File Dialog และ Save จัดเก็บไฟล์ลง Storage ของ Windows Store Apps (C#) |
File Dialog และ Save จัดเก็บไฟล์ลง Storage ของ Windows Store Apps (C#) บทความนี้จะเป็นตัวอย่างการเขียน Apps บน Windows Store โดยจะยกตัวอย่างการเขียน Apps เพื่อจัดเก็บไฟล์รูปภาพ (Image) ผ่านการ Browse เลือกไฟล์ด้วย File Dialog โดยผู้ใช้จะทำการเลือกภาพที่อยู่บนเครื่องที่ติดตั้ง Apps และเมื่อเลือกไฟล์นั้น ๆ แล้ว จะจัดเก็บลงใน Storage ของ Apps ซึ่งประกอบด้วย Local data, Roaming data และ Temporary data.
Syntax การ Save ไฟล์ลงใน Storage ของ Windows Store Apps
using (IRandomAccessStream fileStream = await file.OpenAsync(Windows.Storage.FileAccessMode.Read))
{
Windows.Storage.StorageFolder localFolder = Windows.Storage.ApplicationData.Current.LocalFolder;
StorageFile sampleFile = await localFolder.CreateFileAsync(fileName,
Windows.Storage.CreationCollisionOption.ReplaceExisting);
var size = fileStream.Size;
DataReader dataReader = new DataReader(fileStream);
await dataReader.LoadAsync((uint)size);
byte[] buffer = new byte[(int)size];
dataReader.ReadBytes(buffer);
await Windows.Storage.FileIO.WriteBytesAsync(sampleFile, buffer);
this.lblStatus.Text = "File has been save to LocalFolder (" + fileName + ")";
}
ตัวอย่างการสร้าง File Dialog และ Save ลงใน Storage บน Windows Store Apps
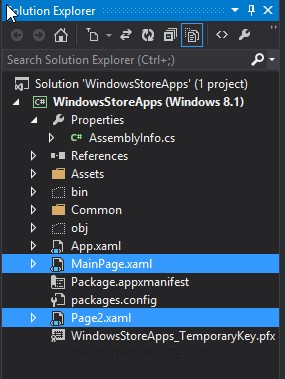
สร้าง Page ขึ้นมา 2 เพจ คือ MainPage.xaml (สำหรับ Browse และ Save ไฟล์) และ Page2.xaml (สำหรับแสดงรายการไฟล์รูป)
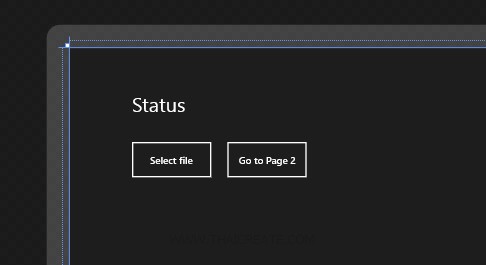
เพจของ MainPage.xaml
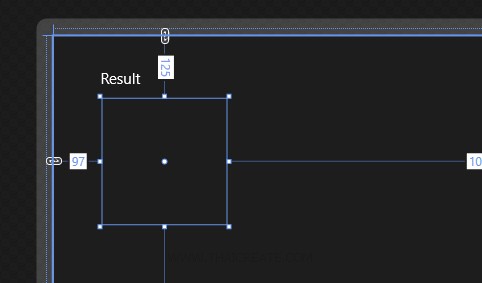
เพจของ Page2.xaml
Example 1 สร้าง Dialog File เพื่อ Browse เลือกไฟล์ และจัดเก็บใน Local Data บน Windows Store Apps
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblStatus" HorizontalAlignment="Left" Margin="93,69,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="30" Text="Status"/>
<Button x:Name="btnSelectfile" Content="Select file" HorizontalAlignment="Left" Margin="90,138,0,0" VerticalAlignment="Top" Height="59" Width="125" Click="btnSelectfile_Click"/>
<Button x:Name="btnGoPage2" Content="Go to Page 2" HorizontalAlignment="Left" Margin="233,138,0,0" VerticalAlignment="Top" Height="59" Width="125" Click="btnGoPage2_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.IO;
using Windows.UI.Xaml.Media.Imaging;
using Windows.Storage;
using Windows.Storage.AccessCache;
using Windows.Storage.Pickers;
using Windows.Storage.Provider;
using Windows.Storage.Streams;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private string fileName = string.Empty;
protected override void OnNavigatedTo(NavigationEventArgs e)
{
}
private async void btnSelectfile_Click(object sender, RoutedEventArgs e)
{
FileOpenPicker picker = new FileOpenPicker();
picker.FileTypeFilter.Add(".png");
picker.FileTypeFilter.Add(".jpg");
picker.FileTypeFilter.Add(".gif");
picker.FileTypeFilter.Add(".bmp");
picker.SuggestedStartLocation = PickerLocationId.Desktop;
picker.ViewMode = PickerViewMode.Thumbnail;
StorageFile file = await picker.PickSingleFileAsync();
fileName = file.Name;
if (file != null)
{
using (IRandomAccessStream fileStream = await file.OpenAsync(Windows.Storage.FileAccessMode.Read))
{
Windows.Storage.StorageFolder localFolder = Windows.Storage.ApplicationData.Current.LocalFolder;
StorageFile sampleFile = await localFolder.CreateFileAsync(fileName,
Windows.Storage.CreationCollisionOption.ReplaceExisting);
var size = fileStream.Size;
DataReader dataReader = new DataReader(fileStream);
await dataReader.LoadAsync((uint)size);
byte[] buffer = new byte[(int)size];
dataReader.ReadBytes(buffer);
await Windows.Storage.FileIO.WriteBytesAsync(sampleFile, buffer);
this.lblStatus.Text = "File has been save to LocalFolder (" + fileName + ")";
}
}
}
private void btnGoPage2_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2), fileName);
}
}
}
Page2.xaml
<Page
x:Class="WindowsStoreApps.Page2"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblResult" HorizontalAlignment="Left" Margin="93,69,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="30" Text="Result"/>
<Image x:Name="imgView" Margin="97,125,1021,392"/>
</Grid>
</Page>
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.IO;
using Windows.UI.Xaml.Media.Imaging;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
string fileName = e.Parameter as string;
if (!string.IsNullOrWhiteSpace(fileName))
{
this.lblResult.Text = "File Name = " + fileName;
}
Windows.Storage.StorageFolder localFolder = Windows.Storage.ApplicationData.Current.LocalFolder;
Windows.Storage.StorageFile sampleFile = await localFolder.GetFileAsync(fileName);
//Windows.Storage.Streams.IBuffer buffer = await Windows.Storage.FileIO.ReadBufferAsync(sampleFile);
//byte[] bytes = buffer.ToArray();
//Stream stream = (await sampleFile.OpenReadAsync()).AsStreamForRead();
//BinaryReader reader = new BinaryReader(stream);
Windows.Storage.Streams.IRandomAccessStream stream = await sampleFile.OpenReadAsync();
BitmapImage bitmapImage = new BitmapImage();
bitmapImage.SetSource(stream);
this.imgView.Source = bitmapImage;
}
}
}
Screenshot
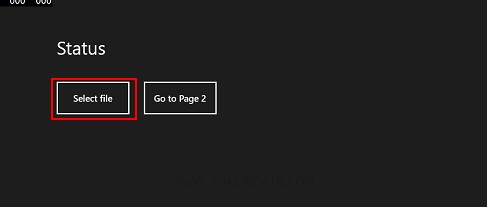
คลิกเลือก Select File

เลือกไฟล์รูปภาพที่อยู่บน Desktop หลังจากนั้นเลือก Open
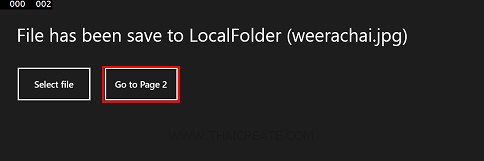
จากนั้นโปรแกรมจะทำการ Save ลงใน Storage ของ Local data สามารถคลิกที่ Go to Page 2 เพื่อเรียกไฟล์จาก Storage มาแสดง
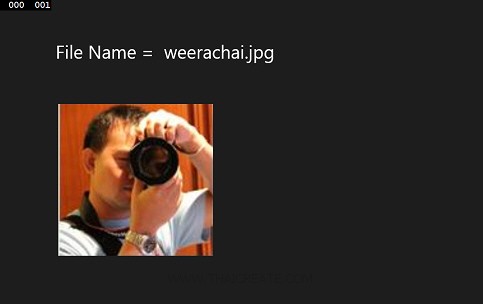
แสดงภาพจาก Storage ที่อ่านมาจาก Local data
สำหรับตัวอย่างแรกเป็นการจัดเก็บลงใน Local data แต่ในกรณีที่ต้องการจัดเก็บลงใน Roaming หรือ Temporary สามารถดูได้ต่อในตัวอย่างที่ 2 และ 3
Example 2 ทดสอบการจัดเก็บลงใน Roaming (RoamingFolder)
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.IO;
using Windows.UI.Xaml.Media.Imaging;
using Windows.Storage;
using Windows.Storage.AccessCache;
using Windows.Storage.Pickers;
using Windows.Storage.Provider;
using Windows.Storage.Streams;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private string fileName = string.Empty;
protected override void OnNavigatedTo(NavigationEventArgs e)
{
}
private async void btnSelectfile_Click(object sender, RoutedEventArgs e)
{
FileOpenPicker picker = new FileOpenPicker();
picker.FileTypeFilter.Add(".png");
picker.FileTypeFilter.Add(".jpg");
picker.FileTypeFilter.Add(".gif");
picker.FileTypeFilter.Add(".bmp");
picker.SuggestedStartLocation = PickerLocationId.Desktop;
picker.ViewMode = PickerViewMode.Thumbnail;
StorageFile file = await picker.PickSingleFileAsync();
fileName = file.Name;
if (file != null)
{
using (IRandomAccessStream fileStream = await file.OpenAsync(Windows.Storage.FileAccessMode.Read))
{
Windows.Storage.StorageFolder roamingFolder = Windows.Storage.ApplicationData.Current.RoamingFolder;
StorageFile sampleFile = await roamingFolder.CreateFileAsync(fileName,
Windows.Storage.CreationCollisionOption.ReplaceExisting);
var size = fileStream.Size;
DataReader dataReader = new DataReader(fileStream);
await dataReader.LoadAsync((uint)size);
byte[] buffer = new byte[(int)size];
dataReader.ReadBytes(buffer);
await Windows.Storage.FileIO.WriteBytesAsync(sampleFile, buffer);
this.lblStatus.Text = "File has been save to RoamingFolder (" + fileName + ")";
}
}
}
private void btnGoPage2_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2), fileName);
}
}
}
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.IO;
using Windows.UI.Xaml.Media.Imaging;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
string fileName = e.Parameter as string;
if (!string.IsNullOrWhiteSpace(fileName))
{
this.lblResult.Text = "File Name = " + fileName;
}
Windows.Storage.StorageFolder roamingFolder = Windows.Storage.ApplicationData.Current.RoamingFolder;
Windows.Storage.StorageFile sampleFile = await roamingFolder.GetFileAsync(fileName);
//Windows.Storage.Streams.IBuffer buffer = await Windows.Storage.FileIO.ReadBufferAsync(sampleFile);
//byte[] bytes = buffer.ToArray();
//Stream stream = (await sampleFile.OpenReadAsync()).AsStreamForRead();
//BinaryReader reader = new BinaryReader(stream);
Windows.Storage.Streams.IRandomAccessStream stream = await sampleFile.OpenReadAsync();
BitmapImage bitmapImage = new BitmapImage();
bitmapImage.SetSource(stream);
this.imgView.Source = bitmapImage;
}
}
}
Example 3 ทดสอบการจัดเก็บลงใน Temporary (TemporaryFolder)
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.IO;
using Windows.UI.Xaml.Media.Imaging;
using Windows.Storage;
using Windows.Storage.AccessCache;
using Windows.Storage.Pickers;
using Windows.Storage.Provider;
using Windows.Storage.Streams;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private string fileName = string.Empty;
protected override void OnNavigatedTo(NavigationEventArgs e)
{
}
private async void btnSelectfile_Click(object sender, RoutedEventArgs e)
{
FileOpenPicker picker = new FileOpenPicker();
picker.FileTypeFilter.Add(".png");
picker.FileTypeFilter.Add(".jpg");
picker.FileTypeFilter.Add(".gif");
picker.FileTypeFilter.Add(".bmp");
picker.SuggestedStartLocation = PickerLocationId.Desktop;
picker.ViewMode = PickerViewMode.Thumbnail;
StorageFile file = await picker.PickSingleFileAsync();
fileName = file.Name;
if (file != null)
{
using (IRandomAccessStream fileStream = await file.OpenAsync(Windows.Storage.FileAccessMode.Read))
{
Windows.Storage.StorageFolder temporaryFolder = ApplicationData.Current.TemporaryFolder;
StorageFile sampleFile = await temporaryFolder.CreateFileAsync(fileName,
Windows.Storage.CreationCollisionOption.ReplaceExisting);
var size = fileStream.Size;
DataReader dataReader = new DataReader(fileStream);
await dataReader.LoadAsync((uint)size);
byte[] buffer = new byte[(int)size];
dataReader.ReadBytes(buffer);
await Windows.Storage.FileIO.WriteBytesAsync(sampleFile, buffer);
this.lblStatus.Text = "File has been save to TemporaryFolder (" + fileName + ")";
}
}
}
private void btnGoPage2_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2), fileName);
}
}
}
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.IO;
using Windows.UI.Xaml.Media.Imaging;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
string fileName = e.Parameter as string;
if (!string.IsNullOrWhiteSpace(fileName))
{
this.lblResult.Text = "File Name = " + fileName;
}
Windows.Storage.StorageFolder temporaryFolder = Windows.Storage.ApplicationData.Current.TemporaryFolder;
Windows.Storage.StorageFile sampleFile = await temporaryFolder.GetFileAsync(fileName);
//Windows.Storage.Streams.IBuffer buffer = await Windows.Storage.FileIO.ReadBufferAsync(sampleFile);
//byte[] bytes = buffer.ToArray();
//Stream stream = (await sampleFile.OpenReadAsync()).AsStreamForRead();
//BinaryReader reader = new BinaryReader(stream);
Windows.Storage.Streams.IRandomAccessStream stream = await sampleFile.OpenReadAsync();
BitmapImage bitmapImage = new BitmapImage();
bitmapImage.SetSource(stream);
this.imgView.Source = bitmapImage;
}
}
}
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
|
|
|
Create/Update Date : |
2014-03-17 12:17:54 /
2017-03-19 14:54:41 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|