Windows Store Apps and Youtube API (C#) |
Windows Store Apps and Youtube API (C#) บทความนี้จะเป็นตัวอย่างการเขียน Windows Store Apps เพื่อเล่นไฟล์ Youtbe ในรูปแบบของ API คือการดึง Playlist จาก Youtbe มาแสดงบนหน้าจอ Apps ด้วยการแสดงรูป Thumbnail พร้อมกับชื่อ Clip จากนั้นเมื่อทำการคลิกที่ Clip นั้น ๆ ก็จะเป็นการเล่นคลิปบน MediaElement
Windows Store Apps and Youtube API (C#)
ตามแนวคิดการเขียน Apps รูปแบบใหม่ Apps ที่ถูกติดตั้งลงบน Client ของ User ในกรณีที่มี Feature ใหม่ ๆ เราจะต้องส่งตัว Update ให้กับ User ทำการ Download และ Update ใหม่ซึ่งอาจจะเสียเวลาในการใช้งาน ฉะนั้นทางที่ดีการพัฒนา Apps ควรจะมี Web Server ที่ทำหน้าที่เป็นตัวกลาง คอยส่งข้อมูลต่าง ๆ ให้กับ Client ฉะนั่นจึงมั่นใจได้ว่า Apps ที่ถูกติดตั้งตาม Client ต่าง ๆ จะมีการ Update ข้อมูลจาก Server ตลอดเวลา
และวิธีที่ได้รับความนิยมและใช้งานง่ายก็คงจะไม่พ้น HTTP ใช้วิธีการการออกแบบ URL ให้ Apps เรียกใช้งาน โดยฝั่ง Web Server อาจจะเขียนด้วย PHP หรือ ASP.Net ส่วนรูปแบบการรับ-ส่งข้อมูลนั้นจะใช้ JSON เป็นตัวกลางในการส่ง
getPlaylist.php
<?php
function getYouTubeIdFromURL($url)
{
$url_string = parse_url($url, PHP_URL_QUERY);
parse_str($url_string, $args);
return isset($args['v']) ? $args['v'] : false;
}
$playlist_id = "LLEPTp5WMAzjh9mOrKUwRLmQ";
$url = "http://gdata.youtube.com/feeds/api/playlists/".$playlist_id."?v=2&alt=json";
$data = json_decode(file_get_contents($url),true);
$info = $data["feed"];
$video = $info["entry"];
$nVideo = count($video);
$resultArray = array();
for($i=0;$i<$nVideo;$i++){
$myVideo["Name"] = $video[$i]['title']['$t'];
$myVideo["YoutubeID"] = getYouTubeIdFromURL($video[$i]['link'][0]['href']);
$myVideo["Image"] = $video[$i]['media$group']['media$thumbnail'][1]['url'];
array_push($resultArray,$myVideo);
}
echo json_encode($resultArray);
?>
จาก Code ของ PHP จะเป็นการเขียน PHP เพื่อดึง Playlist ของ Youtube
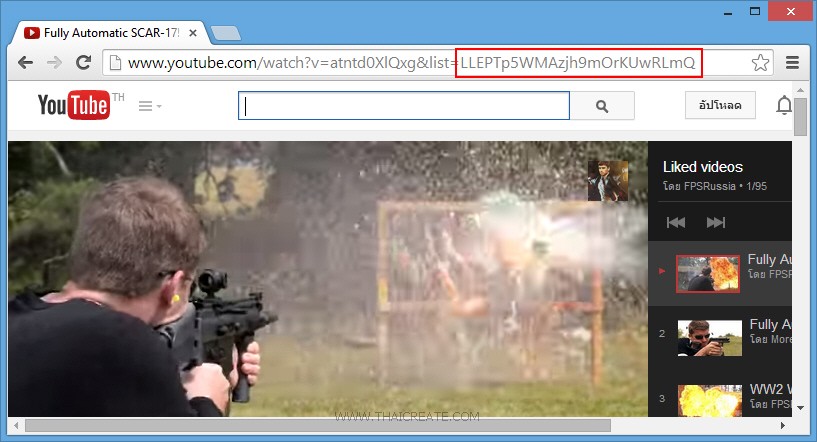
ตัวอย่างการดึง Playlist จาก Youtube ด้วย PHP

ซึ่งเราจะได้ค่าเป็น JSON กลับมา

จากนั้นเราจะใช้ MyToolkit เป็น Class สำหรับเล่นไฟล์ Youtube สามารถ Add ผ่าน NuGet Package ได้
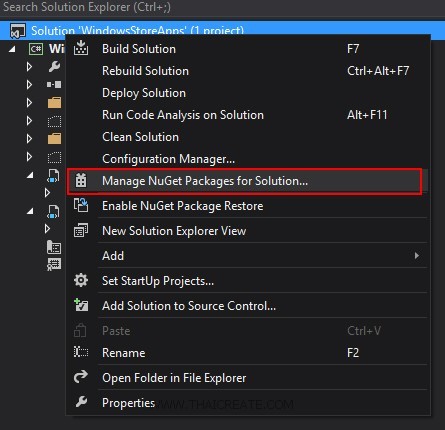
คลิกขวาที่ Reference เลือก Manage NuGet Package
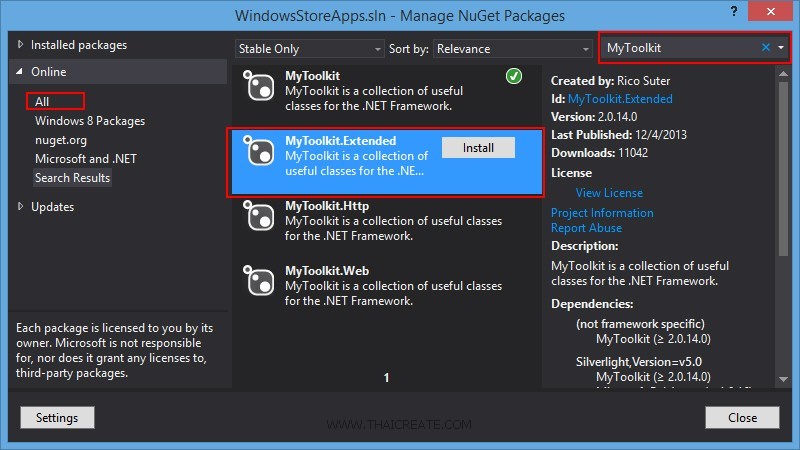
ค้นหา Package ที่มีชื่อว่า MyToolkit.Extended
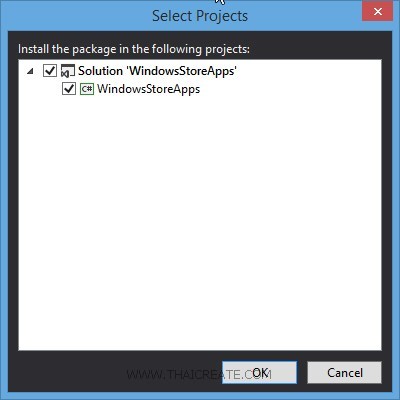
เลือก Install ลงใน Project
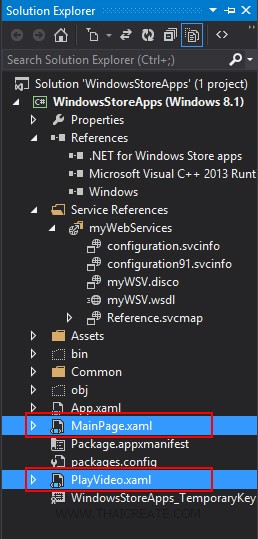
สร้างไฟล์ ขึ้นมา 2 ไฟล์คือ MainPage.xaml (สำหรับแสดง Playlist) , PlayVideo.xaml (สำหรับเล่น Video)
MainPage.xaml เป็น Page สำหรัยแสดง Playlist
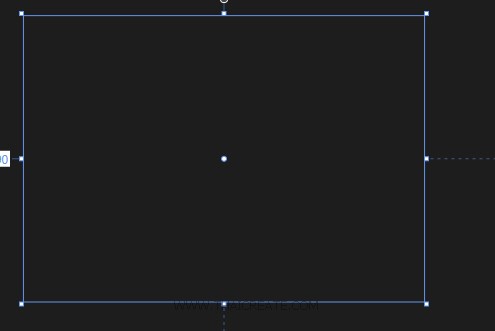
ออกแบบหน้าจอดังรูป
<Page
x:Class="WindowsStoreApps.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<GridView x:Name="myGridView" HorizontalAlignment="Left" Margin="90,42,0,0" VerticalAlignment="Top" Width="800" Height="571" SelectionChanged="myGridView_SelectionChanged">
<GridView.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal" Margin="0,0,0,17">
<Image Height="100" Width="100" Source="{Binding Path=ImagePath}" Margin="12,0,9,0"/>
<StackPanel Width="400">
<TextBlock Text="{Binding Name}" TextWrapping="Wrap" FontSize="20" Margin="12,-6,12,0" />
<TextBlock Text="{Binding YoutubeID}" TextWrapping="Wrap" FontSize="20" Margin="12,-6,12,0"/>
</StackPanel>
</StackPanel>
</DataTemplate>
</GridView.ItemTemplate>
</GridView>
</Grid>
</Page>
MainPage.xam.csl
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using Windows.Devices.Geolocation;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Core;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using System.Net.Http;
using System.Xml.Linq;
using System.Runtime.Serialization;
using System.Runtime.Serialization.Json;
using System.Collections.ObjectModel;
using System.Text;
using Windows.UI.Xaml.Media.Imaging;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
///
public class YoutubeList
{
public string Name { get; set; }
public string YoutubeID { get; set; }
public string Image { get; set; }
public BitmapImage ImagePath { get; set; }
}
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
protected BitmapImage getImageSource(string url)
{
try
{
BitmapImage bm = new BitmapImage(new Uri(url, UriKind.Absolute));
return bm;
}
catch (Exception ex)
{
return null;
}
}
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
HttpClient http = new System.Net.Http.HttpClient();
HttpResponseMessage response = await http.GetAsync("http://localhost/myweb/getPlaylist.php");
response.EnsureSuccessStatusCode();
string resultJSON = string.Empty;
resultJSON = await response.Content.ReadAsStringAsync();
MemoryStream ms = new MemoryStream(Encoding.UTF8.GetBytes(resultJSON));
ObservableCollection<YoutubeList> list = new ObservableCollection<YoutubeList>();
DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(ObservableCollection<YoutubeList>));
list = (ObservableCollection<YoutubeList>)serializer.ReadObject(ms);
List<YoutubeList> ls = new List<YoutubeList>();
foreach (YoutubeList item in list)
{
ls.Add(new YoutubeList { Name = item.Name, YoutubeID = item.YoutubeID, Image = item.Image, ImagePath = getImageSource(item.Image) });
}
this.myGridView.ItemsSource = ls;
}
private void myGridView_SelectionChanged(object sender, SelectionChangedEventArgs e)
{
YoutubeList youtube = (sender as GridView).SelectedItem as YoutubeList;
this.Frame.Navigate(typeof(PlayVideo), youtube.YoutubeID.ToString());
}
}
}
PlayVideo.xaml เป็น Page สำหรับแสดงเล่นไฟล์ Youtube
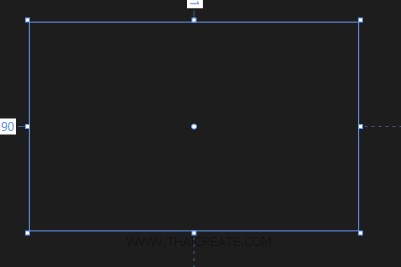
<Page
x:Class="WindowsStoreApps.PlayVideo"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:WindowsStoreApps"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<MediaElement x:Name="myMedia" HorizontalAlignment="Left" Height="416" Margin="90,91,0,0" VerticalAlignment="Top" Width="656"/>
</Grid>
</Page>
PlayVideo.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.InteropServices.WindowsRuntime;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using MyToolkit.Multimedia;
// The Blank Page item template is documented at http://go.microsoft.com/fwlink/?LinkId=234238
namespace WindowsStoreApps
{
/// <summary>
/// An empty page that can be used on its own or navigated to within a Frame.
/// </summary>
public sealed partial class PlayVideo : Page
{
public PlayVideo()
{
this.InitializeComponent();
}
string sYoutubeID = string.Empty;
protected async override void OnNavigatedTo(NavigationEventArgs e)
{
sYoutubeID = e.Parameter as string;
var url = await YouTube.GetVideoUriAsync(sYoutubeID, YouTubeQuality.Quality1080P);
this.myMedia.Source = url.Uri;
}
}
}
Result
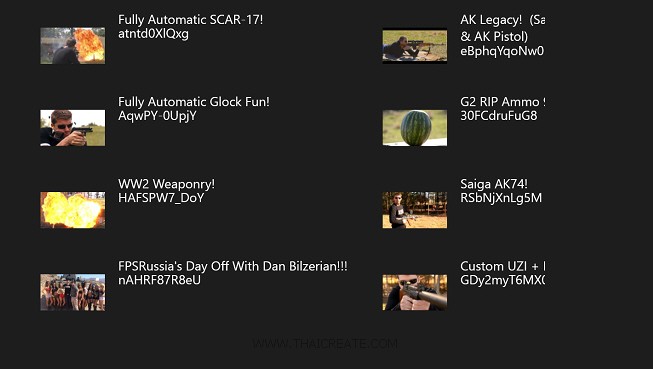
แสดง Playlist จาก Youtbe
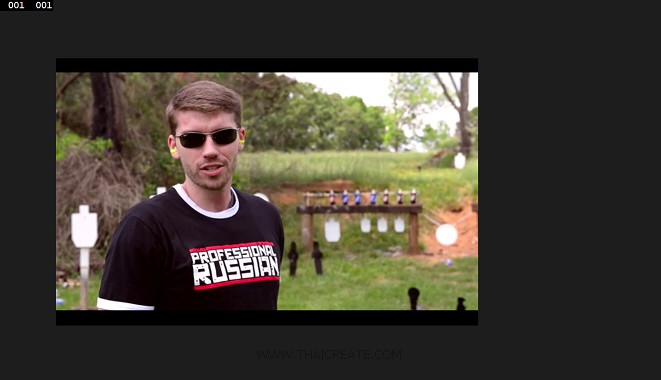
เล่นไฟล์ Youtube ที่ได้จากการคลิกเลือก
.
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
   |
|
|
Create/Update Date : |
2014-06-29 20:53:10 /
2017-03-19 15:01:00 |
|
Download : |
No files |
|
Sponsored Links / Related |
|
|
|
|
|
|
|