Roaming app data จัดเก็บไฟล์ลง Storage บน Windows Store Apps (C#) |
Roaming app data จัดเก็บไฟล์ลง Storage บน Windows Store Apps (C#) สำหรับการจัดเก็บข้อมูลแบบ Roaming บน Windows Store Apps สามารถจัดเก็บข้อมูลประเภทต่าง ๆ ได้เหมือนกับ Local app data เช่น ตัวแปรแบบ Settings , ตัวแปรแบบ Object และไฟล์ประเภทต่าง ๆ ได้ทุกประเภท แต่ Roaming app data จะเป็นการจัดเก็บไว้บน Server ของ Cloud Windows Store ซึ่งแบบ Roaming นั้น จะควบคุมจัดการให้ทุกๆเครื่องที่มีผู้ใช้คนเดียวกันมีข้อมูลเหมือนกัน และเรียกใช้ข้อมูลได้เหมือนกันทุกประการ ไม่ว่าจะเรียกใช้จากเครื่องไหนก็ตาม ซึ่งจะแตกต่างกับ Local app data ตรงที่ Local จะเก็บเฉพาะเครื่องนั้น ๆ เท่านั้น
เพิ่มเติม การจัดเก็บข้อมูล Roaming นิยมจัดเก็บข้อมูลเล็ก ๆ ที่มีขนาดไม่ใหญ่ เช่นค่า Setting , Preferences และ Customization เพราะถ้าใช้ Roaming เก็บข้อมูลมากจนเกินไป Apps ของเราอาจจะถูกระงับการรับส่งข้อมูลผ่าน Roaming ได้
ตัวอย่างการจัดเก็บค่าแบบ Settings
สร้างตัวแปร Settings
Windows.Storage.ApplicationDataContainer roamingSettings = Windows.Storage.ApplicationData.Current.RoamingSettings;
// Simple setting
roamingSettings.Values["exampleSetting"] = "Hello World";
// High Priority setting, for example, last page position in book reader app
roamingSettings.values["HighPriority"] = "65";
อ่านตัวแปร Settings
Windows.Storage.ApplicationDataContainer roamingSettings = Windows.Storage.ApplicationData.Current.RoamingSettings;
// Simple setting
Object value = roamingSettings.Values["exampleSetting"];
ตัวอย่างการจัดเก็บค่าแบบ Object
สร้างตัวแปร Object
// Composite setting
Windows.Storage.ApplicationDataCompositeValue composite = new Windows.Storage.ApplicationDataCompositeValue();
composite["intVal"] = 1;
composite["strVal"] = "string";
roamingSettings.Values["exampleCompositeSetting"] = composite;
อ่านตัวแปร Object
// Composite setting
Windows.Storage.ApplicationDataCompositeValue composite =
(Windows.Storage.ApplicationDataCompositeValue)roamingSettings.Values["exampleCompositeSetting"];
if (composite == null)
{
// No data
}
else
{
// Access data in composite["intVal"] and composite["strVal"]
}
ตัวอย่างการจัดเก็บแบบ Text file
การจัดเก็บแบบ Text file
async void WriteTimestamp()
{
Windows.Globalization.DateTimeFormatting.DateTimeFormatter formatter =
new Windows.Globalization.DatetimeFormatting.DateTimeFormatter("longtime");
StorageFile sampleFile = await roamingFolder.CreateFileAsync("dataFile.txt",
CreationCollisionOption.ReplaceExisting);
await FileIO.WriteTextAsync(sampleFile, formatter.Format(DateTime.Now));
}
การอ่าน Text file
async void ReadTimestamp()
{
try
{
StorageFile sampleFile = await roamingFolder.GetFileAsync("dataFile.txt");
String timestamp = await FileIO.ReadTextAsync(sampleFile);
// Data is contained in timestamp
}
catch (Exception)
{
// Timestamp not found
}
}
ลองมาดูตัวอย่างเพื่อความเข้าใจมากขึ้น
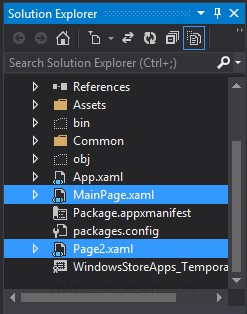
สร้าง Page ขึ้นมา 2 Page โดยเพจแรกใช้สร้างตัวแปร และ เพจสอง ใช้สำหรับการอ่านค่า
Example 1 สร้างตัวแปร Settings และอ่านตัวแปร Settings (Roaming app data)
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblStatus" HorizontalAlignment="Left" Margin="93,69,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="30" Text="Status"/>
<Button x:Name="btnGoPage2" Content="Go to Page 2" HorizontalAlignment="Left" Margin="90,135,0,0" VerticalAlignment="Top" Height="59" Width="125" Click="btnGoPage2_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
Windows.Storage.ApplicationDataContainer roamingSettings = Windows.Storage.ApplicationData.Current.RoamingSettings;
roamingSettings.Values["exampleSetting"] = "Hello World";
this.lblStatus.Text = "Roaming app data has been created.";
}
private void btnGoPage2_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2));
}
}
}
Page2.xaml
<Page
x:Class="WindowsStoreApps.Page2"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblResult" HorizontalAlignment="Left" Margin="93,69,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="30" Text="Result"/>
</Grid>
</Page>
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
Windows.Storage.ApplicationDataContainer roamingSettings = Windows.Storage.ApplicationData.Current.RoamingSettings;
Object value = roamingSettings.Values["exampleSetting"];
this.lblResult.Text = value.ToString();
}
}
}
Screenshot
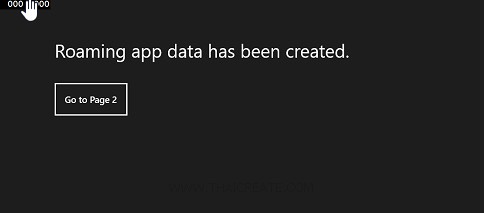
สร้างค่าตัวแปร
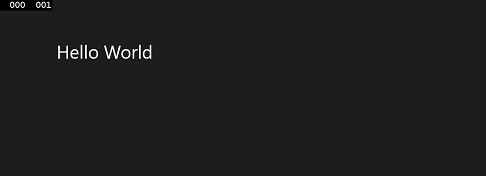
แสดงค่าตัวแปร
Example 2 สร้างตัวแปร Object และอ่านตัวแปร Object (Roaming app data)
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblStatus" HorizontalAlignment="Left" Margin="93,69,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="30" Text="Status"/>
<Button x:Name="btnGoPage2" Content="Go to Page 2" HorizontalAlignment="Left" Margin="90,135,0,0" VerticalAlignment="Top" Height="59" Width="125" Click="btnGoPage2_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
Windows.Storage.ApplicationDataContainer roamingSettings = Windows.Storage.ApplicationData.Current.RoamingSettings;
Windows.Storage.ApplicationDataCompositeValue composite = new Windows.Storage.ApplicationDataCompositeValue();
composite["intVal"] = 1;
composite["strVal"] = "My Value";
roamingSettings.Values["exampleCompositeSetting"] = composite;
this.lblStatus.Text = "Roaming app data has been created.";
}
private void btnGoPage2_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2));
}
}
}
Page2.xaml
<Page
x:Class="WindowsStoreApps.Page2"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblResult" HorizontalAlignment="Left" Margin="93,69,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="30" Text="Result"/>
</Grid>
</Page>
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
Windows.Storage.ApplicationDataContainer roamingSettings = Windows.Storage.ApplicationData.Current.RoamingSettings;
Windows.Storage.ApplicationDataCompositeValue composite =
(Windows.Storage.ApplicationDataCompositeValue) roamingSettings.Values["exampleCompositeSetting"];
if (composite != null)
{
this.lblResult.Text = "intVal = " + composite["intVal"] + " and strVal = " + composite["strVal"];
}
}
}
}
Screenshot
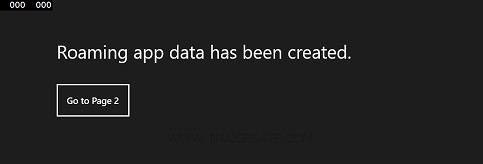
สร้างตัวแปร
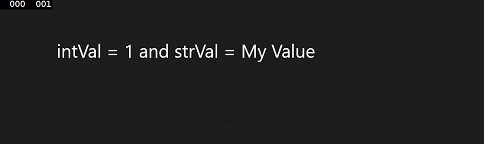
แสดงค่าตัวแปร
Example 3 สร้าง Text file และการอ่าน Text file (Roaming app data)
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblStatus" HorizontalAlignment="Left" Margin="93,69,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="30" Text="Status"/>
<Button x:Name="btnGoPage2" Content="Go to Page 2" HorizontalAlignment="Left" Margin="90,135,0,0" VerticalAlignment="Top" Height="59" Width="125" Click="btnGoPage2_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
string myString = "Welcome to ThaiCreate.Com";
Windows.Storage.StorageFolder roamingFolder = Windows.Storage.ApplicationData.Current.RoamingFolder;
Windows.Storage.StorageFile sampleFile = await roamingFolder.CreateFileAsync("dataFile.txt",
Windows.Storage.CreationCollisionOption.ReplaceExisting);
await Windows.Storage.FileIO.WriteTextAsync(sampleFile, myString);
this.lblStatus.Text = "Text file has been created.";
}
private void btnGoPage2_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2));
}
}
}
Page2.xaml
<Page
x:Class="WindowsStoreApps.Page2"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblResult" HorizontalAlignment="Left" Margin="93,69,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="30" Text="Result"/>
</Grid>
</Page>
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
Windows.Storage.StorageFolder roamingFolder = Windows.Storage.ApplicationData.Current.RoamingFolder;
Windows.Storage.StorageFile sampleFile = await roamingFolder.GetFileAsync("dataFile.txt");
String myString = await Windows.Storage.FileIO.ReadTextAsync(sampleFile);
this.lblResult.Text = myString;
}
}
}
Screenshot
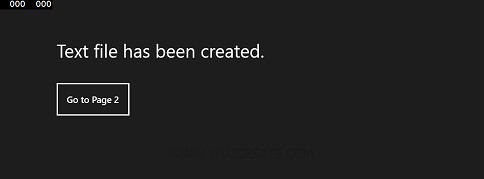
สร้าง Text file
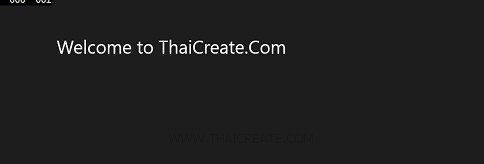
แสดงค่าใน Text file
อ่านเพิ่มเติม
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2014-03-17 12:17:18 /
2017-03-19 14:55:25 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|