ASP.NET GridView Control - DropDownList in GridView |
ASP.NET GridView Control - DropDownList in GridView ตัวอย่างนี้จะเป็นการใช้งาน GridView และ DropDownList โดยจะทำการ BindData ลงใน Column ที่เป็น DropDownList โดยในตัวอย่างจะทำการ Bind ในส่วนของ Footer และในส่วนของ Edit Item
Language Code : VB.NET || C#
Framework : 2,3,4
GridViewDropDownList.aspx
<%@ Page Language="VB" %>
<%@ import Namespace="System.Data" %>
<%@ import Namespace="System.Data.OleDb" %>
<script runat="server">
Dim objConn As OleDbConnection
Dim objCmd As OleDbCommand
Dim strSQL As String
Sub Page_Load(sender As Object, e As EventArgs)
Dim strConnString As String
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source="& _
Server.MapPath("database/mydatabase.mdb")&";"
objConn = New OleDbConnection(strConnString)
objConn.Open()
IF Not Page.IsPostBack() Then
BindData()
End IF
End Sub
Sub BindData()
strSQL = "SELECT * FROM customer"
Dim dtReader As OleDbDataReader
objCmd = New OleDbCommand(strSQL, objConn)
dtReader = objCmd.ExecuteReader()
'*** BindData to GridView ***'
myGridView.DataSource = dtReader
myGridView.DataBind()
dtReader.Close()
dtReader = Nothing
End Sub
Sub Page_UnLoad()
objConn.Close()
objConn = Nothing
End Sub
Function DataTableCountryCode() As DataTable
Dim strConnString As String
Dim dtAdapter As OleDbDataAdapter
Dim dt As New DataTable
strConnString = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source="& _
Server.MapPath("database/mydatabase.mdb")&";"
objConn = New OleDbConnection(strConnString)
objConn.Open()
Dim strSQL As String
strSQL = "SELECT * FROM country"
dtAdapter = New OleDbDataAdapter(strSQL, objConn)
dtAdapter.Fill(dt)
dtAdapter = Nothing
Return dt
End Function
Sub modEditCommand(sender As Object, e As GridViewEditEventArgs)
myGridView.EditIndex = e.NewEditIndex
myGridView.ShowFooter = False
BindData()
End Sub
Sub modCancelCommand(sender As Object, e As GridViewCancelEditEventArgs)
myGridView.EditIndex = -1
myGridView.ShowFooter = True
BindData()
End Sub
Sub modDeleteCommand(sender As Object, e As GridViewDeleteEventArgs)
strSQL = "DELETE FROM customer WHERE CustomerID = '" & myGridView.DataKeys.Item(e.RowIndex).Value & "'"
objCmd = New OleDbCommand(strSQL, objConn)
objCmd.ExecuteNonQuery()
myGridView.EditIndex = -1
BindData()
End Sub
Sub myGridView_RowCommand(source As Object, e As GridViewCommandEventArgs)
If e.CommandName = "Add" Then
'*** CustomerID ***'
Dim txtCustomerID As TextBox = CType(myGridView.FooterRow.FindControl("txtAddCustomerID"), TextBox)
'*** Name ***'
Dim txtName As TextBox = CType(myGridView.FooterRow.FindControl("txtAddName"), TextBox)
'*** Email ***'
Dim txtEmail As TextBox = CType(myGridView.FooterRow.FindControl("txtAddEmail"), TextBox)
'*** CountryCode ***'
Dim ddlCountryCode As DropDownList = CType(myGridView.FooterRow.FindControl("ddlAddCountryCode"), DropDownList)
'*** Budget ***'
Dim txtBudget As TextBox = CType(myGridView.FooterRow.FindControl("txtAddBudget"), TextBox)
'*** Used ***'
Dim txtUsed As TextBox = CType(myGridView.FooterRow.FindControl("txtAddUsed"), TextBox)
strSQL = "INSERT INTO customer (CustomerID,Name,Email,CountryCode,Budget,Used) " & _
" VALUES ('" & txtCustomerID.Text & "','" & txtName.Text & "','" & txtEmail.Text & "' " & _
" ,'" & ddlCountryCode.SelectedItem.Value & "','" & txtBudget.Text & "','" & txtUsed.Text & "') "
objCmd = New OleDbCommand(strSQL, objConn)
objCmd.ExecuteNonQuery()
BindData()
End If
End Sub
Sub myGridView_RowDataBound(source As Object, e As GridViewRowEventArgs)
'*** Footer ***'
IF e.Row.RowType = DataControlRowType.Footer Then
'*** CountryCode ***'
Dim ddlCountryCode As DropDownList = CType(e.Row.FindControl("ddlAddCountryCode"), DropDownList)
IF Not IsNothing(ddlCountryCode) Then
With ddlCountryCode
.DataSource = DataTableCountryCode
.DataTextField = "CountryName"
.DataValueField = "CountryCode"
.DataBind()
End With
End IF
End IF
'*** Edit ***'
IF e.Row.RowType = DataControlRowType.DataRow Then
'*** CustomerID ***'
Dim txtCustomerID As TextBox = CType(e.Row.FindControl("txtEditCustomerID"), TextBox)
IF Not IsNothing(txtCustomerID) Then
txtCustomerID.Text = e.Row.DataItem("CustomerID")
End IF
'*** Name ***'
Dim txtName As TextBox = CType(e.Row.FindControl("txtEditName"), TextBox)
IF Not IsNothing(txtName) Then
txtName.Text = e.Row.DataItem("Name")
End IF
'*** Email ***'
Dim txtEmail As TextBox = CType(e.Row.FindControl("txtEditEmail"), TextBox)
IF Not IsNothing(txtEmail) Then
txtEmail.Text = e.Row.DataItem("Email")
End IF
'*** CountryCode ***'
Dim ddlCountryCode As DropDownList = CType(e.Row.FindControl("ddlEditCountryCode"), DropDownList)
IF Not IsNothing(ddlCountryCode) Then
With ddlCountryCode
.DataSource = DataTableCountryCode
.DataTextField = "CountryName"
.DataValueField = "CountryCode"
.DataBind()
End With
ddlCountryCode.SelectedIndex = ddlCountryCode.Items.IndexOf(ddlCountryCode.Items.FindByValue(e.Row.DataItem("CountryCode")))
End IF
'*** Budget ***'
Dim txtBudget As TextBox = CType(e.Row.FindControl("txtEditBudget"), TextBox)
IF Not IsNothing(txtBudget) Then
txtBudget.Text = e.Row.DataItem("Budget")
End IF
'*** Used ***'
Dim txtUsed As TextBox = CType(e.Row.FindControl("txtEditUsed"), TextBox)
IF Not IsNothing(txtUsed) Then
txtUsed.Text = e.Row.DataItem("Used")
End IF
End IF
End Sub
Sub modUpdateCommand(s As Object, e As GridViewUpdateEventArgs)
'*** CustomerID ***'
Dim txtCustomerID As TextBox = CType(myGridView.Rows(e.RowIndex).FindControl("txtEditCustomerID"), TextBox)
'*** Name ***'
Dim txtName As TextBox = CType(myGridView.Rows(e.RowIndex).FindControl("txtEditName"), TextBox)
'*** Email ***'
Dim txtEmail As TextBox = CType(myGridView.Rows(e.RowIndex).FindControl("txtEditEmail"), TextBox)
'*** CountryCode ***'
Dim ddlCountryCode As DropDownList = CType(myGridView.Rows(e.RowIndex).FindControl("ddlEditCountryCode"), DropDownList)
'*** Budget ***'
Dim txtBudget As TextBox = CType(myGridView.Rows(e.RowIndex).FindControl("txtEditBudget"), TextBox)
'*** Used ***'
Dim txtUsed As TextBox = CType(myGridView.Rows(e.RowIndex).FindControl("txtEditUsed"), TextBox)
strSQL = "UPDATE customer SET CustomerID = '" & txtCustomerID.Text & "' " & _
" ,Name = '" & txtName.Text & "' " & _
" ,Email = '" & txtEmail.Text & "' " & _
" ,CountryCode = '" & ddlCountryCode.SelectedItem.Value & "' " & _
" ,Budget = '" & txtBudget.Text & "' " & _
" ,Used = '" & txtUsed.Text & "' " & _
" WHERE CustomerID = '" & myGridView.DataKeys.Item(e.RowIndex).Value & "'"
objCmd = New OleDbCommand(strSQL, objConn)
objCmd.ExecuteNonQuery()
myGridView.EditIndex = -1
myGridView.ShowFooter = True
BindData()
End Sub
</script>
<html>
<head>
<title>ThaiCreate.Com ASP.NET - GridView</title>
</head>
<body>
<form id="form1" runat="server">
<asp:GridView id="myGridView" runat="server" AutoGenerateColumns="False"
ShowFooter="True"
DataKeyNames="CustomerID"
OnRowEditing="modEditCommand"
OnRowCancelingEdit="modCancelCommand"
OnRowDeleting="modDeleteCommand"
OnRowUpdating="modUpdateCommand"
OnRowCommand="myGridView_RowCommand"
OnRowDataBound="myGridView_RowDataBound">
<Columns>
<asp:TemplateField HeaderText="CustomerID">
<ItemTemplate>
<asp:Label id="lblCustomerID" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CustomerID") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditCustomerID" size="5" runat="server"></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddCustomerID" size="5" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Name">
<ItemTemplate>
<asp:Label id="lblName" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Name") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditName" size="10" runat="server"></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddName" size="10" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Email">
<ItemTemplate>
<asp:Label id="lblEmail" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Email") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditEmail" size="20" runat="server"></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddEmail" size="20" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="CountryCode">
<ItemTemplate>
<asp:Label id="lblCountryCode" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.CountryCode") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:DropDownList id="ddlEditCountryCode" runat="server"></asp:DropDownList>
</EditItemTemplate>
<FooterTemplate>
<asp:DropDownList id="ddlAddCountryCode" runat="server"></asp:DropDownList>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Budget">
<ItemTemplate>
<asp:Label id="lblBudget" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Budget") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditBudget" size="6" runat="server"></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddBudget" size="6" runat="server"></asp:TextBox>
</FooterTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Used">
<ItemTemplate>
<asp:Label id="lblUsed" runat="server" Text='<%# DataBinder.Eval(Container, "DataItem.Used") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox id="txtEditUsed" size="6" runat="server"></asp:TextBox>
</EditItemTemplate>
<FooterTemplate>
<asp:TextBox id="txtAddUsed" size="6" runat="server"></asp:TextBox>
<asp:Button id="btnAdd" runat="server" Text="Add" CommandName="Add"></asp:Button>
</FooterTemplate>
</asp:TemplateField>
<asp:CommandField ShowEditButton="True" CancelText="Cancel" DeleteText="Delete" EditText="Edit" UpdateText="Update" HeaderText="Modify" />
<asp:CommandField ShowDeleteButton="True" HeaderText="Delete" />
</Columns>
</asp:GridView>
</form>
</body>
</html>
Screenshot
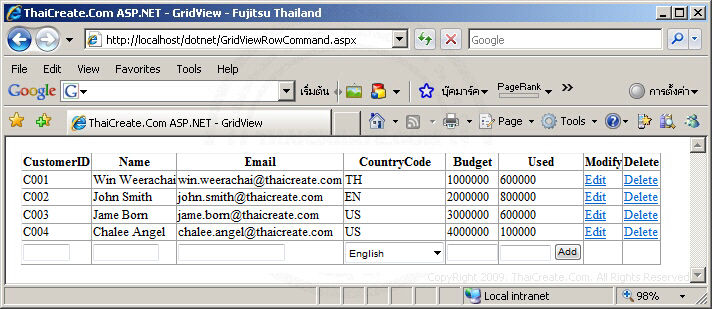
|
ช่วยกันสนับสนุนรักษาเว็บไซต์ความรู้แห่งนี้ไว้ด้วยการสนับสนุน Source Code 2.0 ของทีมงานไทยครีเอท
|
|
|
By : |
ThaiCreate.Com Team (บทความเป็นลิขสิทธิ์ของเว็บไทยครีเอทห้ามนำเผยแพร่ ณ เว็บไซต์อื่น ๆ) |
|
Score Rating : |
  |
|
|
Create/Update Date : |
2010-09-10 17:35:52 /
2017-03-28 21:20:27 |
|
Download : |
|
|
Sponsored Links / Related |
|
|
|
|
|
|