ดาวน์โหลดจัดเก็บไฟล์ลงบน Storage ของ Windows Store Apps (C#) |
ดาวน์โหลดจัดเก็บไฟล์ลงบน Storage ของ Windows Store Apps (C#) ตัวอย่างนี้จะเป็นการเขียน Apps บน Windows Store เพื่อเรียกไฟล์ที่อยู่บน URL และ Download ไฟล์ที่อยู่ในรูปแบบของ URL ของเว็บไซต์ ซึ่งจะเป็น URL ของรูปภาพ โดยจะทำการ Download และ Save ไฟล์นั้นลงใน Storage ของ Windows Store Apps ซึ่งประกอบด้วย Local data, Roaming data และ Temporary data.
Syntax การ Download ไฟล์จาก URL ลงใน Storage
var httpClient = new HttpClient();
string strURL = this.txtURL.Text;
HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Get, strURL);
HttpResponseMessage response = await httpClient.SendAsync(request,
HttpCompletionOption.ResponseHeadersRead);
var imageFile = await ApplicationData.Current.RoamingFolder.CreateFileAsync(fileName,
CreationCollisionOption.ReplaceExisting);
var fs = await imageFile.OpenAsync(FileAccessMode.ReadWrite);
DataWriter writer = new DataWriter(fs.GetOutputStreamAt(0));
writer.WriteBytes(await response.Content.ReadAsByteArrayAsync());
await writer.StoreAsync();
writer.DetachStream();
await fs.FlushAsync();
fs.Dispose();
writer.Dispose();
ตัวอย่างการเขียน Apps เพื่อ Downloadไฟล์จาก URL ลงใน Storage บน Windows Store Apps
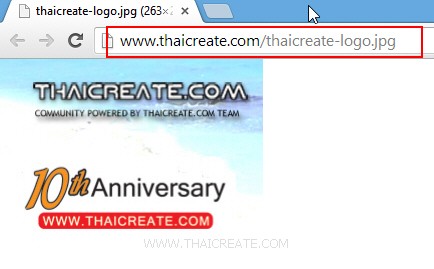
ทดสอบเรียกไฟล์ที่อยู่บน URL
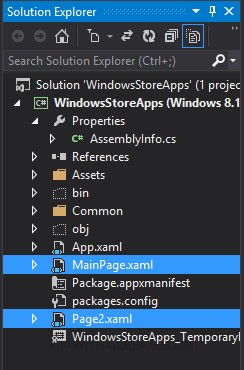
สร้าง Page ขึ้นมา 2 เพจ คือ MainPage.xaml (สำหรับ Download และ Save ไฟล์) และ Page2.xaml (สำหรับแสดงรายการไฟล์รูป)
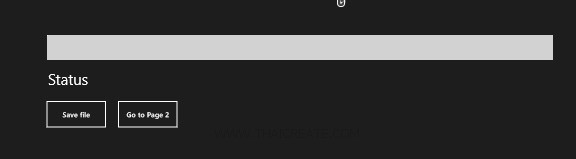
เพจของ MainPage.xaml
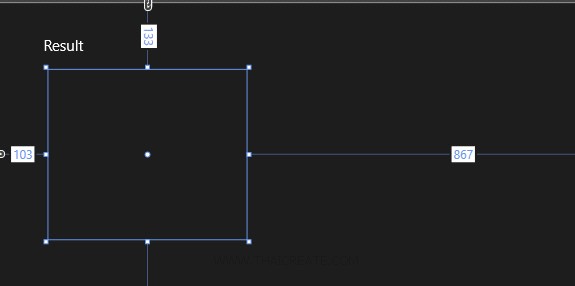
เพจของ Page2.xaml
Example 1 สร้าง Input เพื่อรับค่า URL ไฟล์ Download และจัดเก็บใน Local Data บน Windows Store Apps
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBox x:Name="txtURL" HorizontalAlignment="Left" Height="50" Margin="96,72,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="1012" FontSize="30"/>
<TextBlock x:Name="lblStatus" HorizontalAlignment="Left" Margin="98,144,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="30" Text="Status"/>
<Button x:Name="btnSave" Content="Save file" HorizontalAlignment="Left" Margin="92,201,0,0" VerticalAlignment="Top" Height="59" Width="125" Click="btnSavefile_Click"/>
<Button x:Name="btnGoPage2" Content="Go to Page 2" HorizontalAlignment="Left" Margin="235,201,0,0" VerticalAlignment="Top" Height="59" Width="125" Click="btnGoPage2_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.Net.Http;
using Windows.UI.Xaml.Media.Imaging;
using Windows.Storage;
using Windows.Storage.AccessCache;
using Windows.Storage.Pickers;
using Windows.Storage.Provider;
using Windows.Storage.Streams;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private string fileName = "thaicreate-logo.jpg";
protected override void OnNavigatedTo(NavigationEventArgs e)
{
}
private async void btnSavefile_Click(object sender, RoutedEventArgs e)
{
var httpClient = new HttpClient();
HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Get, this.txtURL.Text);
HttpResponseMessage response = await httpClient.SendAsync(request,
HttpCompletionOption.ResponseHeadersRead);
var imageFile = await ApplicationData.Current.LocalFolder.CreateFileAsync(fileName,
CreationCollisionOption.ReplaceExisting);
var fs = await imageFile.OpenAsync(FileAccessMode.ReadWrite);
DataWriter writer = new DataWriter(fs.GetOutputStreamAt(0));
writer.WriteBytes(await response.Content.ReadAsByteArrayAsync());
await writer.StoreAsync();
writer.DetachStream();
await fs.FlushAsync();
fs.Dispose();
writer.Dispose();
this.lblStatus.Text = "File has been download to LocalFolder (" + fileName + ")";
}
private void btnGoPage2_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2), fileName);
}
}
}
Page2.xaml
<Page
x:Class="WindowsStoreApps.Page2"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblResult" HorizontalAlignment="Left" Margin="93,69,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="30" Text="Result"/>
<Image x:Name="imgView" Margin="103,133,867,296"/>
</Grid>
</Page>
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.IO;
using Windows.UI.Xaml.Media.Imaging;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
string fileName = e.Parameter as string;
if (!string.IsNullOrWhiteSpace(fileName))
{
this.lblResult.Text = "File Name = " + fileName;
}
Windows.Storage.StorageFolder localFolder = Windows.Storage.ApplicationData.Current.LocalFolder;
Windows.Storage.StorageFile sampleFile = await localFolder.GetFileAsync(fileName);
Windows.Storage.Streams.IRandomAccessStream stream = await sampleFile.OpenReadAsync();
BitmapImage bitmapImage = new BitmapImage();
bitmapImage.SetSource(stream);
this.imgView.Source = bitmapImage;
}
}
}
Screenshot
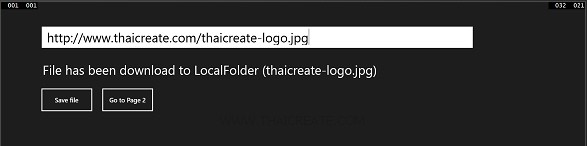
แสดง Input เพื่อรับค่า URL และ Download ไฟล์ลงใน Storage ของ Windows Store Apps และให้คลิกที่ Go to Page 2 เพื่อดูไฟล์ที่ถูก Save ลงใน Storage
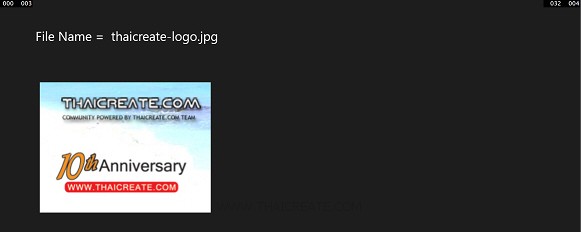
แสดงไฟล์ที่ถูก Save ลงใน Storage
สำหรับตัวอย่างแรกเป็นการจัดเก็บลงใน Local data แต่ในกรณีที่ต้องการจัดเก็บลงใน Roaming หรือ Temporary สามารถดูได้ต่อในตัวอย่างที่ 2 และ 3
Example 2 ทดสอบการจัดเก็บลงใน Roaming (RoamingFolder)
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.Net.Http;
using Windows.UI.Xaml.Media.Imaging;
using Windows.Storage;
using Windows.Storage.AccessCache;
using Windows.Storage.Pickers;
using Windows.Storage.Provider;
using Windows.Storage.Streams;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private string fileName = "thaicreate-logo.jpg";
protected override void OnNavigatedTo(NavigationEventArgs e)
{
}
private async void btnSavefile_Click(object sender, RoutedEventArgs e)
{
var httpClient = new HttpClient();
HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Get, this.txtURL.Text);
HttpResponseMessage response = await httpClient.SendAsync(request,
HttpCompletionOption.ResponseHeadersRead);
var imageFile = await ApplicationData.Current.RoamingFolder.CreateFileAsync(fileName,
CreationCollisionOption.ReplaceExisting);
var fs = await imageFile.OpenAsync(FileAccessMode.ReadWrite);
DataWriter writer = new DataWriter(fs.GetOutputStreamAt(0));
writer.WriteBytes(await response.Content.ReadAsByteArrayAsync());
await writer.StoreAsync();
writer.DetachStream();
await fs.FlushAsync();
fs.Dispose();
writer.Dispose();
this.lblStatus.Text = "File has been download to LocalFolder (" + fileName + ")";
}
private void btnGoPage2_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2), fileName);
}
}
}
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.IO;
using Windows.UI.Xaml.Media.Imaging;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
string fileName = e.Parameter as string;
if (!string.IsNullOrWhiteSpace(fileName))
{
this.lblResult.Text = "File Name = " + fileName;
}
Windows.Storage.StorageFolder roamingFolder = Windows.Storage.ApplicationData.Current.RoamingFolder;
Windows.Storage.StorageFile sampleFile = await roamingFolder.GetFileAsync(fileName);
Windows.Storage.Streams.IRandomAccessStream stream = await sampleFile.OpenReadAsync();
BitmapImage bitmapImage = new BitmapImage();
bitmapImage.SetSource(stream);
this.imgView.Source = bitmapImage;
}
}
}
Example 3 ทดสอบการจัดเก็บลงใน Temporary (TemporaryFolder)
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.Net.Http;
using Windows.UI.Xaml.Media.Imaging;
using Windows.Storage;
using Windows.Storage.AccessCache;
using Windows.Storage.Pickers;
using Windows.Storage.Provider;
using Windows.Storage.Streams;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private string fileName = "thaicreate-logo.jpg";
protected override void OnNavigatedTo(NavigationEventArgs e)
{
}
private async void btnSavefile_Click(object sender, RoutedEventArgs e)
{
var httpClient = new HttpClient();
HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Get, this.txtURL.Text);
HttpResponseMessage response = await httpClient.SendAsync(request,
HttpCompletionOption.ResponseHeadersRead);
var imageFile = await ApplicationData.Current.TemporaryFolder.CreateFileAsync(fileName,
CreationCollisionOption.ReplaceExisting);
var fs = await imageFile.OpenAsync(FileAccessMode.ReadWrite);
DataWriter writer = new DataWriter(fs.GetOutputStreamAt(0));
writer.WriteBytes(await response.Content.ReadAsByteArrayAsync());
await writer.StoreAsync();
writer.DetachStream();
await fs.FlushAsync();
fs.Dispose();
writer.Dispose();
this.lblStatus.Text = "File has been download to LocalFolder (" + fileName + ")";
}
private void btnGoPage2_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2), fileName);
}
}
}
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.IO;
using Windows.UI.Xaml.Media.Imaging;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
string fileName = e.Parameter as string;
if (!string.IsNullOrWhiteSpace(fileName))
{
this.lblResult.Text = "File Name = " + fileName;
}
Windows.Storage.StorageFolder temporaryFolder = Windows.Storage.ApplicationData.Current.TemporaryFolder;
Windows.Storage.StorageFile sampleFile = await temporaryFolder.GetFileAsync(fileName);
Windows.Storage.Streams.IRandomAccessStream stream = await sampleFile.OpenReadAsync();
BitmapImage bitmapImage = new BitmapImage();
bitmapImage.SetSource(stream);
this.imgView.Source = bitmapImage;
}
}
}

.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
|
|
|
Create/Update Date : |
2014-03-17 12:18:49 /
2017-03-19 14:53:56 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|