ Object ของ Class
ส่ง Parameters
this.Frame.Navigate(typeof(Page2),this.txtName.Text);
รับ Parameters
protected override void OnNavigatedTo(NavigationEventArgs e)
{
string name = e.Parameter as string;
if (!string.IsNullOrWhiteSpace(name))
{
this.lblResult.Text = "Hello, " + name;
}
}
Windows Store Apps การส่ง ตัวแปร หรือ Parameters ข้าม Page
กลับมายัง Project ของ Windows Store Apps บน Visual Studio
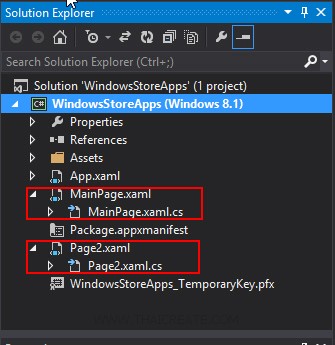
ตอนนี้เรามี Page ของ Apps อยู่ 2 Page คือ MainPage.xaml และ Page2.xaml โดยออกแบบ Layout และเขียน Event ในการส่งค่าดังนี้
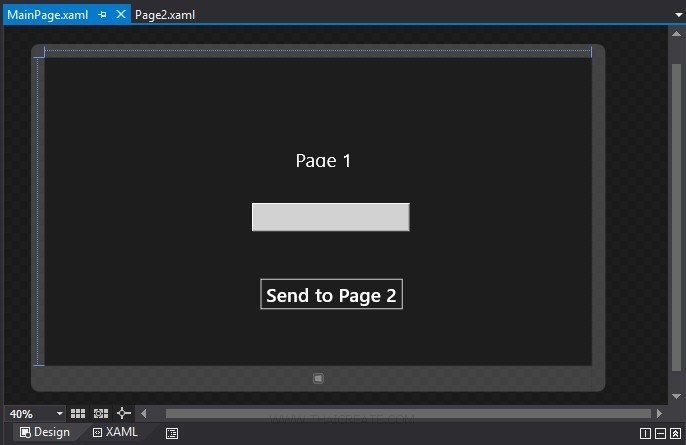
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblTitle" HorizontalAlignment="Center" Margin="626,229,431,0" TextWrapping="Wrap" Text="Page 1" VerticalAlignment="Top" Height="43" Width="309" FontSize="48"/>
<TextBox x:Name="txtName" HorizontalAlignment="Left" Height="70" Margin="518,363,0,0" TextWrapping="Wrap" Text="" VerticalAlignment="Top" Width="393" FontSize="48"/>
<Button x:Name="btnSend" Content="Send to Page 2" HorizontalAlignment="Left" Margin="536,549,0,0" VerticalAlignment="Top" FontSize="48" Click="btnSend_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private void btnSend_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2),this.txtName.Text);
}
}
}
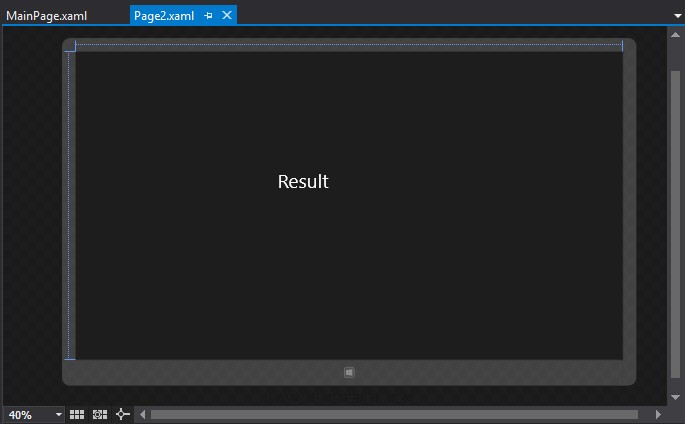
Page2.xaml
<Page
x:Class="WindowsStoreApps.Page2"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblResult" HorizontalAlignment="Center" Margin="504,296,393,0" TextWrapping="Wrap" Text="Result" VerticalAlignment="Top" Height="86" Width="469" FontSize="48"/>
</Grid>
</Page>
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
string name = e.Parameter as string;
if (!string.IsNullOrWhiteSpace(name))
{
this.lblResult.Text = "Hello, " + name;
}
else
{
this.lblResult.Text = "Name is required. Go back and enter a name.";
}
}
}
}
Screenshot
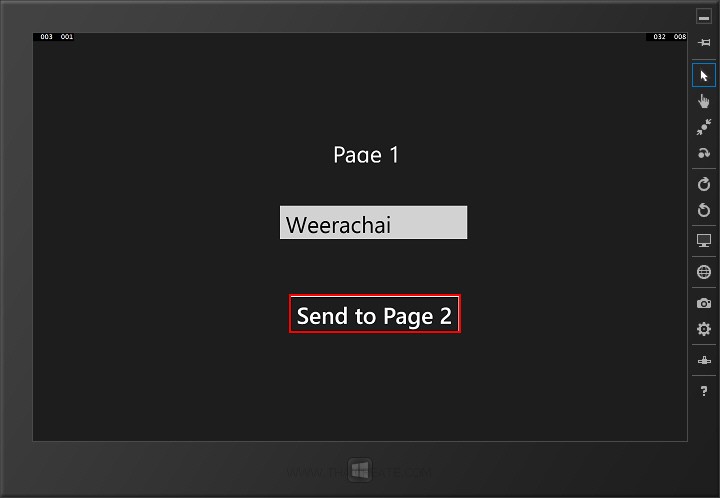
ทดสอบ Input ข้อมูลใน TextBox และคลิกที่ Button เพื่อส่งไปยัง Page2
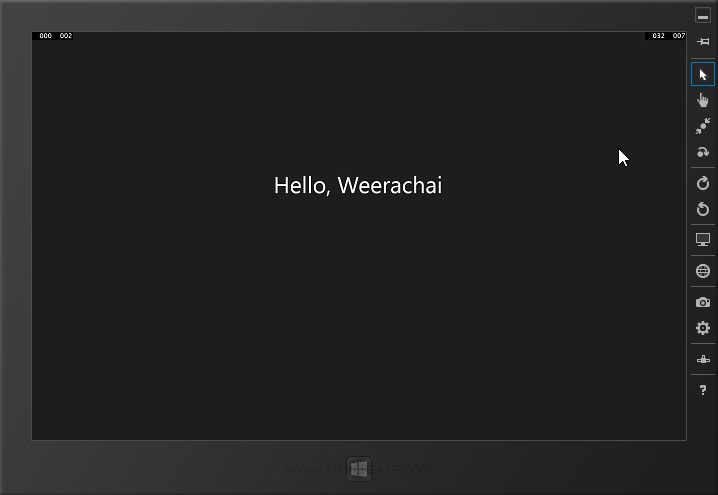
Page2 ทำการรับค่าและแสดงค่า Parameters ออกทางหน่าจอของ Apps
นอกจากนี้ค่า Pagemeters ยังสามารถส่งค่าเป็นแบบ Object ในรุปแบบของ Class ได้เช่นเดียวกัน
public class Member
{
public string ID { get; set; }
public string User { get; set; }
}
private void btnSend_Click(object sender, RoutedEventArgs e)
{
Member member = new Member();
member.ID = "1";
member.User = "Win";
this.Frame.Navigate(typeof(Page2), member);
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
Member member = e.Parameter as Member;
this.lblResult.Text = String.Format("ID={0} User={1}",
member.ID,
member.User);
}
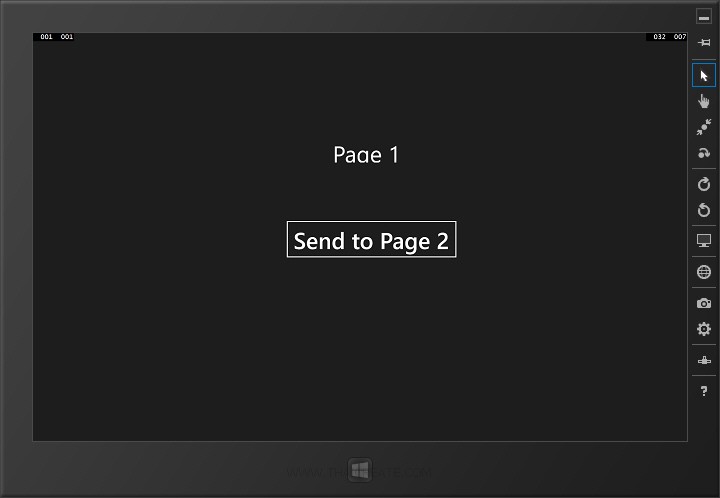
ทดสอบการทำงาน
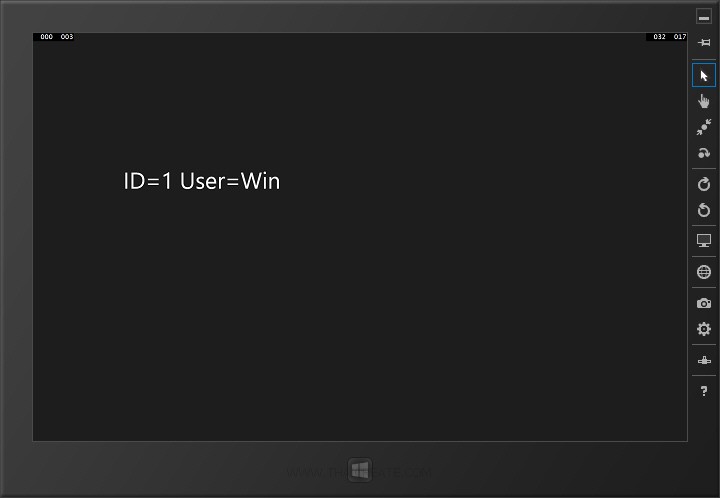
ทดสอบการทำงาน
หรือจะส่งค่าแบบ List ในรูปแบบ Array กับ Object
private void btnSend_Click(object sender, RoutedEventArgs e)
{
var myList = new List<string>()
{
"Bat",
"Rat",
"Cat",
};
this.Frame.Navigate(typeof(Page2), myList);
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
var myList = e.Parameter as List<string>;
this.lblResult.Text = "";
foreach (string val in myList)
{
this.lblResult.Text = this.lblResult.Text + " " + val;
}
}
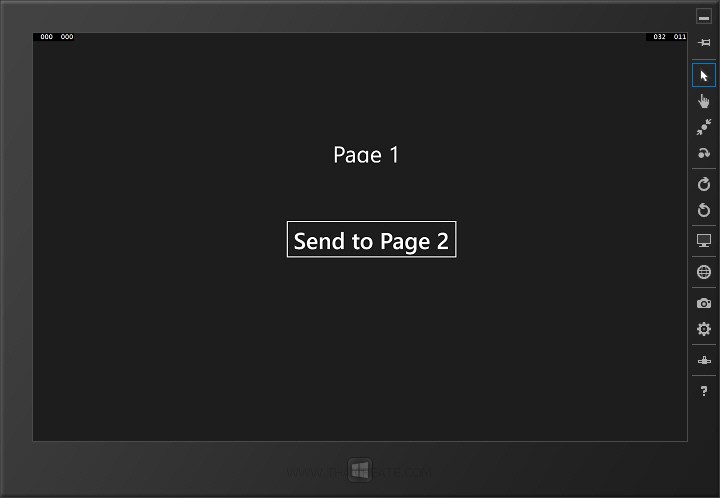
ทดสอบการทำงาน
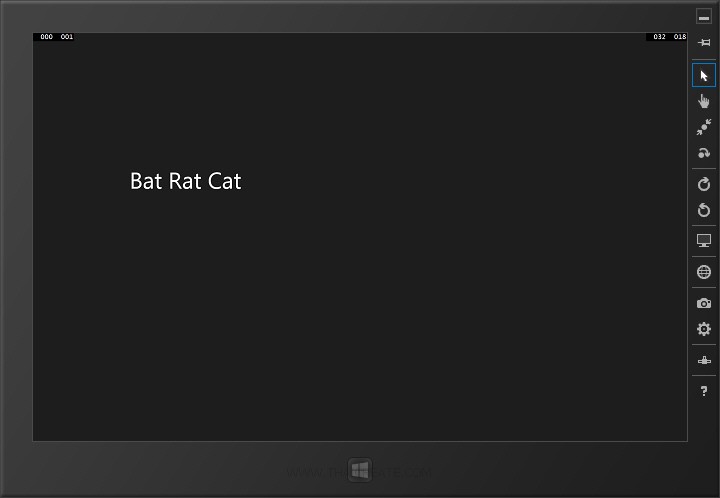
ทดสอบการทำงาน
จากบทความนี้เราะจะเห็นว่าการส่งค่า Parameters ระหว่าง Page บน Windows Store Apps นั้น สามารถส่งได้ทั้งในรูปแบบของ String และก็ชุดข้อมูลที่อยู่ในรูปแบบของ Array , Object หรือ Class และในบทความนี้ก็เป็นเพียงตัวอย่างที่ง่าย ๆ และสั้น ๆ แต่สามารถนำไปประยุกต์ใช้งานกับการเขียนโปรแกรมที่หลากหลายและซับซ้อนยิ่งขึ้น
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
|
|
|
Create/Update Date : |
2014-01-29 20:30:11 /
2017-03-19 14:33:51 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|