WebView แสดงผล URL เว็บไซต์ หรือ HTML บน Windows Store Apps (C#) |
WebView แสดงผล URL เว็บไซต์ หรือ HTML บน Windows Store Apps (C#) สำหรับ WebView บน Windows Store Apps ใช้สำหรับการแสดงข้อมูล URL (Website) บนหน้าจอ Apps ของ Windows Store Application โดย WebView เป็น Controls ที่ใช้งานง่าย เพียงแค่ระบุ URL ก็สามารถแสดงผลได้ทันที สามารถแสดงผลได้ทั้ง URL และ HTML ในรูปแบบของ String หรือ ไฟล์ HTML ก็ได้เช่นเดียวกัน
Example 1 แสดงผล URL ของเว็บไซต์ด้วย WebView แบบง่าย ๆ
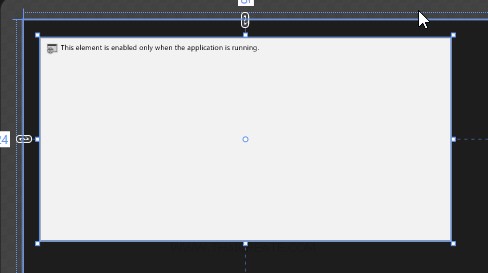
ลาก Control ของ WebView ไว้บนหน้าจอ Page
<WebView x:Name="myWebView" Source="https://www.thaicreate.com" />
Code เต็ม ๆ
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<WebView x:Name="myWebView" HorizontalAlignment="Left" Source="https://www.thaicreate.com" Height="304" Margin="24,26,0,0" VerticalAlignment="Top" Width="613"/>
</Grid>
</Page>
Screenshot
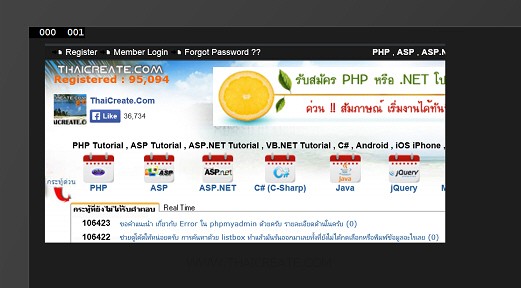
แสดงผล URL บน WebView
Example 2 สร้าง TextBox สำหรับกรอก URL และปุ่ม Button สำหรับ เปิดเว็บไซต์
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<WebView x:Name="myWebView" HorizontalAlignment="Left" Height="304" Margin="33,68,0,0" VerticalAlignment="Top" Width="613"/>
<TextBox x:Name="txtURL" HorizontalAlignment="Left" Height="27" Margin="32,26,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="535"/>
<Button x:Name="btnGo" Content="Go !!" HorizontalAlignment="Left" Margin="582,22,0,0" VerticalAlignment="Top" Click="btnGo_Click"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private void btnGo_Click(object sender, RoutedEventArgs e)
{
this.myWebView.Navigate(new Uri(this.txtURL.Text, UriKind.Absolute));
}
}
}
Screenshot
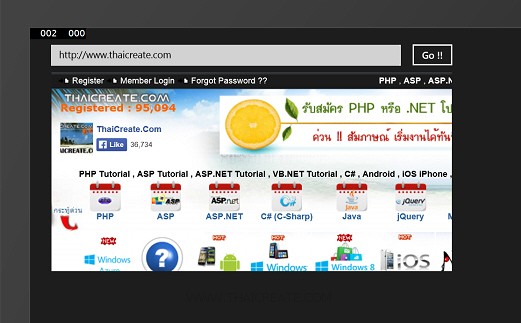
สามารถกรอก URL และแสดงผลเว็บไซต์ของ URL นั้่น ๆ
Example 3 แสดงผล HTML จากไฟล์ที่สร้างไว้ใน Resource
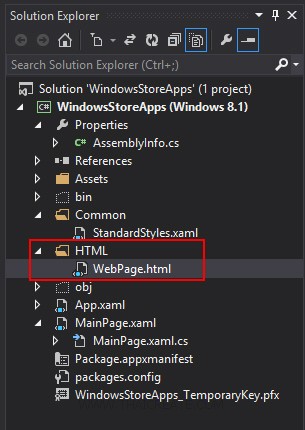
สร้างไฟล์ HTML
HTML/WebPage.html
<html>
<head>
<title>Sample Readme File</title>
</head>
<body>
<p>Sample Readme Content</p>
</body>
</html>
ไฟล์ HTML
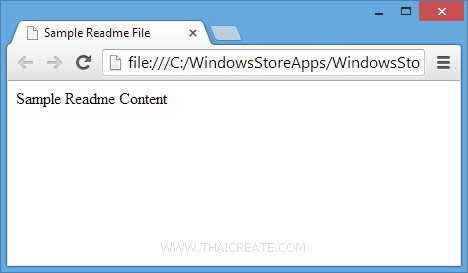
ทดสอบเรียกไฟล์ HTML ซึ่งเราจะแสดงผลบน WebView
var html = await Windows.Storage.PathIO.ReadTextAsync("ms-appx:///HTML/WebPage.html");
this.myWebView.NavigateToString(html);
Code เต็ม ๆ
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<WebView x:Name="myWebView" HorizontalAlignment="Left" Source="https://www.thaicreate.com" Height="304" Margin="24,26,0,0" VerticalAlignment="Top" Width="613"/>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
var html = await Windows.Storage.PathIO.ReadTextAsync("ms-appx:///HTML/WebPage.html");
this.myWebView.NavigateToString(html);
}
}
}
Screenshot
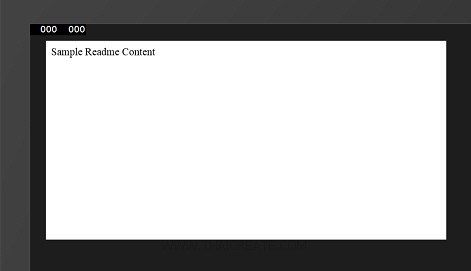
แสดงผล HTML บน WebView
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
 |
|
|
Create/Update Date : |
2014-03-02 10:46:50 /
2017-03-19 14:44:21 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|