การสร้าง Text file และการจัดเก็บบน Storage ของ Windows Store Apps (C#) |
การสร้าง Text file และการจัดเก็บบน Storage ของ Windows Store Apps (C#) สำหรับ Text file (.txt) เป็นรูปแบบการจัดเก็บข้อความหรือข้อมูลและเรียกใช้ที่ง่ายที่สุด สามารถจัดเก็บได้ในปริมาณมาก สามารถอ่านเขียนได้อย่างรวดเร็ว และการจัดเก็บลงใน Storage ก็ใช้ประมาณเนื้อที่น้อยอาจจะเรียกได้ว่าเท่ากับขนาด Size ของ ข้อความที่เก็บจริง ๆ และบน Windows Store Apps ก็สามารถที่จะเขียนโปรแกรมให้จัดเก็บข้อความในรูปแบบของ Text file ได้เช่นเดียวกัน สามารถจัดเก็บได้ทั้งใน Local data , Roaming data หรือ Temporary data ซึ่งรูปแบบการเขียนและอ่านก็จะไม่แตกต่างกัน มาลองดูตัวอย่างรูปแบบการเขียนและอ่าน Text file แบบง่าย ๆ จากบทความนี้
Syntax การเขียน Text file ลงใน Storage
string myString = this.txtName.Text;
Windows.Storage.StorageFolder localFolder = Windows.Storage.ApplicationData.Current.LocalFolder;
Windows.Storage.StorageFile sampleFile = await localFolder.CreateFileAsync("dataFile.txt",
Windows.Storage.CreationCollisionOption.ReplaceExisting);
await Windows.Storage.FileIO.WriteTextAsync(sampleFile, myString);
Syntax การอ่าน Text file จาก Storage
Windows.Storage.StorageFolder localFolder = Windows.Storage.ApplicationData.Current.LocalFolder;
Windows.Storage.StorageFile sampleFile = await localFolder.GetFileAsync("dataFile.txt");
String myString = await Windows.Storage.FileIO.ReadTextAsync(sampleFile);
this.lblResult.Text = myString;
ตัวอย่างการเขียน Apps เพื่อ เขียน Text file ไฟล์ ลงใน Storage บน Windows Store Apps
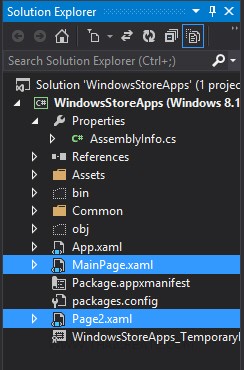
สร้าง Page ขึ้นมา 2 เพจ คือ MainPage.xaml (สำหรับเขียนลงใน Text file) และ Page2.xaml (สำหรับอ่าน Text file มาแสดง)
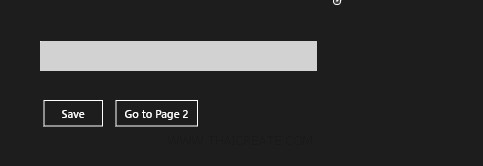
เพจของ MainPage.xaml
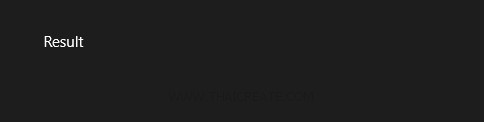
เพจของ Page2.xaml
Example 1 สร้าง Input เพื่อรับค่า Text และเขียนลง Storage จัดเก็บใน Local Data บน Windows Store Apps
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Button x:Name="btnSave" Content="Save" HorizontalAlignment="Left" Margin="94,203,0,0" VerticalAlignment="Top" Height="59" Width="125" FontSize="22" Click="btnSave_Click"/>
<Button x:Name="btnGoPage2" Content="Go to Page 2" HorizontalAlignment="Left" Margin="238,203,0,0" VerticalAlignment="Top" Height="59" Width="171" Click="btnGoPage2_Click" FontSize="22"/>
<TextBox x:Name="txtName" HorizontalAlignment="Left" Height="60" Margin="90,88,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="554" FontSize="36"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
}
private async void btnSave_Click(object sender, RoutedEventArgs e)
{
string myString = this.txtName.Text;
Windows.Storage.StorageFolder localFolder = Windows.Storage.ApplicationData.Current.LocalFolder;
Windows.Storage.StorageFile sampleFile = await localFolder.CreateFileAsync("dataFile.txt",
Windows.Storage.CreationCollisionOption.ReplaceExisting);
await Windows.Storage.FileIO.WriteTextAsync(sampleFile, myString);
}
private void btnGoPage2_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2));
}
}
}
Page2.xaml
<Page
x:Class="WindowsStoreApps.Page2"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblResult" HorizontalAlignment="Left" Margin="93,69,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="30" Text="Result"/>
</Grid>
</Page>
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
Windows.Storage.StorageFolder localFolder = Windows.Storage.ApplicationData.Current.LocalFolder;
Windows.Storage.StorageFile sampleFile = await localFolder.GetFileAsync("dataFile.txt");
String myString = await Windows.Storage.FileIO.ReadTextAsync(sampleFile);
this.lblResult.Text = myString;
}
}
}
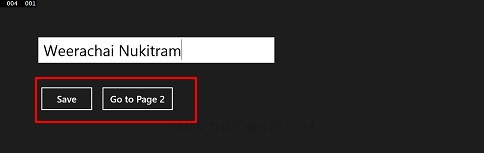
แสดง Input เพื่อรับค่า Text และ เขียน Text file ไฟล์ลงใน Storage ของ Windows Store Apps และให้คลิกที่ Go to Page 2 เพื่อดูไฟล์ที่ถูก Save ลงใน Storage
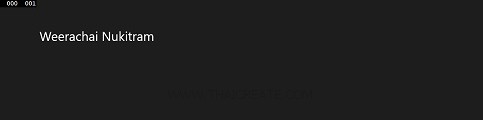
แสดงข้อความที่ถูกเขียนลงใน Text file ที่อยู่ใน Storage
สำหรับตัวอย่างแรกเป็นการจัดเก็บลงใน Local data แต่ในกรณีที่ต้องการจัดเก็บลงใน Roaming หรือ Temporary สามารถดูได้ต่อในตัวอย่างที่ 2 และ 3
Example 2 ทดสอบการจัดเก็บลงใน Roaming (RoamingFolder)
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
}
private async void btnSave_Click(object sender, RoutedEventArgs e)
{
string myString = this.txtName.Text;
Windows.Storage.StorageFolder roamingFolder = Windows.Storage.ApplicationData.Current.RoamingFolder;
Windows.Storage.StorageFile sampleFile = await roamingFolder.CreateFileAsync("dataFile.txt",
Windows.Storage.CreationCollisionOption.ReplaceExisting);
await Windows.Storage.FileIO.WriteTextAsync(sampleFile, myString);
}
private void btnGoPage2_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2));
}
}
}
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
Windows.Storage.StorageFolder roamingFolder = Windows.Storage.ApplicationData.Current.RoamingFolder;
Windows.Storage.StorageFile sampleFile = await roamingFolder.GetFileAsync("dataFile.txt");
String myString = await Windows.Storage.FileIO.ReadTextAsync(sampleFile);
this.lblResult.Text = myString;
}
}
}
Example 3 ทดสอบการจัดเก็บลงใน Temporary (TemporaryFolder)
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
}
private async void btnSave_Click(object sender, RoutedEventArgs e)
{
string myString = this.txtName.Text;
Windows.Storage.StorageFolder temporaryFolder = Windows.Storage.ApplicationData.Current.TemporaryFolder;
Windows.Storage.StorageFile sampleFile = await temporaryFolder.CreateFileAsync("dataFile.txt",
Windows.Storage.CreationCollisionOption.ReplaceExisting);
await Windows.Storage.FileIO.WriteTextAsync(sampleFile, myString);
}
private void btnGoPage2_Click(object sender, RoutedEventArgs e)
{
this.Frame.Navigate(typeof(Page2));
}
}
}
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class Page2 : Page
{
public Page2()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
Windows.Storage.StorageFolder temporaryFolder = Windows.Storage.ApplicationData.Current.TemporaryFolder;
Windows.Storage.StorageFile sampleFile = await temporaryFolder.GetFileAsync("dataFile.txt");
String myString = await Windows.Storage.FileIO.ReadTextAsync(sampleFile);
this.lblResult.Text = myString;
}
}
}
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2014-03-17 12:19:05 /
2017-03-19 14:53:33 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|