Windows Store Apps and Send Message / SMS (C#) |
Windows Store Apps and Send Message / SMS (C#) อุปกรณ์ที่ติดตั้ง Windows 8 นั้นในปัจจุบันไม่ได้มีเฉพาะ PC หรือ Notebook เท่านั้น แต่ยังมีอุปรกรณ์ประเภท Tablets หรือที่เรารู้จักกันในชื่อของ Surface โดยในบางรุ่นของ Surface สามารถใส่ Sim Card ได้ สามารถโทรออกและส่ง SMS ไปยังหมายโลขโทรศัพท์ปลายทางได้ ซึ่งปกติเราก็สามารถใช้ Apps มาที่พร้อมกับ Windows 8 ได้ หรือจะเขียน Apps บน Windows Store Apps ส่งก็ได้เช่นเดียวกัน
Windows Store Apps and Send Message / SMS (C#)
วิธีการส่ง SMS บน Windows Store Apps
เรียกใช้ Class ของ Windows.Devices.Sms
using Windows.Devices.Sms;
ส่ง SMS ออกจากเครื่องด้วยคำสั่ง
device = await SmsDevice.GetDefaultAsync();
SmsTextMessage msg = new SmsTextMessage();
msg.To = PHONE_NUMBER;
msg.Body = MESSAGE;
await device.SendMessageAsync(msg);
Example ตัวอย่างการเขียน Windows Store Apps ด้วยภาษา C# เพื่อส่ง SMS
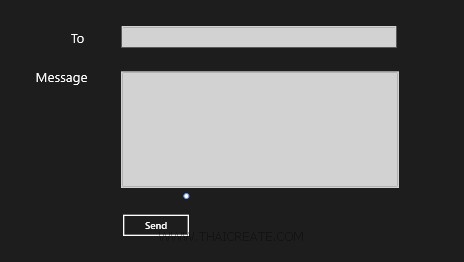
ออกแบบหน้าจอสำหรับ Input หมายเลขโทรศัพท์ และ ข้อความ
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
xmlns:bm="using:Bing.Maps">
<Grid Background="{StaticResource ApplicationPageBackgroundThemeBrush}">
<TextBlock HorizontalAlignment="Left" TextWrapping="Wrap" Text="To" FontSize="20" VerticalAlignment="Top" Margin="510,136,0,0"/>
<TextBox HorizontalAlignment="Left" TextWrapping="Wrap" x:Name="txtTo" VerticalAlignment="Top" Margin="586,129,0,0" Width="412"/>
<TextBox HorizontalAlignment="Left" TextWrapping="Wrap" x:Name="txtMessage" VerticalAlignment="Top" Margin="585,197,0,0" Width="417" Height="175"/>
<TextBlock HorizontalAlignment="Left" TextWrapping="Wrap" Text="Message" FontSize="20" VerticalAlignment="Top" Margin="457,195,0,0" Width="83"/>
<Button Content="Send" x:Name="btnSend" HorizontalAlignment="Left" VerticalAlignment="Top" Margin="585,409,0,0" Width="105" Click="btnSend_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using Windows.UI.Popups;
using Windows.Devices.Sms;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
private SmsDevice device;
public MainPage()
{
this.InitializeComponent();
}
private async void btnSend_Click(object sender, RoutedEventArgs e)
{
try
{
device = await SmsDevice.GetDefaultAsync();
SmsTextMessage msg = new SmsTextMessage();
msg.To = this.txtTo.Text;
msg.Body = this.txtMessage.Text;
await device.SendMessageAsync(msg);
MessageDialog msgDialog = new MessageDialog("Text message sent", "Status");
await msgDialog.ShowAsync();
}
catch (Exception err)
{
device = null;
}
}
}
}
Result
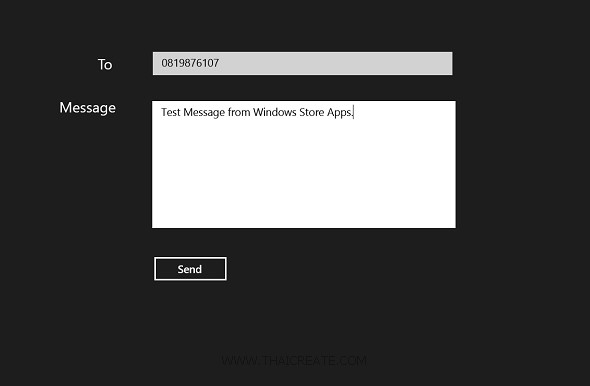
ผลลัพธ์ที่ได้ ในกรณีที่อุปกรณ์ที่ติดตั้ง Apps มี Sim Card ที่สามารถส่ง SMS ได้ โปรแกรมก็จะทำการส่ง SMS ไปยังหมายเลขปลายทาง
นอกจากนี้ยังมีตัวอย่างการดึง SMS จากระบบได้เช่น
// Handle a request to listen for received messages.
private async void Receive_Click(object sender, RoutedEventArgs e)
{
// If this is the first request, get the default SMS device. If this
// is the first SMS device access, the user will be prompted to grant
// access permission for this application.
if (device == null)
{
try
{
rootPage.NotifyUser("Getting default SMS device ...", NotifyType.StatusMessage);
device = await SmsDevice.GetDefaultAsync();
}
catch (Exception ex)
{
rootPage.NotifyUser("Failed to find SMS device\n" + ex.Message, NotifyType.ErrorMessage);
return;
}
}
// Attach a message received handler to the device, if not already listening
if (!listening)
{
try
{
msgCount = 0;
device.SmsMessageReceived += device_SmsMessageReceived;
listening = true;
rootPage.NotifyUser("Waiting for message ...", NotifyType.StatusMessage);
}
catch (Exception ex)
{
rootPage.NotifyUser(ex.Message, NotifyType.ErrorMessage);
// On failure, release the device. If the user revoked access or the device
// is removed, a new device object must be obtained.
device = null;
}
}
}
Reference : http://code.msdn.microsoft.com/windowsapps/Sms-SendReceive-fa02e55e
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2014-06-23 13:19:41 /
2017-03-19 15:11:56 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|