Windows Store App and PDF Viewer (C#) |
Windows Store App and PDF Viewer (C#) บน App ของ Windows Store เราอาจจะเคยเห็นพวก App ที่ใช้สำหรับอ่าน Ebook หรือ Cartoon โดยรูปแบบการอ่านจะสามารถย่อ-ขยาย หรือเลื่อนไปยังหน้าต่าง ๆ ของ Ebook ได้ ในปัจจุบันการอ่าน Ebook บนอุปกรณ์ Tablets ก็ได้รับความนิยมมาก และในอนาคตก็อาจจะมาทดแทนหนังสือทั่ว ๆ ไปอย่างแน่นอน เพราะลดตนทุน มีความสะดวกและง่ายในการอ่าน ส่วนรูปแบบไฟล์นั้น Ebook ต่าง ๆ เหล่านี้ก็ก็ล้วนอยู่ในรูป PDF ไฟล์ โดยไฟล์เหล่านี้เราสามารถ Download จากแหล่งต่าง ๆ มาจัดเก็ยไว้ในเครื่อง จากนั้นก็ใช้ Apps ที่เรามีอยู่นั้นทำการเปิดไฟล์ PDF เหล่านั้น
ในตัวอย่างนี้จะใช้รูปแบบการแปลงไฟล์ PDF ให้เป็นรูปภาพ โดยจะใช้การอ่านจำนวนหน้าของไฟล์ PDF จากนั้นแปลงเป็น Image แล้วค่อยแสดงผลบน Control ของ FlipView ซึ่งจะทำให้เราสามารถที่จะการ Slide เลื่อนไปยังหน้าต่าง ๆ ได้
รูปแบบการอ่าน PDF ไฟล์บน Windows Store Apps (C#)
บน XAML สร้าง FlipView ซึ่งเป็น Control สำหรับแสดงข้อมูลแบบ Flip (Slide)
<FlipView x:Name="myFlipView">
<FlipView.ItemTemplate>
<DataTemplate>
<Grid>
<Image Source="{Binding Image}" HorizontalAlignment="Center" Height="{Binding Height}" Width="{Binding Width}"></Image>
</Grid>
</DataTemplate>
</FlipView.ItemTemplate>
<FlipView.ItemsPanel>
<ItemsPanelTemplate>
<VirtualizingStackPanel AreScrollSnapPointsRegular="True" Orientation="Horizontal" CleanUpVirtualizedItemEvent="VirtualizingStackPanel_CleanUpVirtualizedItemEvent" />
</ItemsPanelTemplate>
</FlipView.ItemsPanel>
</FlipView>
เรียกใช้งาน Class Library ของ Windows.Data.Pdf
using Windows.Data.Pdf;
สร้าง File Picker สำหรับ Browse เพื่อเลือกไฟล์
FileOpenPicker openPicker = new FileOpenPicker();
openPicker.ViewMode = PickerViewMode.List;
openPicker.SuggestedStartLocation = PickerLocationId.DocumentsLibrary;
openPicker.FileTypeFilter.Add(".pdf");
StorageFile pdfFile = await openPicker.PickSingleFileAsync();
การอ่านไฟล์ PDF จำนวนหน้าทั้งหมด และแสดงผลบน Flip View
var pdfDocument = await PdfDocument.LoadFromFileAsync(pdfFile);
for (int i = 0; i < pdfDocument.PageCount; i++)
{
items.Add(new FData() { PageNumber = i, PdfDoc = pdfDocument, Width = Window.Current.Bounds.Width, Height = Window.Current.Bounds.Height });
}
myFlipView.ItemsSource = items;
ลองมาดูตัวอย่างแบบเต็ม ๆ
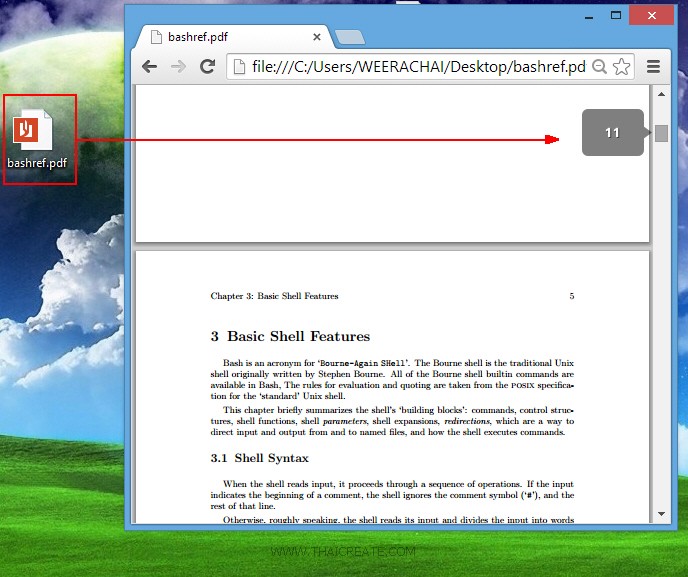
ไฟล์ PDF ที่อยู่บน Desktop ซึ่งประกอบด้วยหลาย ๆ หน้า

Example การเขียน Windows Store Apps อ่าน PDF ไฟล์ มาแสดงบนหน้าจอ ของ Windows Store Apps
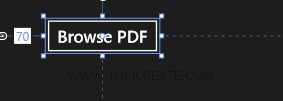
ออกแบบหน้าจอดังรูป ซึ่งประกอบด้วย Button และ FlipView
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<FlipView x:Name="myFlipView">
<FlipView.ItemTemplate>
<DataTemplate>
<Grid>
<Image Source="{Binding Image}" HorizontalAlignment="Center" Height="{Binding Height}" Width="{Binding Width}"></Image>
</Grid>
</DataTemplate>
</FlipView.ItemTemplate>
<FlipView.ItemsPanel>
<ItemsPanelTemplate>
<VirtualizingStackPanel AreScrollSnapPointsRegular="True" Orientation="Horizontal" CleanUpVirtualizedItemEvent="VirtualizingStackPanel_CleanUpVirtualizedItemEvent" />
</ItemsPanelTemplate>
</FlipView.ItemsPanel>
</FlipView>
<Button x:Name="btnBrowse" Content="Browse PDF" HorizontalAlignment="Left" Margin="70,38,0,0" VerticalAlignment="Top" FontSize="25" Click="btnBrowse_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using Windows.Storage;
using Windows.Storage.AccessCache;
using Windows.Storage.Pickers;
using Windows.Storage.Provider;
using Windows.Storage.Streams;
using Windows.Data.Pdf;
using System.ComponentModel;
using Windows.ApplicationModel;
using System.Collections.ObjectModel;
using Windows.UI.Xaml.Media.Imaging;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
ObservableCollection<FData> items = new ObservableCollection<FData>();
private void VirtualizingStackPanel_CleanUpVirtualizedItemEvent(object sender, CleanUpVirtualizedItemEventArgs e)
{
var item = e.Value as FData;
item.Dispose();
}
private async void btnBrowse_Click(object sender, RoutedEventArgs e)
{
FileOpenPicker openPicker = new FileOpenPicker();
openPicker.ViewMode = PickerViewMode.List;
openPicker.SuggestedStartLocation = PickerLocationId.DocumentsLibrary;
openPicker.FileTypeFilter.Add(".pdf");
StorageFile pdfFile = await openPicker.PickSingleFileAsync();
if(pdfFile != null)
{
var pdfDocument = await PdfDocument.LoadFromFileAsync(pdfFile);
for (int i = 0; i < pdfDocument.PageCount; i++)
{
items.Add(new FData() { PageNumber = i, PdfDoc = pdfDocument, Width = Window.Current.Bounds.Width, Height = Window.Current.Bounds.Height });
}
myFlipView.ItemsSource = items;
}
}
}
public class FData : INotifyPropertyChanged
{
public int PageNumber { get; set; }
public PdfDocument PdfDoc { get; set; }
public double Height { get; set; }
public double Width { get; set; }
private ImageSource _image = null;
public ImageSource Image
{
get
{
if (_image == null)
Rendering();
return _image;
}
set
{
_image = value;
}
}
private async void Rendering()
{
using (var page = PdfDoc.GetPage((uint)PageNumber))
{
var renderOption = new PdfPageRenderOptions();
BitmapImage img = new BitmapImage();
renderOption.DestinationHeight = (uint)(Height);
InMemoryRandomAccessStream stream = new InMemoryRandomAccessStream();
await page.RenderToStreamAsync(stream, renderOption);
img.SetSource(stream);
_image = img;
if (PropertyChanged != null)
PropertyChanged(this, new PropertyChangedEventArgs("Image"));
_image = null;
}
}
public event PropertyChangedEventHandler PropertyChanged;
internal void Dispose()
{
_image = null;
}
}
}
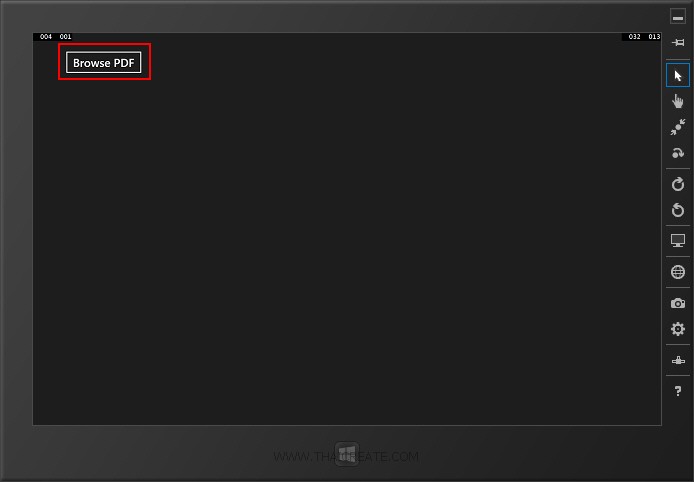
คลิกที่ปุ่ม Browse แสดง File Picker เพื่อเลือกไฟล์ PDF
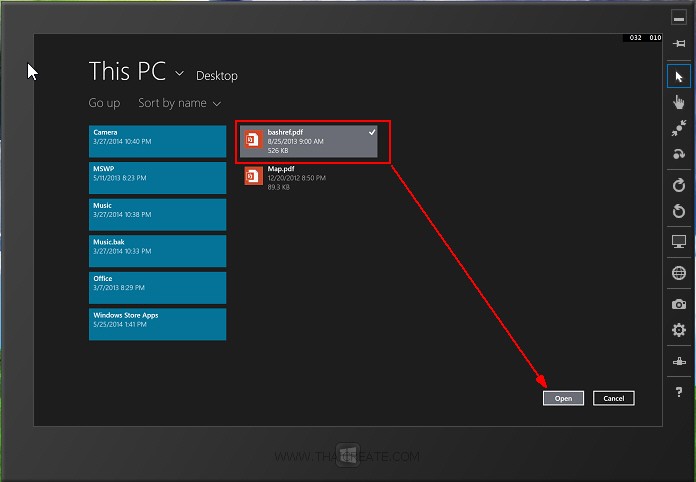
เลือกไฟล์ PDF ที่อยู่บน Desktop
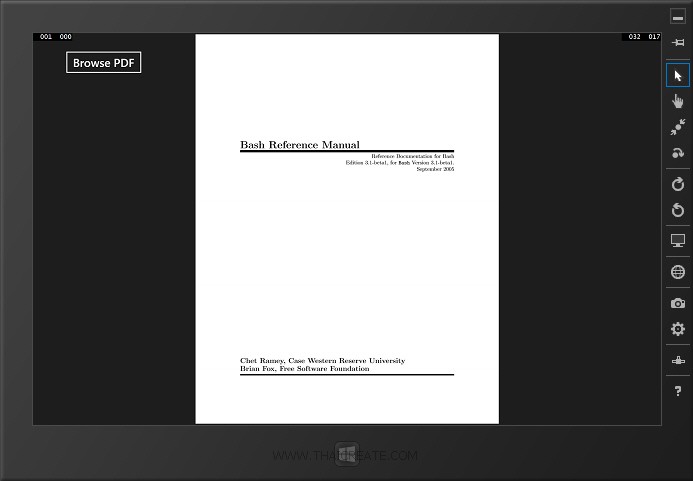
จากนั้นโปรแกรมจะแสดง PDF ไฟล์
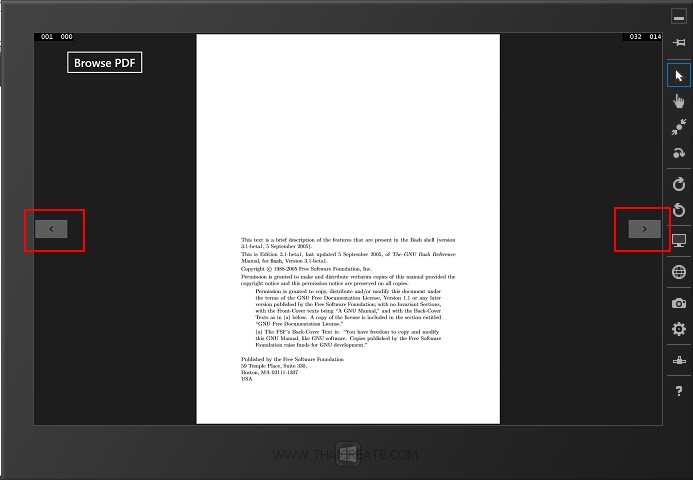
กรณีที่ PDF มีมากกว่า 1 หน้า สามารถเลื่อนเลื่อนไปดูหน้าถัด ๆ ไปได้ หรือจะย้อนกลับ
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2014-05-27 11:56:25 /
2017-03-19 15:13:06 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|