Windows Store App and XML Parser / Feed from PHP & MySQL (C#) |
Windows Store App and XML Parser / Feed from PHP & MySQL (C#) การเขียน Windows Store Apps กับ XML ไฟล์ การ Feed ข้อมูลจาก XML เชื่อมต่อกับ HTTP ในรูปแบบของ URL ทำงานบน Web Server ในฝั่งของ Web Server ที่ถูกเรียกผ่าน URL จะถูกทำงานด้วยภาษา PHP กับ MySQL Database โดยหน้าที่ของ PHP คืออ่านข้อมูลจาก MySQL Database จากนั้นแปลงให้อยู่ในรูปแบบของ XML ไฟล์ ซึ่งเมื่อได้ XML แล้ว Windows Store Apps ก็จะเรียกผ่าน URL ส่งค่า Result เพื่อแสดงผลบนหน้าจอของ Windows Store Apps
ฝั่ง Web Server (PHP/MySQL)
MySQL Table บน Web Server

CREATE TABLE `customer` (
`CustomerID` varchar(4) NOT NULL,
`Name` varchar(50) NOT NULL,
`Email` varchar(50) NOT NULL,
`CountryCode` varchar(2) NOT NULL,
`Budget` double NOT NULL,
`Used` double NOT NULL,
PRIMARY KEY (`CustomerID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
INSERT INTO `customer` VALUES ('C001', 'Win Weerachai', '[email protected]', 'TH', 1000000, 600000);
INSERT INTO `customer` VALUES ('C002', 'John Smith', '[email protected]', 'UK', 2000000, 800000);
INSERT INTO `customer` VALUES ('C003', 'Jame Born', '[email protected]', 'US', 3000000, 600000);
INSERT INTO `customer` VALUES ('C004', 'Chalee Angel', '[email protected]', 'US', 4000000, 100000);
PHP ที่ทำหน้าที่อ่านข้อมูล MySQL Database และแปลงเป็น XML
getXML.php
<?php
header("Content-type:text/xml; charset=UTF-8");
header("Cache-Control: no-store, no-cache, must-revalidate");
header("Cache-Control: post-check=0, pre-check=0", false);
echo '<?xml version="1.0" encoding="utf-8"?>';
$objConnect = mysql_connect("localhost","root","root");
$objDB = mysql_select_db("mydatabase");
$strSQL = "SELECT * FROM customer WHERE 1 ";
$objQuery = mysql_query($strSQL);
?>
<customers>
<?php
while($obResult = mysql_fetch_array($objQuery))
{
?>
<customer>
<CustomerID><?php echo $obResult["CustomerID"];?></CustomerID>
<Name><?php echo $obResult["Name"];?></Name>
<Email><?php echo $obResult["Email"];?></Email>
<CountryCode><?php echo $obResult["CountryCode"];?></CountryCode>
<Budget><?php echo $obResult["Budget"];?></Budget>
<Used><?php echo $obResult["Used"];?></Used>
</customer>
<?php
}
?>
</customers>
<?php
mysql_close($objConnect);
?>
เมื่อทดสอบเรียก PHP เราจะได้ Result ที่เป็น XML ดังนี้
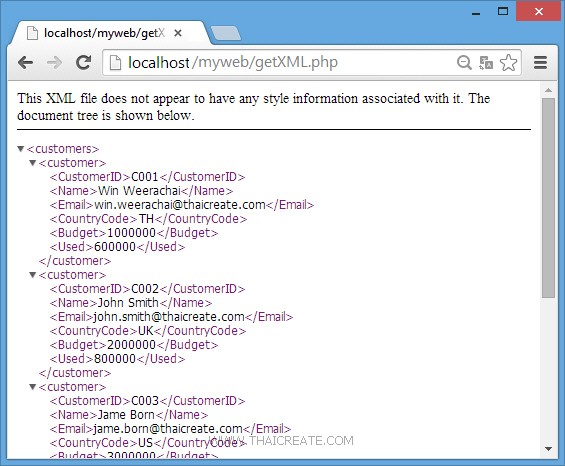
ฝั่ง Windows Store Apps (C#)
สร้าง Class สำหรับ Mapping Column
public class myCustomer
{
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
ดึงค่า Result จาก Web Server ซึ่งจะได้ค่าเป็น XML กลับมา
string URL = "http://localhost/myweb/getXML.php";
var xDoc = XDocument.Load(URL);
การอ่าน XML และ Mapping กับ Column ในรูปแบบของ List Object ซึ่งสามารถใช้ Linq ได้
var data = from query in xDoc.Descendants("customer")
select new myCustomer()
{
CustomerID = (string)query.Element("CustomerID"),
Name = (string)query.Element("Name"),
Email = (string)query.Element("Email"),
CountryCode = (string)query.Element("CountryCode"),
Budget = (string)query.Element("Budget"),
Used = (string)query.Element("Used")
};
สามารถทำ List ที่ได้ไปแสดงผลบน Data Source ได้
this.myListView.ItemsSource = data;
ลองมาดูตัวอย่างแบบเต็ม ๆ
Example การเขียน Windows Store Apps อ่าน XML ไฟล์ มาแสดงบนหน้าจอ ListView ของ Windows Store Apps
MainPage.xaml
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<ListView x:Name="myListView" HorizontalAlignment="Left" Height="453" Margin="58,98,0,0" VerticalAlignment="Top" Width="989">
<ListView.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal" Margin="0,0,0,17">
<StackPanel Width="80">
<TextBlock Text="{Binding CustomerID}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0" FontFamily="Global User Interface"/>
</StackPanel>
<StackPanel Width="150">
<TextBlock Text="{Binding Name}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="300">
<TextBlock Text="{Binding Email}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="50">
<TextBlock Text="{Binding CountryCode}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Budget}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
<StackPanel Width="100">
<TextBlock Text="{Binding Used}" TextWrapping="Wrap" FontSize="20" Foreground="#FFBFB9B9" Margin="5,0,0,0"/>
</StackPanel>
</StackPanel>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
</Grid>
</Page>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.Xml.Linq;
using Windows.Data.Xml.Dom;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
public class myCustomer
{
public string CustomerID { get; set; }
public string Name { get; set; }
public string Email { get; set; }
public string CountryCode { get; set; }
public string Budget { get; set; }
public string Used { get; set; }
}
protected override void OnNavigatedTo(NavigationEventArgs e)
{
string URL = "http://localhost/myweb/getXML.php";
var xDoc = XDocument.Load(URL);
var data = from query in xDoc.Descendants("customer")
select new myCustomer()
{
CustomerID = (string)query.Element("CustomerID"),
Name = (string)query.Element("Name"),
Email = (string)query.Element("Email"),
CountryCode = (string)query.Element("CountryCode"),
Budget = (string)query.Element("Budget"),
Used = (string)query.Element("Used")
};
this.myListView.ItemsSource = data;
}
}
}
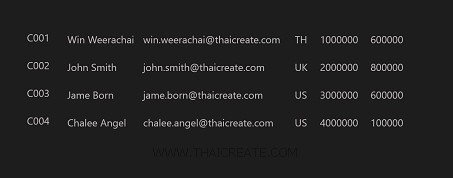
ชุดข้อมูลที่ได้จาก XML ไฟล์ ซึ่งนำไป Binding Data บน ListView
.
|