Windows Store Apps and Send Mail Using SMTP Server (C#) |
Windows Store Apps and Send Mail Using SMTP Server (C#) สำหรับการส่งอีเมล์บน Windows Store Apps ด้วย C# อาจจะมีความยุ่งยากพอควร เพราะจะไม่มี Class ของ System.Net ให้ใช้เหมือนกัน .Net Application อื่น ๆ ซึ่งถ้าจะต้องการส่งอีเมล์ผ่าน SMTP บน Windows Store Apps อาจจะต้องลงหา Library ที่รองรับทำงานบน WinRT และตอนนี้ที่ใช้งานได้ฟรีก็ห็นมีอยู่ไม่กี่ตัว เช่น HigLabo โดย Library นี้สามารถ Download ใช้งานได้ฟรี ทั้งการติดตั้งจาก dll หรือจะผ่าน Manage NuGet Packages
Windows Store Apps และการส่งอีเมล์ด้วย SMTP (C#)
ก่อนการใช้งานเราจะต้องมี Account ที่สามารถ Authen ผ่าน SMTP ทำการ Logon ด้วย User/Password แล้วค่อยทำการเขียนเพื่อส่งอีเมล์ไปยัง Account ปลายทาง
Download HigLabo Library for WinRT
http://higlabo.codeplex.com/
ให้เลือก Version ที่เป็น WinRT โดยจะประกอบด้วย
- Net (Http OAuth Socket)
- Mail (Smtp POP3 IMAP)
- Twitter, Facebook, Rss, Dropbox, SugarSync, Box.net
- WindowsLive RestService
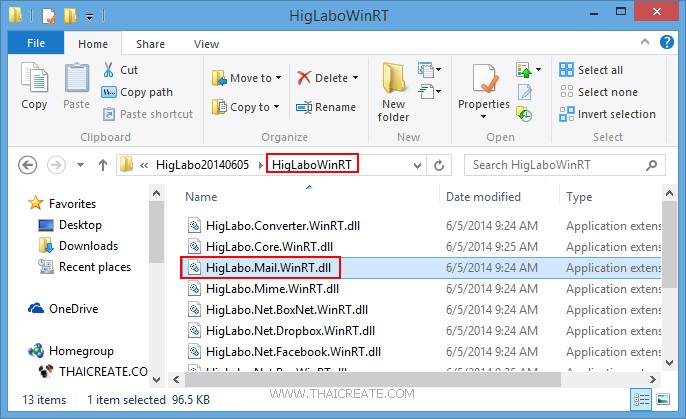
หลังจาก Download ไฟล์จะเห็นว่า HigLabo มี Library ให้เลือกใช้มากมาย ตามประเภทของการใช้งาน เช่น MAIL , POP และตัวที่เราจะใช้คือ HigLabo.Mail.WinRT.dll
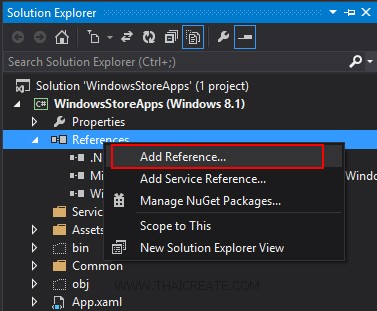
ให้คลิกวาที่ Reference -> Add Reference
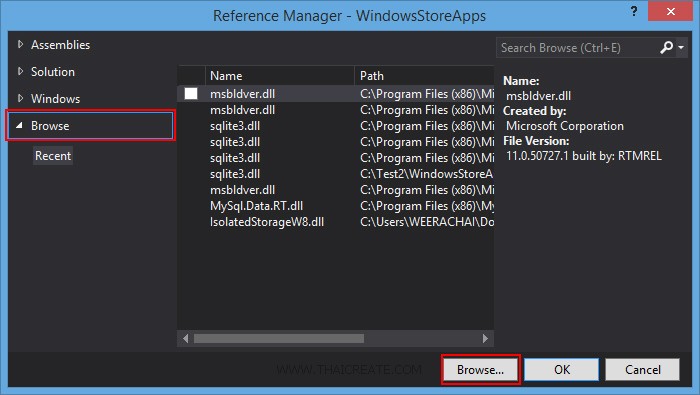
เลือก Browse จากนั้นเลือกไฟล์ dll ที่ต้องการ
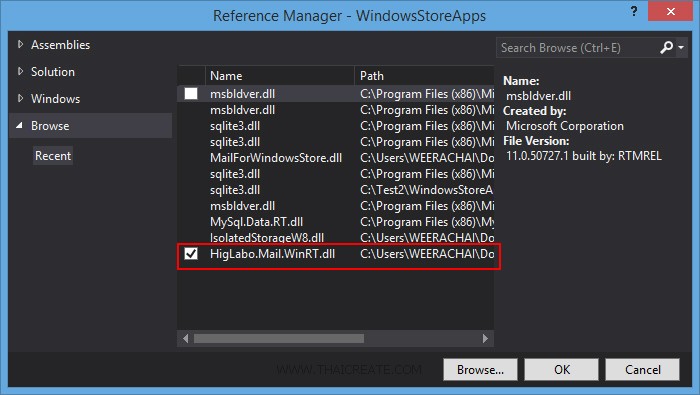
ได้ไฟล์ dll เข้ามาในรายการดังรูป
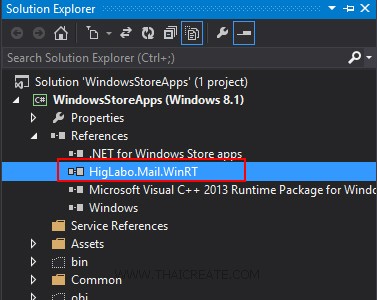
เข้ามาใน Reference เรียบร้อยแล้ว
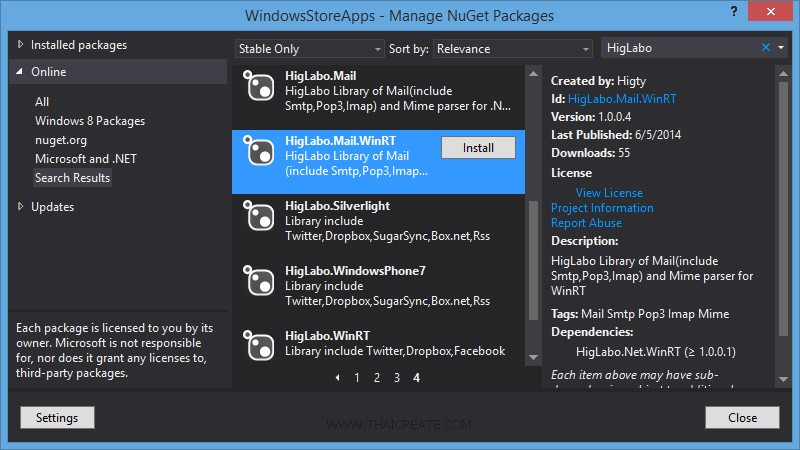
หรือจะใช้วิธีการติดตั้งผ่าน Nuget Packages ก็สามารถทำได้เช่นเดียวกัน
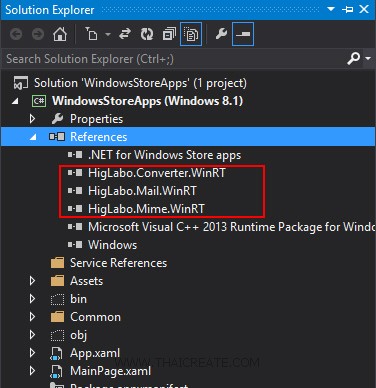
ตัวอย่างการ Add Reference ที่จำเป็นจะต้องใช้ในการเขียนเพื่อส่งอีเมล์ด้วย SMTP
รูปแบบการเขียน Windows Store Apps ด้วย C# สำหรับการส่งอีเมล์ด้วย SMTP
เรียกใช้ Library ของ HigLabo
using HigLabo.Net.Smtp;
using HigLabo.Core;
using HigLabo.Mime.Internal;
using HigLabo.Net.Mail;
using HigLabo.Converter;
Code สำหรับส่งอีเมล์
using (var cl = new SmtpClient())
{
cl.ServerName = "smtp.thaicreate.com";
cl.Port = "25";
cl.Tls = true;
cl.AuthenticateMode = SmtpAuthenticateMode.Auto;
cl.UserName = "[email protected]";
cl.Password = "password";
SmtpMessage mg = new SmtpMessage();
mg.Date = DateTime.Now.ToUniversalTime();
mg["Mime-Version"] = "1.0";
mg.From = "[email protected]";
mg.ReplyTo = "[email protected]";
mg.To.Add(new MailAddress("[email protected]"));
mg.Subject = "WinRT Test";
mg.BodyText = "<div style=\"font-size:32px;\">Large size</div>";
mg.IsHtml = true;
var im = await KnownFolders.PicturesLibrary.GetFileAsync("xxx.png");
var bb = await GetByteFromFile(im);
var ct = new SmtpContent();
ct.LoadData("image/png", bb);
mg.Contents.Add(ct);
String text = mg.GetDataText();
var rs = cl.SendMail(mg);
}
ซึ่งสิ่งที่จำเป็นจะต้องมีคือ Email Account ที่ประกอบด้วย SMTP / User และ Password ในการ Login ลองมาดูซะตัวอย่าง เพื่อความเข้าใจมากขึ้น
Example การเขียน Windows Store Apps ด้วยภาษา C# ไว้สำหรับการส่ง Email ด้วย SMTP
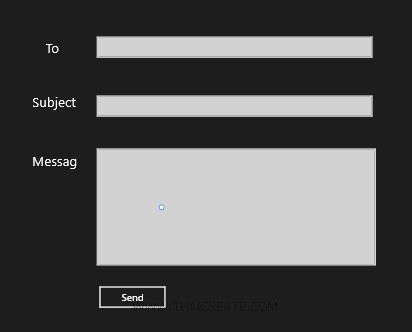
ออกแบบหน้าจอของ App ประกอบด้วย TextBox สำหรับรับข้อมูลเพื่อส่งอีเมล์ไปยัง User
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock HorizontalAlignment="Left" TextWrapping="Wrap" Text="To" FontSize="20" VerticalAlignment="Top" Margin="510,136,0,0"/>
<TextBox HorizontalAlignment="Left" TextWrapping="Wrap" x:Name="txtTo" VerticalAlignment="Top" Margin="586,129,0,0" Width="412"/>
<TextBox HorizontalAlignment="Left" TextWrapping="Wrap" x:Name="txtSubject" VerticalAlignment="Top" Margin="586,217,0,0" Width="412"/>
<TextBlock HorizontalAlignment="Left" TextWrapping="Wrap" Text="Subject" FontSize="20" VerticalAlignment="Top" Margin="490,217,0,0"/>
<TextBox HorizontalAlignment="Left" TextWrapping="Wrap" x:Name="txtMessage" VerticalAlignment="Top" Margin="586,296,0,0" Width="417" Height="175"/>
<TextBlock HorizontalAlignment="Left" TextWrapping="Wrap" Text="Messag" FontSize="20" VerticalAlignment="Top" Margin="490,304,0,0"/>
<Button Content="Send" x:Name="btnSend" HorizontalAlignment="Left" VerticalAlignment="Top" Margin="587,499,0,0" Width="105" Click="btnSend_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using HigLabo.Net.Smtp;
using HigLabo.Core;
using HigLabo.Mime.Internal;
using HigLabo.Net.Mail;
using HigLabo.Converter;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private void btnSend_Click(object sender, RoutedEventArgs e)
{
using (var cl = new SmtpClient())
{
cl.ServerName = "smtp.thaicreate.com";
cl.Port = "25";
cl.Tls = true;
cl.AuthenticateMode = SmtpAuthenticateMode.Auto;
cl.UserName = "[email protected]";
cl.Password = "password";
SmtpMessage mg = new SmtpMessage();
mg.Date = DateTime.Now.ToUniversalTime();
mg["Mime-Version"] = "1.0";
mg.From = "[email protected]";
mg.ReplyTo = "[email protected]";
mg.To.Add(new MailAddress(this.txtTo.Text));
mg.Subject = this.txtSubject.Text;
mg.BodyText = this.txtMessage.Text;
mg.IsHtml = true;
String text = mg.GetDataText();
var rs = cl.SendMail(mg);
}
}
}
}
Result
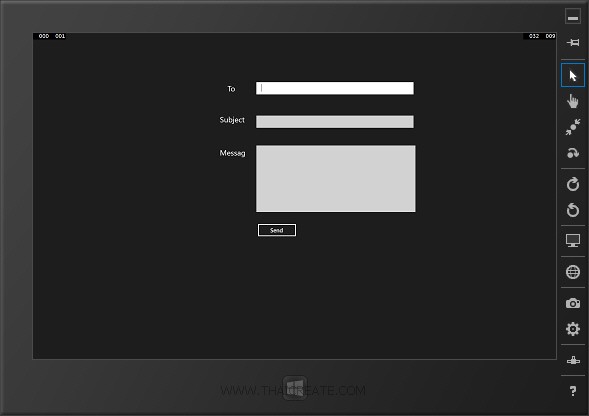
แบบ Form สำหรับ Input ข้อมูล แจะส่ง Email ไปยังปลายทาง
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2014-06-23 13:19:17 /
2017-03-19 15:12:43 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|