Windows Store Apps การอ่าน Text file และ CSV และการ Data Binding(C#) |
Windows Store Apps การอ่าน Text file และ CSV และการ Data Binding(C#) บทความนี้จะเป็นตัวอย่างการเขียน Windows Store Apps เพื่ออ่าน Text file ที่ได้จากการ Browse File ที่อยู่บน Storage โดยนำผลลัพธ์ที่ได้จากการอ่านมาแสดงบนหน้าจอของ Windows Store Apps และตัวอย่างที่สอง การอ่านไฟล์ CSV ที่อยู่ในรูปแบบของ Delimiter-separated เพื่อนำข้อมูลที่ได้จาก CSV มาทำการ Binding บน Control เช่น ListView
Syntax การอ่าน Text file
FileOpenPicker openPicker = new FileOpenPicker();
openPicker.ViewMode = PickerViewMode.List;
openPicker.SuggestedStartLocation = PickerLocationId.DocumentsLibrary;
openPicker.FileTypeFilter.Add(".txt");
StorageFile txtFile = await openPicker.PickSingleFileAsync();
String myString = await Windows.Storage.FileIO.ReadTextAsync(txtFile);
Syntax การอ่าน CSV file และการทำ Data Binding
FileOpenPicker openPicker = new FileOpenPicker();
openPicker.ViewMode = PickerViewMode.List;
openPicker.SuggestedStartLocation = PickerLocationId.DocumentsLibrary;
openPicker.FileTypeFilter.Add(".csv");
StorageFile csvFile = await openPicker.PickSingleFileAsync();
if (csvFile != null)
{
String myString = await Windows.Storage.FileIO.ReadTextAsync(csvFile);
List<myCustomer> ls = new List<myCustomer>();
string[] strText = myString.Split('\n');
foreach (string str in strText)
{
string[] strLine = str.Split(',');
ls.Add(new myCustomer
{
CustomerID = strLine[0],
Name = strLine[1],
Email = strLine[2],
CountryCode = strLine[3],
Budget = strLine[4],
Used = strLine[5]
});
}
this.myListView.ItemsSource = ls;
}
Example 1 การสร้าง File Picker สำหรับทำการ Browse เพื่อเลือก Text file และการแสดงข้อความของ Text file
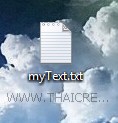
Text file ที่อยู่บน Desktop
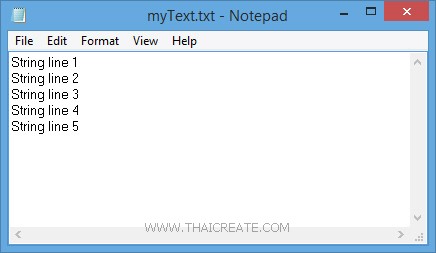
รายละเอียดไฟล์
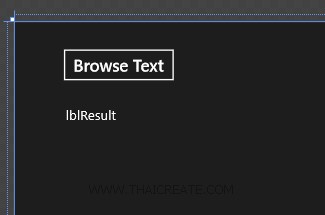
ออกแบบหน้าจอปุ่ม Button เพื่อจะทำการ Browse เลือกไฟล์ และมี TextBlock สำหรับแสดง Result ที่ได้จาก Text file
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<TextBlock x:Name="lblResult" HorizontalAlignment="Left" Margin="66,83,0,0" TextWrapping="Wrap" VerticalAlignment="Top" FontSize="20" Text="lblResult"/>
<Button x:Name="btnBrowse" Content="Browse PDF" HorizontalAlignment="Left" Margin="70,38,0,0" VerticalAlignment="Top" FontSize="25" Click="btnBrowse_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using Windows.Storage;
using Windows.Storage.Pickers;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private async void btnBrowse_Click(object sender, RoutedEventArgs e)
{
FileOpenPicker openPicker = new FileOpenPicker();
openPicker.ViewMode = PickerViewMode.List;
openPicker.SuggestedStartLocation = PickerLocationId.DocumentsLibrary;
openPicker.FileTypeFilter.Add(".txt");
StorageFile txtFile = await openPicker.PickSingleFileAsync();
if(txtFile != null)
{
String myString = await Windows.Storage.FileIO.ReadTextAsync(txtFile);
// use myString
this.lblResult.Text = string.Empty;
int i = 0;
string[] strText = myString.Split('\n');
foreach (string str in strText)
{
i++;
this.lblResult.Text = this.lblResult.Text + i + ". " +str;
this.lblResult.Text = this.lblResult.Text + Environment.NewLine;
}
}
}
}
}
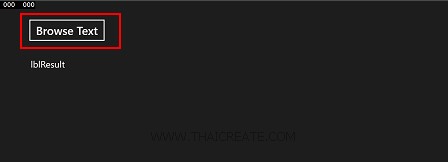
คลิกที่ Button เลือก Browse ไฟล์
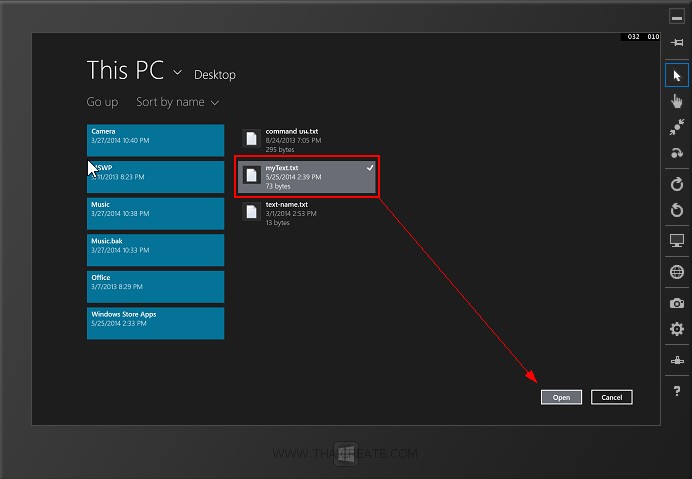
เลือก Text file ที่อยู่บน Desktop
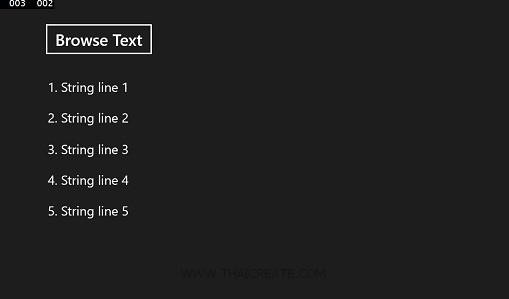
แสดง Text file บนหน้าจอของ Windows Store Apps
Example 2 การสร้าง File Picker สำหรับทำการ Browse เพื่อเลือก CSV file และทำการ Binding Data บน ListView

customer.csv
C001,Win Weerachai,[email protected],TH,1000000,600000
C002,John Smith,[email protected],UK,2000000,800000
C003,Jame Born,[email protected],US,3000000,600000
C004,Chalee Angel,[email protected],US,4000000,100000
ตัวอย่างไฟล์ CSV ที่จะทำการทดสอบ ซึ่งได้จัดเก็บไว้บน Desktop
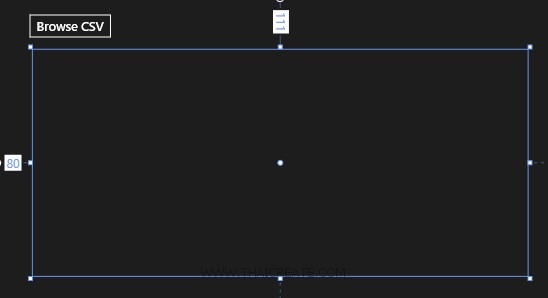
ออกแบบหน้าขอของ Windows Store Apps ด้วย Button และ ListView
MainPage.xaml.cs
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Button x:Name="btnBrowse" Content="Browse CSV" HorizontalAlignment="Left" Margin="70,38,0,0" VerticalAlignment="Top" FontSize="25" Click="btnBrowse_Click"/>
<ListView x:Name="myListView" HorizontalAlignment="Left" Height="453" Margin="80,111,0,0" VerticalAlignment="Top" Width="989">
<ListView.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal" Margin="0,0,0,17">
<StackPanel Width="80">
<TextBlock Text="{Binding CustomerID}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0" FontFamily="Global User Interface"/>
</StackPanel>
<StackPanel Width="150">
<TextBlock Text="{Binding Name}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="300">
<TextBlock Text="{Binding Email}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="50">
<TextBlock Text="{Binding CountryCode}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Budget}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Used}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
</StackPanel>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
</Grid>
</Page>
Page2.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using Windows.Storage;
using Windows.Storage.Pickers;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
public class myCustomer
{
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
private async void btnBrowse_Click(object sender, RoutedEventArgs e)
{
FileOpenPicker openPicker = new FileOpenPicker();
openPicker.ViewMode = PickerViewMode.List;
openPicker.SuggestedStartLocation = PickerLocationId.DocumentsLibrary;
openPicker.FileTypeFilter.Add(".csv");
StorageFile csvFile = await openPicker.PickSingleFileAsync();
if (csvFile != null)
{
String myString = await Windows.Storage.FileIO.ReadTextAsync(csvFile);
List<myCustomer> ls = new List<myCustomer>();
string[] strText = myString.Split('\n');
foreach (string str in strText)
{
string[] strLine = str.Split(',');
ls.Add(new myCustomer
{
CustomerID = strLine[0],
Name = strLine[1],
Email = strLine[2],
CountryCode = strLine[3],
Budget = strLine[4],
Used = strLine[5]
});
}
this.myListView.ItemsSource = ls;
}
}
}
}
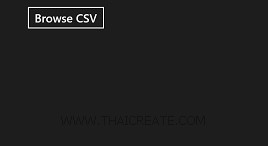
คลิกที่ Browse เพื่อเลือก CSV
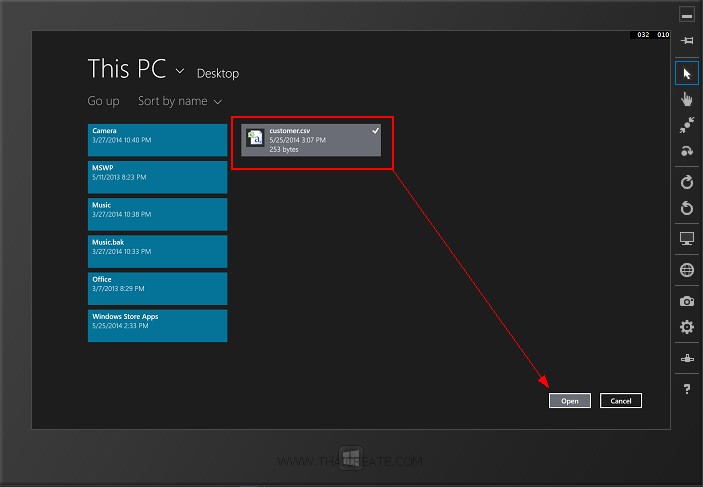
เลือกไฟล์ CSV ที่อยู่บน Desktop
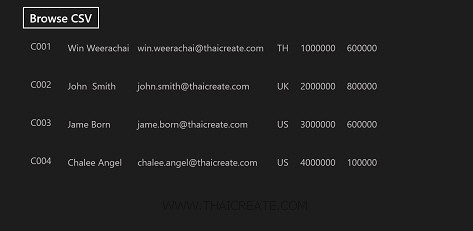
แสดงข้อมูลจาก CSV ซึ่งถูก Binding บน ListView
.
|