Windows Store Apps and Video Capture from Camera (C#) |
Windows Store Apps and Video Capture from Camera (C#) บทความนี้จะเป็นตัวอย่างการเขียน Windows Store Apps ด้วยภาษา C# ทำงานร่วมกับ XAML โดยจะยกตัวอย่างการเขียน Apps เพื่อเรียกใช้งาน Camera (กล้อง) ที่อยู่บนอุปกรณ์ที่ติดตั้ง Apps ด้วยการเรียกกล้องขึ้นมาแสดง พร้อมกับอัดเป็นคลิป Video และหลังจากที่ได้เป็นไฟล์ Video ก็ให้นำไฟล์นั้นมาเล่นบนหน้าจอ Apps พร้อม ๆ กับการขัดเก็บไฟล์ที่ได้ลงใน Storage
ขั้นตอนการเรียกใช้ CameraCaptureUI เพื่อ Capture Video
เรียกใช้งาน Class ของ Windows.Media.Capture และ Windows.Graphics.Display
using Windows.Media.Capture;
using Windows.Graphics.Display;
ทำการเปิดกล้องโดยเรียก CameraCaptureUI
CameraCaptureUI videocamera = new CameraCaptureUI();
[cs]กำหนดคุณสมบัติของ Format
[cs]videocamera.VideoSettings.Format = CameraCaptureUIVideoFormat.Mp4;
videocamera.VideoSettings.AllowTrimming = true;
videocamera.VideoSettings.MaxDurationInSeconds = 30;
videocamera.VideoSettings.MaxResolution = CameraCaptureUIMaxVideoResolution.HighestAvailable;
[cs]สิ่งที่ได้กลับมาคือ StorageFile
[cs]StorageFile video = await videocamera.CaptureFileAsync(CameraCaptureUIMode.Video);
สามารถแปลงเป็นในรูปแบบของ Streaming เพื่อแสดงผลบน Control ของ MediaElement
IRandomAccessStream stream = await video.OpenAsync(FileAccessMode.Read);
myMedia.SetSource(stream, "video/mp4");
myMedia.Visibility = Visibility.Visible;
Example 1 ตัวอย่างการเขียน Windows Store Apps เพื่อทำการ Capture Video จากกล้อง Camera
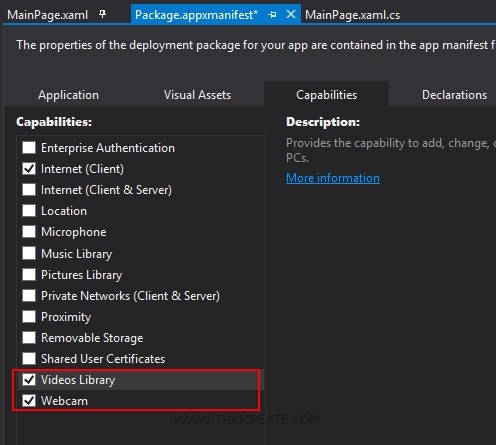
กรณีที่มีการใช้กล้องจะต้อง Allow ในส่วนของ Webcam และ Video Library
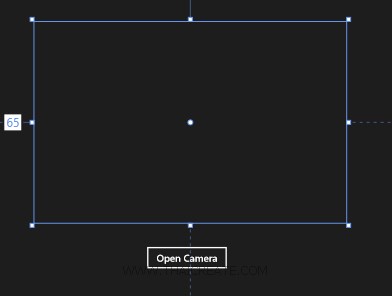
ออกแบบหน้าจอประกอบด้วย Control ของ MediaElement และ Button
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
xmlns:bm="using:Bing.Maps">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<MediaElement x:Name="myMedia" HorizontalAlignment="Left" Height="300" Margin="65,46,0,0" VerticalAlignment="Top" Width="465"/>
<Button x:Name="btnCamera" Content="Open Camera" HorizontalAlignment="Left" Margin="230,379,0,0" VerticalAlignment="Top" Click="btnCamera_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using Windows.Media.Capture;
using Windows.Graphics.Display;
using Windows.UI.Xaml.Media.Imaging;
using Windows.Storage;
using Windows.Storage.Streams;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private async void btnCamera_Click(object sender, RoutedEventArgs e)
{
CameraCaptureUI videocamera = new CameraCaptureUI();
videocamera.VideoSettings.Format = CameraCaptureUIVideoFormat.Mp4;
videocamera.VideoSettings.AllowTrimming = true;
videocamera.VideoSettings.MaxDurationInSeconds = 30;
videocamera.VideoSettings.MaxResolution = CameraCaptureUIMaxVideoResolution.HighestAvailable;
StorageFile video = await videocamera.CaptureFileAsync(CameraCaptureUIMode.Video);
if (video != null)
{
IRandomAccessStream stream = await video.OpenAsync(FileAccessMode.Read);
myMedia.SetSource(stream, "video/mp4");
myMedia.Visibility = Visibility.Visible;
}
}
}
}
Result
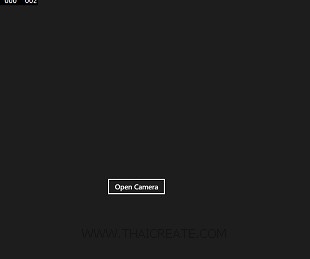
ทดสอบการทำงาน ให้คลิก Open Camera
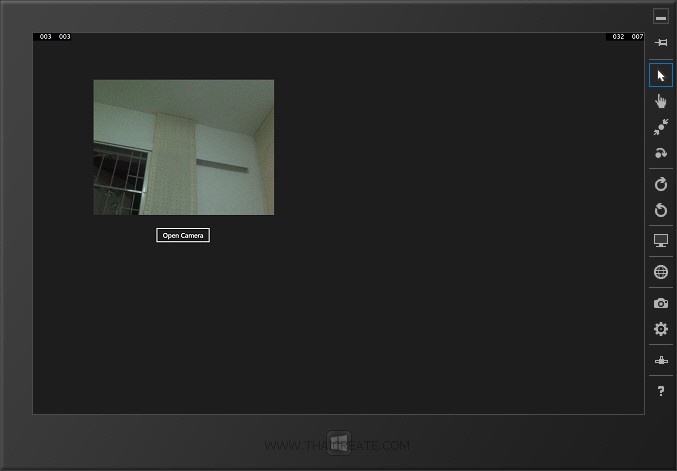
ขั้นตอนนี้จะเป็นการ Capture เป็นไฟล์ Video จากนั้นเราจะสามารถนำไฟล์นั้นกลับมาเล่นบน MediaElement ได้
ในกรณีที่ต้องการให้จัดเก็บลงใน Storage ให้เพิ่มคำสั่งนี้เข้าไป
var targetFile = await ApplicationData.Current.LocalFolder.CreateFileAsync("myVideo.mp4");
await video.MoveAndReplaceAsync(targetFile);
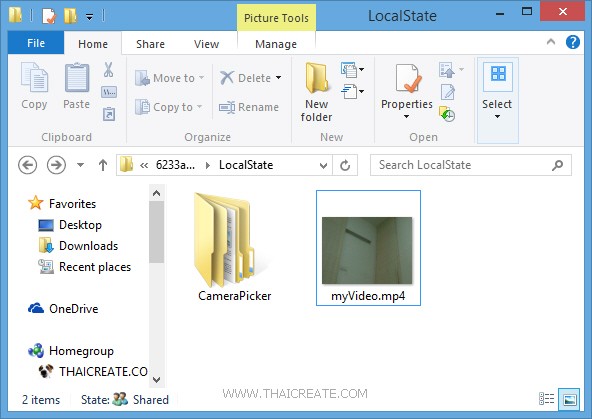
โปรแกรมก็จะนำไฟล์ที่ได้จัดเก็บลงใน Storage
Code เต็ม ๆ
private async void btnCamera_Click(object sender, RoutedEventArgs e)
{
CameraCaptureUI videocamera = new CameraCaptureUI();
videocamera.VideoSettings.Format = CameraCaptureUIVideoFormat.Mp4;
videocamera.VideoSettings.AllowTrimming = true;
videocamera.VideoSettings.MaxDurationInSeconds = 30;
videocamera.VideoSettings.MaxResolution = CameraCaptureUIMaxVideoResolution.HighestAvailable;
StorageFile video = await videocamera.CaptureFileAsync(CameraCaptureUIMode.Video);
if (video != null)
{
IRandomAccessStream stream = await video.OpenAsync(FileAccessMode.Read);
myMedia.SetSource(stream, "video/mp4");
myMedia.Visibility = Visibility.Visible;
var targetFile = await ApplicationData.Current.LocalFolder.CreateFileAsync("myVideo.mp4");
await video.MoveAndReplaceAsync(targetFile);
}
}
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2014-06-23 13:18:26 /
2017-03-19 15:02:21 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|