Context Menus and Popup Menu บน Windows Store Apps (C#) |
Context Menus and Popup Menu บน Windows Store Apps (C#) สำหรับ Context Menu หรือ Popup Menu เป็นกล้องเมนูสี่เหลี่ยมเล็ก ๆ คล้าย ๆ กับการคลิกขวาบนหน้าจอ Windows OS ทั่ว ๆ ไป โดยเหมาะสำหรับทำเป็น Function พิเศษของเหตุการณ์ใดเหตุการณ์หนึ่ง เช่น เราอาจจะคุ้นเคยเมื่อคลิกขวาที่ไฟล์ แล้วมี Menu ว่า Copy , Cut หรือ Delete เป็นต้น แต่บน Windows Store Apps เราสามารถนำมาประยุกต์ใช้เป็นพวก Menu ของ Apps ไว้เลือกรายการ หรือเป็นคำสั่งพิเศษสำหรับการทำงานต่าง ๆ
รูปแบบการสร้าง Context Menu
PopupMenu menu = new PopupMenu();
menu.Commands.Add(new UICommand
{
Id = 1,
Label = "Menu 1",
Invoked = DoTasks
});
menu.Commands.Add(new UICommand
{
Id = 2,
Label = "Menu 2",
Invoked = DoTasks
});
var pointTransform = ((Button)sender).TransformToVisual(Window.Current.Content);
var screenCoords = pointTransform.TransformPoint(new Point(210, 100));
await menu.ShowAsync(screenCoords);
รูปแบบการอ่าน Event ที่เกิดจากการคลิก Context Menu
var currentId = (int)command.Id;
MessageDialog msgDialog;
switch (currentId)
{
case 1:
msgDialog = new MessageDialog( "Menu 1","Your selected");
msgDialog.ShowAsync();
break;
case 2:
msgDialog = new MessageDialog("Menu 2", "Your selected");
msgDialog.ShowAsync();
break;
default :
break;
}
มาดูตัวอย่างกันแบบง่าย ๆ
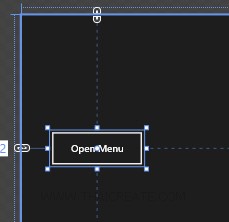
สร้างปุ่ม Button แบบง่าย ๆ เพื่อที่จะเรียก Context หรือ Popup Menu
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Button x:Name="OpenPopupMenu" Width="140" Height="54" Content="Open Menu" Click="OpenPopupMenu_Click" Margin="42,172,0,542" />
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Popups;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private async void OpenPopupMenu_Click(object sender, RoutedEventArgs e)
{
PopupMenu menu = new PopupMenu();
menu.Commands.Add(new UICommand
{
Id = 1,
Label = "Menu 1",
Invoked = DoTasks
});
menu.Commands.Add(new UICommand
{
Id = 2,
Label = "Menu 2",
Invoked = DoTasks
});
menu.Commands.Add(new UICommand
{
Id = 3,
Label = "Menu 3",
Invoked = DoTasks
});
menu.Commands.Add(new UICommand
{
Id = 4,
Label = "Menu 4",
Invoked = DoTasks
});
menu.Commands.Add(new UICommand
{
Id = 5,
Label = "Menu 5",
Invoked = DoTasks
});
var pointTransform = ((Button)sender).TransformToVisual(Window.Current.Content);
var screenCoords = pointTransform.TransformPoint(new Point(210, 100));
await menu.ShowAsync(screenCoords);
}
private void DoTasks(IUICommand command)
{
var currentId = (int)command.Id;
MessageDialog msgDialog;
switch (currentId)
{
case 1:
msgDialog = new MessageDialog( "Menu 1","Your selected");
msgDialog.ShowAsync();
break;
case 2:
msgDialog = new MessageDialog("Menu 2", "Your selected");
msgDialog.ShowAsync();
break;
case 3:
msgDialog = new MessageDialog("Menu 3", "Your selected");
msgDialog.ShowAsync();
break;
case 4:
msgDialog = new MessageDialog("Menu 4", "Your selected");
msgDialog.ShowAsync();
break;
case 5:
msgDialog = new MessageDialog("Menu 5","Your selected");
msgDialog.ShowAsync();
break;
default :
break;
}
}
}
}
Screenshot
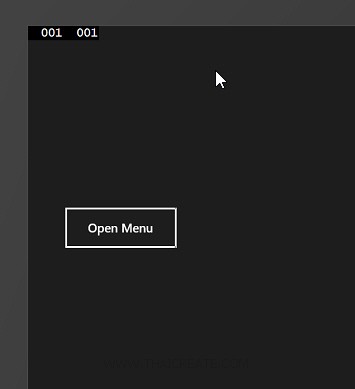
แสดงหน้าจอ Apps ให้ทดสอบที่คลิก Button เพื่อเรียก Context Menu หรือ Popup Menu
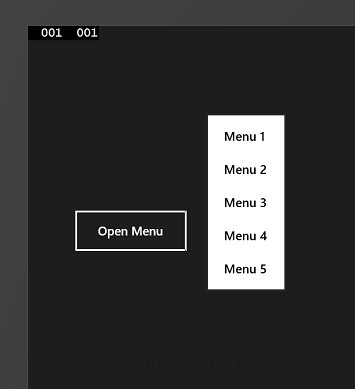
แสดง Context Menu หรือ Popup Menu
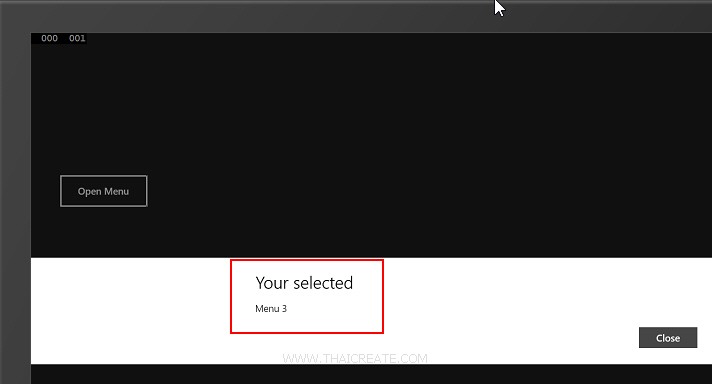
แสดงเหตุการณ์เมื่อมีการคลิกเลือกที่ Menu ย่อย
.
|